Optimizing Data Processing with MapReduce
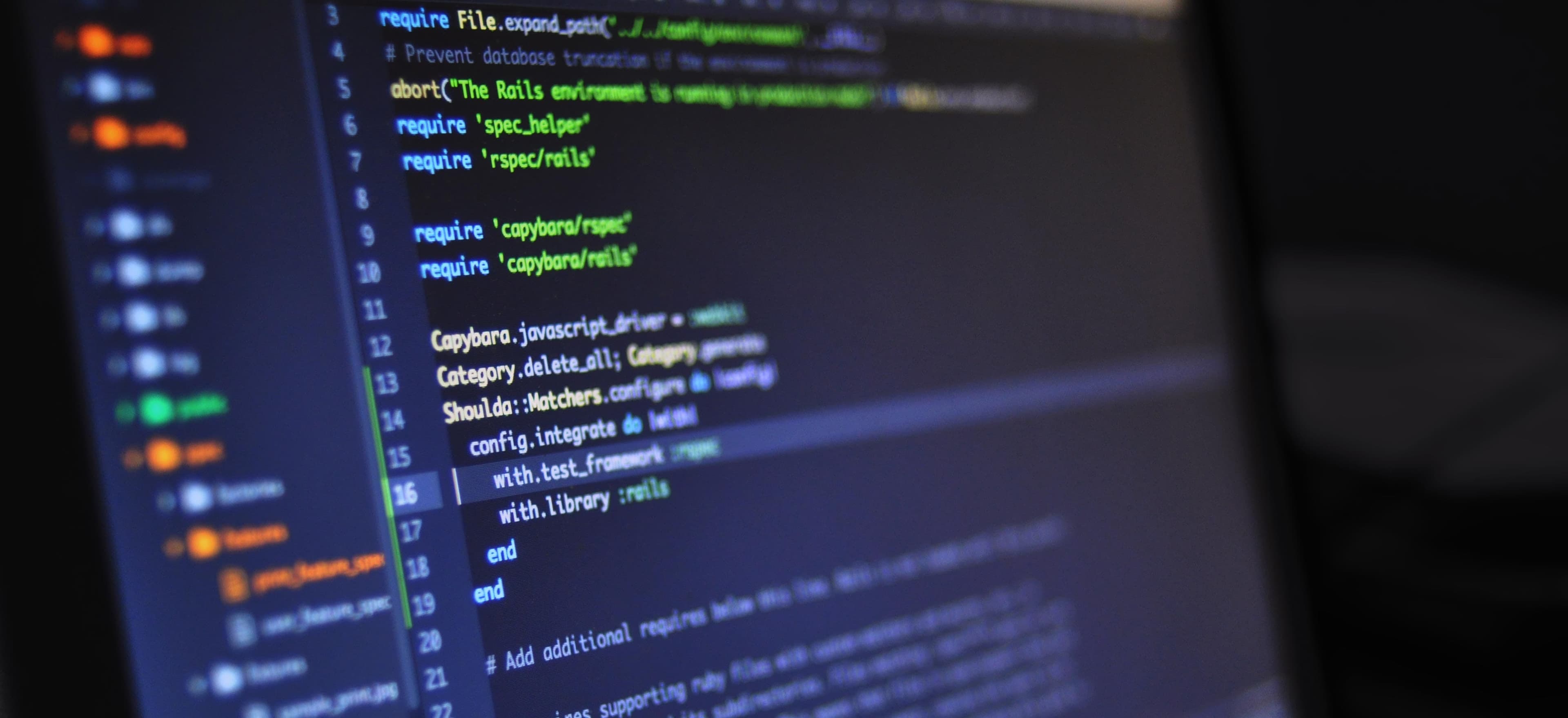
- Published on
Optimizing Data Processing with MapReduce
In the world of big data, efficient data processing is key to unlocking valuable insights and driving business growth. One of the most powerful tools for large-scale data processing is Apache Hadoop, which provides a framework for distributed storage and processing of large datasets. At the core of Hadoop's processing framework is MapReduce, a programming model designed to process vast amounts of data in parallel across a distributed cluster of servers.
In this article, we will explore how to optimize data processing using MapReduce in Java. We will cover key optimization techniques and best practices to improve the performance of MapReduce jobs, allowing you to harness the full potential of Hadoop for your data processing needs.
Understanding MapReduce
MapReduce is a programming model that enables parallel processing of large datasets across a distributed cluster. It consists of two main phases: the Map phase and the Reduce phase.
-
Map phase: In this phase, the input data is divided into independent chunks and processed in parallel by map tasks. Each map task applies a user-defined function (the "map" function) to the input data and generates a set of intermediate key-value pairs.
-
Reduce phase: The intermediate key-value pairs from the Map phase are shuffled, sorted, and then processed by reduce tasks in parallel. The user-defined "reduce" function is applied to the intermediate key-value pairs, producing the final output of the MapReduce job.
Optimizing MapReduce Jobs
Optimizing MapReduce jobs in Java involves a combination of algorithmic improvements, efficient data serialization, and cluster configuration settings. Let's dive into some key optimization techniques that can significantly improve the performance of your MapReduce jobs.
