Troubleshooting Common Spring Dependency Injection Issues
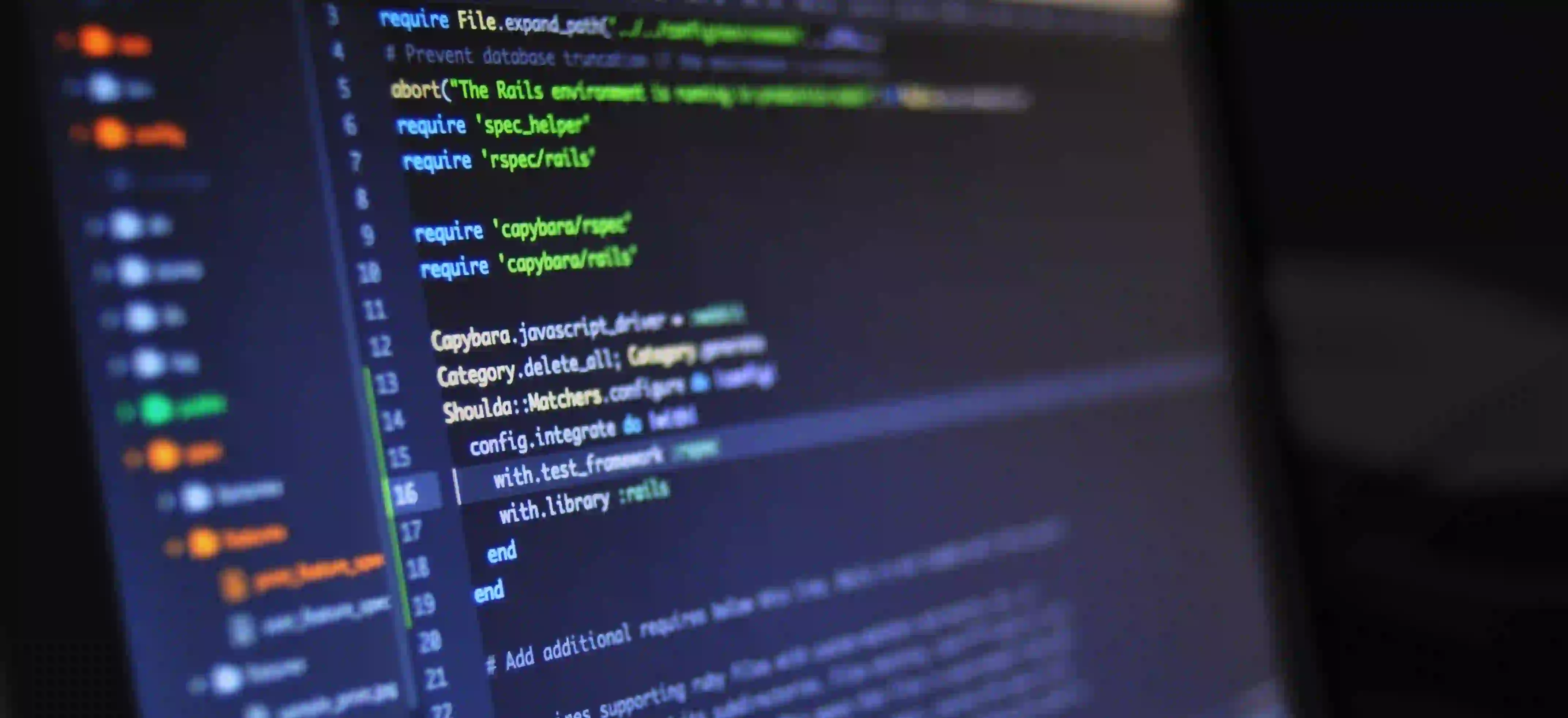
Troubleshooting Common Spring Dependency Injection Issues
When working with the Spring framework, dependency injection is a fundamental concept that enables the construction of loosely coupled and easily testable components. However, like any technology, you may encounter issues related to dependency injection. This article will discuss some common issues that developers face when working with Spring's dependency injection and how to troubleshoot them.
1. NoSuchBeanDefinitionException
The NoSuchBeanDefinitionException
is a common issue that occurs when Spring cannot find a bean definition for a given class. This can happen for several reasons:
Possible Causes:
- The component is not annotated or configured as a Spring bean.
- The component's package is not being scanned by the Spring container.
- The component is defined in a different configuration file.
Troubleshooting Steps:
- Check Component Annotation: Ensure that the class is annotated with
@Component
,@Service
,@Repository
, or@Controller
to indicate that it is a Spring bean. - Scan Configuration: Verify that the package containing the component is being scanned by the Spring container. You can use the
@ComponentScan
annotation or XML configuration to specify the base package(s) to scan. - Check Configuration Files: If using XML configuration, confirm that the component is defined in the appropriate configuration file and that the file is being loaded by the Spring context.
