Troubleshooting Common Tasks in GWT
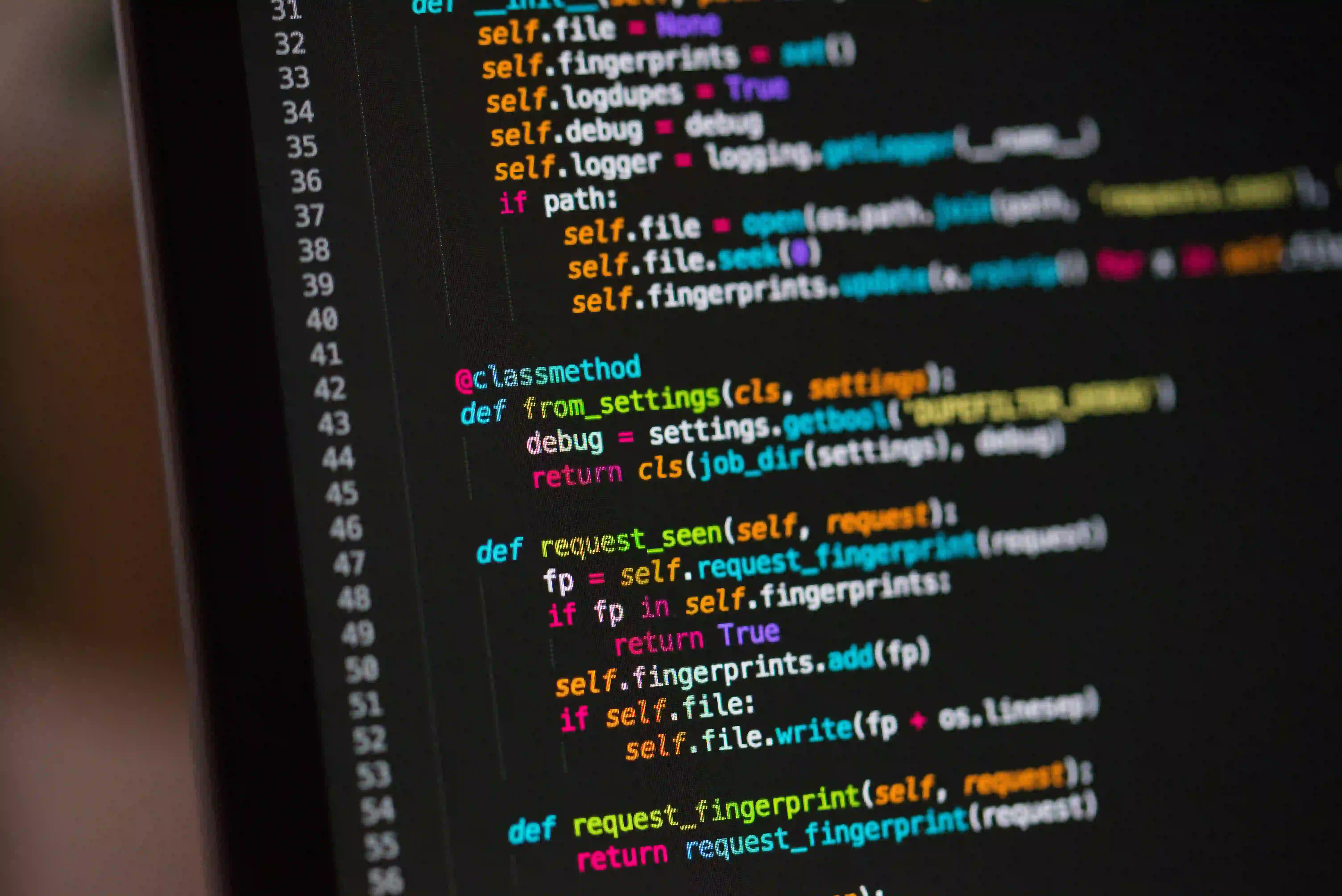
Troubleshooting Common Tasks in GWT
In this blog post, we will cover some common tasks in Google Web Toolkit (GWT) and provide troubleshooting tips to help you resolve any issues you may encounter along the way. GWT is a powerful framework for building modern web applications using Java.
1. Dealing with Cross-Browser Compatibility
When developing web applications with GWT, it's essential to ensure cross-browser compatibility. One common issue developers face is the CSS and layout inconsistencies across different browsers.
To tackle this issue, you can use GWT's CssResource
and ClientBundle
features. By using these features, you can create browser-specific CSS styles and manage them efficiently. This ensures that your application's styles are consistent across various browsers.
Here's an example of using CssResource
and ClientBundle
:
public interface MyResources extends ClientBundle {
@Source("myStyles.css")
MyCssResource style();
}
public interface MyCssResource extends CssResource {
String errorText();
}
In this example, MyCssResource
defines a style called errorText
, which can be used to apply a consistent style for error messages across different browsers.
Additionally, using tools like GWT's CssResource
ensures that only the necessary styles are included in the final compiled CSS, which can help in reducing the overall file size and improving performance.
2. Optimizing Performance
Performance optimization is crucial for web applications, and GWT provides various tools and techniques to help improve the performance of your GWT applications.
One common performance optimization technique is code splitting. GWT allows you to split your application's code into multiple fragments, which can be loaded on-demand. This can significantly reduce the initial load time of your application, especially for larger codebases.
Here's an example of code splitting in GWT:
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.core.client.GWT;
import com.google.gwt.user.client.ui.RootPanel;
public class MyEntryPoint implements EntryPoint {
public void onModuleLoad() {
// Splitting the code into multiple fragments
GWT.runAsync(new RunAsyncCallback() {
public void onSuccess() {
// Code to be executed
}
public void onFailure(Throwable reason) {
// Handle failure
}
});
}
}
In this example, GWT.runAsync
is used to split the code into fragments, and the onSuccess
method contains the code that needs to be executed when the fragment is successfully loaded.
Another performance optimization technique is the use of GWT's built-in compiler optimizations, such as dead code elimination and method inlining. These optimizations can help reduce the size of the compiled JavaScript code and improve runtime performance.
3. Debugging GWT Applications
Debugging GWT applications can sometimes be challenging, especially when dealing with client-side code that is compiled from Java to JavaScript.
To effectively debug GWT applications, you can use SuperDevMode, which allows you to make changes to your Java code and see the results instantly without recompiling the entire application.
To enable SuperDevMode, you can use the following command when starting the development mode server:
mvn gwt:run-codeserver
With SuperDevMode enabled, you can use your browser's developer tools to set breakpoints, inspect variables, and step through the Java code as if it were running in the browser.
Additionally, using GWT's logging framework can be helpful for debugging. By adding log statements to your code, you can easily track the flow of your application and identify any potential issues.
Lessons Learned
In conclusion, GWT is a robust framework for building modern web applications, and by following the troubleshooting tips provided in this post, you can effectively deal with common tasks such as cross-browser compatibility, performance optimization, and debugging.
By leveraging GWT's features and tools, you can ensure that your GWT applications are robust, performant, and maintainable.
For further information on GWT, you can refer to the official GWT documentation.
We hope you found this post helpful in troubleshooting common tasks in GWT. Happy coding!