1. Partitioning
Partitioning the intermediate key-value pairs generated by the Map phase is crucial for efficient parallel processing in the Reduce phase. By ensuring that key-value pairs with the same key are routed to the same reduce task, unnecessary data shuffling and network traffic can be minimized.
In Java MapReduce, partitioning is achieved by implementing a custom Partitioner
and overriding the getPartition
method to define the logic for partitioning key-value pairs. This allows you to control how keys are distributed to reduce tasks, optimizing data locality and reducing network overhead.
Example: Custom Partitioner
public class CustomPartitioner extends Partitioner<Text, IntWritable> {
@Override
public int getPartition(Text key, IntWritable value, int numPartitions) {
// Custom logic to assign partition based on key
// Return the partition number
}
}
2. Combiners
Combiners are mini-reduce functions that run on the output of the Map phase before the data is shuffled and sent to the Reduce phase. They summarize the map output locally on each mapper, reducing the amount of data transferred across the network and improving overall efficiency.
By using combiners, you can effectively reduce the volume of intermediate data, lowering the burden on the network and the subsequent reduce tasks. This is particularly beneficial for MapReduce jobs with high data skew and can significantly enhance performance.
Example: Implementing a Combiner
public class CustomCombiner extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) {
// Custom logic to combine intermediate values
// Write combined output to the context
}
}
3. Input and Output Formats
Choosing the appropriate input and output formats is essential for efficient data processing in MapReduce. By utilizing custom input and output formats tailored to your data requirements, you can optimize the serialization and deserialization of data, reducing processing overhead and improving job performance.
In Java MapReduce, you can create custom input and output formats by extending FileInputFormat
and FileOutputFormat
classes, allowing you to define how input data is read and how output data is written. This level of customization enables seamless integration of complex data types and can lead to significant performance gains.
Example: Custom Input and Output Formats
public class CustomInputFormat extends FileInputFormat<LongWritable, Text> {
public RecordReader<LongWritable, Text> createRecordReader(
InputSplit split, TaskAttemptContext context) {
// Custom logic to read input data and create key-value pairs
}
}
4. Speculative Execution
Speculative execution is a feature in Hadoop that aims to mitigate the impact of slow-running tasks by launching duplicate tasks on different nodes. If a task takes longer than expected to complete, speculative execution allows another instance of the task to run in parallel. Once one instance completes, the others are automatically terminated.
Enabling speculative execution for MapReduce jobs can help prevent job slowdown due to straggler tasks, which can prolong job completion times in large-scale clusters. However, it's important to use speculative execution judiciously, as launching excessive speculative tasks can introduce unnecessary load on the cluster.
5. Data Compression
Data compression plays a crucial role in optimizing the storage and transfer of data in MapReduce jobs. By compressing intermediate data outputs and input splits, you can reduce disk I/O, lower storage requirements, and minimize network traffic, resulting in improved overall performance.
In Java MapReduce, you can configure data compression for Map output, Reduce input, and Hadoop's intermediate data storage using mapreduce.map.output.compress
, mapreduce.output.fileoutputformat.compress
, and mapreduce.output.fileoutputformat.compress.type
properties, respectively.
6. Task JVM Reuse
Task JVM reuse is an optimization technique that involves reusing JVM instances for running multiple tasks within a single job. By reusing JVMs, you can eliminate the overhead of JVM startup and shutdown for each task, leading to considerable performance improvements, especially for jobs with a large number of tasks.
In Hadoop, you can enable task JVM reuse by setting the mapreduce.job.jvm.numtasks
property, which specifies the maximum number of tasks that can be executed in a single JVM instance. It is important to strike a balance between the number of tasks and the memory consumption of the JVM to avoid potential out-of-memory issues.
To Wrap Things Up
Optimizing data processing with MapReduce in Java is a multifaceted endeavor that involves a combination of algorithmic improvements, efficient data serialization, and cluster configuration settings. By implementing key optimization techniques such as partitioning, combiners, custom input and output formats, speculative execution, data compression, and task JVM reuse, you can significantly enhance the performance of your MapReduce jobs and unlock the full potential of Hadoop for large-scale data processing.
With a deep understanding of these optimization techniques and best practices, you can harness the power of MapReduce to efficiently process massive datasets, derive valuable insights, and drive informed decision-making for your organization.
By incorporating these optimization techniques into your MapReduce workflows, you can ensure that your data processing tasks are executed with maximum efficiency, allowing you to extract actionable intelligence from your big data resources.
Additional Resources
- Apache Hadoop: Official website for Apache Hadoop.
- Hadoop MapReduce Tutorial: Official MapReduce tutorial provided by Apache Hadoop.
- Hadoop: The Definitive Guide: A comprehensive guide to Hadoop and its related technologies.
In conclusion, optimizing data processing with MapReduce in Java is essential for efficient big data handling. By implementing the discussed optimization techniques and best practices, you can significantly improve the performance of MapReduce jobs, ensuring that your data processing tasks are executed with maximum efficiency.