@Component
public class MyComponent {
// Component implementation
}
2. Circular Dependency Exception
In some cases, you may encounter a circular dependency between Spring beans, leading to a BeanCurrentlyInCreationException
. This happens when two or more beans depend on each other directly or indirectly.
Possible Causes:
- Over-reliance on constructor injection when using the same or closely related classes.
- Misconfiguration causing the circular dependency to go unnoticed during application startup.
Troubleshooting Steps:
- Use Setter Injection: Consider using setter injection instead of constructor injection for one of the dependencies. This allows one of the beans to be created without its dependencies immediately.
- Refactor Code: Evaluate if the circular dependency indicates a design issue that may need refactoring. Look for opportunities to break the circular dependency by introducing interfaces or moving common functionality to a separate class.
@Service
public class ServiceA {
private ServiceB serviceB;
@Autowired
public void setServiceB(ServiceB serviceB) {
this.serviceB = serviceB;
}
// ServiceA implementation
}
3. Autowired
Not Working
If you find that the @Autowired
annotation does not inject the expected dependencies, there are a few potential reasons for this issue.
Possible Causes:
- The class where
@Autowired
is used is not managed by the Spring container. - Missing or incorrect configuration of component scanning.
- Incorrect use of
@Autowired
with unsupported types.
Troubleshooting Steps:
- Check Component Registration: Verify that the class using
@Autowired
is itself managed by Spring. Annotate the class with@Component
or one of its stereotype annotations if it represents a Spring bean. - Scan Configuration: Ensure that the package containing the class is scanned for components. Use
@ComponentScan
or XML configuration to specify the package to scan. - Check Variable Types: Confirm that the type being injected matches the type of the bean to be injected. If the dependency is an interface, ensure that there is only one implementation to avoid ambiguity.
@Component
public class MyService {
private MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
// MyService implementation
}
4. No qualifying bean of type
Error
When using @Autowired
or constructor injection, you might encounter a No qualifying bean of type
error, indicating that Spring cannot find a suitable bean to inject.
Possible Causes:
- Multiple beans of the same type are available, causing ambiguity.
- The expected bean is not defined or scanned by the Spring context.
- Errors in the bean configuration or creation process.
Troubleshooting Steps:
- Qualify Bean: If there are multiple beans of the same type, use the
@Qualifier
annotation to specify the bean to be injected. - Check Bean Definition: Ensure that the bean being injected is defined as a Spring bean, either through annotations or XML configuration.
- Inspect Bean Creation: Look for errors or exceptions during the creation of the bean and address any issues that prevent its availability for injection.
@Component
public class MyService {
private MyRepository repository;
@Autowired
public MyService(@Qualifier("myRepositoryImpl") MyRepository repository) {
this.repository = repository;
}
// MyService implementation
}
5. Property Value Not Resolving
When using property placeholder in Spring to inject externalized properties, you may encounter issues where the property values are not resolved as expected.
Possible Causes:
- Incorrect configuration of property placeholder.
- Absence of property files or misplacement of property files.
- Errors in property key naming or property file loading.
Troubleshooting Steps:
- Check Property Placeholder Configuration: Ensure that the property placeholder is configured correctly in the Spring context configuration file, and the placeholder prefix and suffix are defined accurately.
- Verify Property Files: Check for the presence of property files in the expected locations, such as the classpath or custom locations defined in the configuration.
- Inspect Property Key Naming: Confirm that the property keys used in the application code match those defined in the property files, including case sensitivity.
@Configuration
@PropertySource("classpath:myapp.properties")
public class AppConfig {
@Value("${app.username}")
private String username;
// AppConfig configuration
}
To Wrap Things Up
In this article, we have explored several common issues related to Spring dependency injection and provided effective troubleshooting steps for each. By understanding these common problems and their solutions, developers can overcome challenges encountered when working with dependency injection in the Spring framework. Remember to utilize the provided troubleshooting steps when facing dependency injection issues in your Spring-based applications.
By implementing these best practices and troubleshooting tips, you can ensure that your Spring dependency injection works seamlessly, allowing for the construction of robust and maintainable applications.
Now that you're equipped to handle common Spring dependency injection issues, you can approach your development tasks with confidence, knowing that you have the knowledge to navigate and resolve these challenges effectively. Happy coding!
Remember, understanding the 'why' behind the code is crucial for troubleshooting and becoming a more proficient developer. If you want to delve deeper into Spring Dependency Injection, be sure to check out the official Spring Framework Documentation for comprehensive insights.