Asserting Hibernate SQL Statement Count: Best Practices
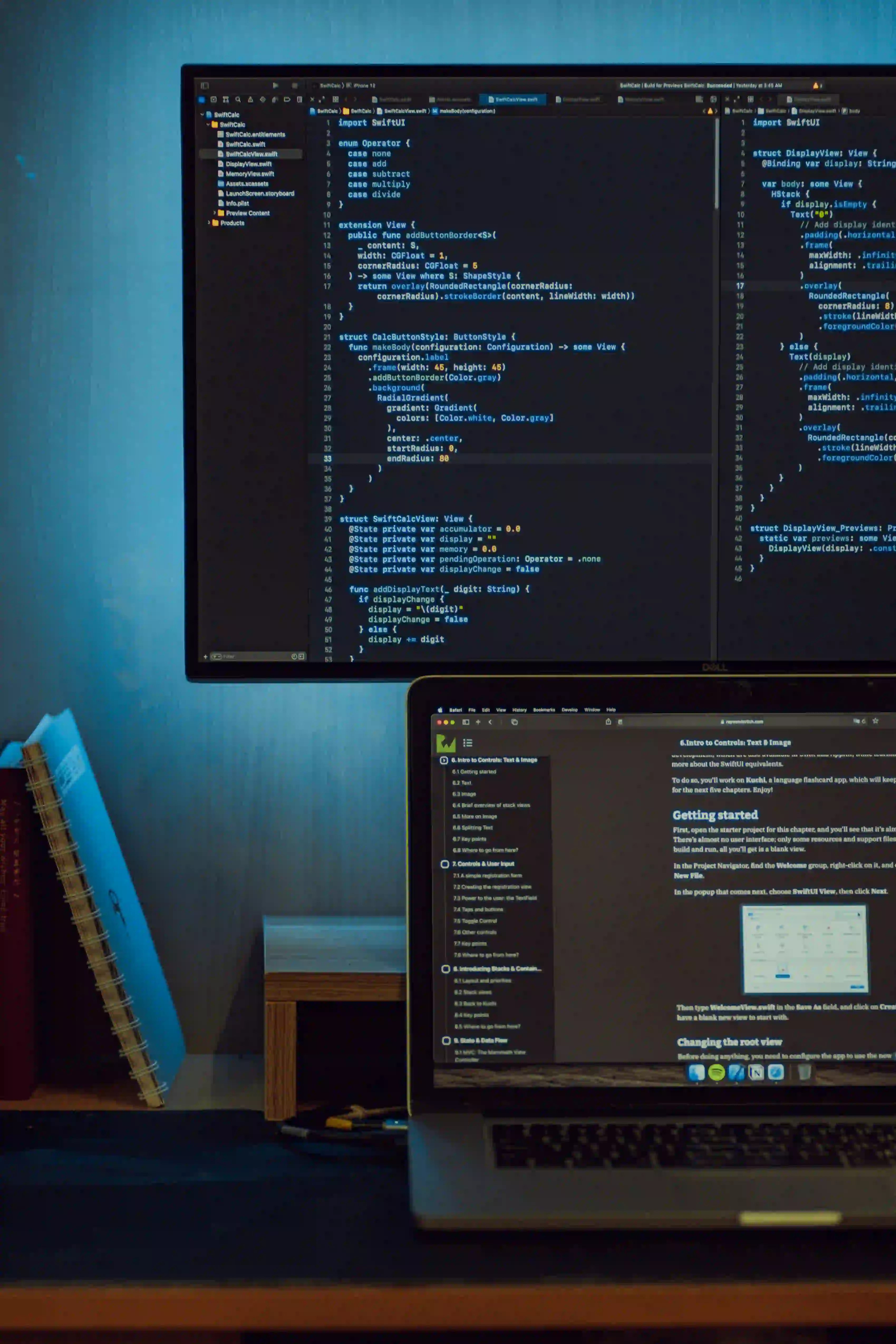
Asserting Hibernate SQL Statement Count: Best Practices
When working with Hibernate, it's essential to have visibility into the SQL statements being executed. In a complex application, the number of SQL queries can significantly impact performance. In this article, we'll explore best practices for asserting Hibernate SQL statement count to optimize application performance.
Why Asserting SQL Statement Count is Important
Hibernate, as an Object-Relational Mapping (ORM) framework, abstracts the underlying database interactions. However, this abstraction can lead to the generation of numerous SQL statements, often resulting in the N+1 query problem. By asserting the SQL statement count, developers can identify and address inefficient database access patterns.
Enabling Hibernate SQL Logging
One approach to assert SQL statement count in Hibernate is by enabling SQL logging. This can be achieved by configuring Hibernate to log SQL statements, including their parameters. The following configuration in application.properties
enables SQL logging in a Spring Boot application:
spring.jpa.properties.hibernate.show_sql=true
spring.jpa.properties.hibernate.format_sql=true
spring.jpa.properties.hibernate.type=trace
The spring.jpa.properties.hibernate.show_sql=true
property enables logging of SQL statements, while spring.jpa.properties.hibernate.format_sql=true
ensures that the logged SQL is formatted for readability. Additionally, setting spring.jpa.properties.hibernate.type=trace
provides more detailed information in the logs.
Why Enable SQL Logging?
Enabling SQL logging provides developers with a detailed overview of the SQL statements executed by Hibernate. This visibility is crucial for identifying inefficient queries, detecting N+1 query problems, and optimizing database access patterns.
Using Hibernate Statistics
Hibernate provides a powerful Statistics API that exposes various metrics, including the number of executed SQL statements. By leveraging Hibernate Statistics, developers can programmatically assert SQL statement count and gain insights into entity load and cache usage.
To enable Hibernate Statistics, the following code can be added to the Hibernate configuration:
entityManager.unwrap(Session.class).getSessionFactory().getStatistics().setStatisticsEnabled(true);
The getStatistics()
method returns an instance of Statistics
that provides access to statistics related to Hibernate usage.
Why Use Hibernate Statistics?
Utilizing Hibernate Statistics allows for programmatic access to crucial metrics, enabling detailed analysis of Hibernate's behavior. This approach is beneficial when asserting SQL statement count programmatically or integrating statistical metrics into monitoring and profiling tools.
Verifying SQL Statement Count in Unit Tests
Unit testing is an integral part of asserting SQL statement count. When writing unit tests for Hibernate-based components, it's essential to verify the number of executed SQL statements. This can be achieved using libraries like AssertJ and Mockito to assert the SQL statement count during test execution.
Consider the following example of unit test verification using Mockito:
@ExtendWith(MockitoExtension.class)
class UserRepositoryTest {
@Mock
private EntityManager entityManager;
@Test
void getUserById_withAssertions() {
// Create and execute the tested method that uses the entityManager
Mockito.verify(entityManager, times(1)).createNativeQuery(anyString()).getResultList();
}
}
In this example, Mockito.verify
is used to assert that a specific number of SQL statements were executed during the test. This approach ensures that the tested methods interact with the database efficiently.
Why Verify SQL Statement Count in Unit Tests?
Verifying SQL statement count in unit tests guarantees that the application components interact with the database optimally. It also serves as a proactive measure to prevent inefficient database access patterns from creeping into the codebase.
Profiling SQL Statements with JProfiler
JProfiler is a powerful Java profiler that can be used to profile SQL statements executed by Hibernate. By analyzing SQL statement count and execution times, developers can identify performance bottlenecks and optimize database interactions.
When profiling Hibernate with JProfiler, focus on the JDBC/Hibernate section to gain insights into SQL statement execution. Identify high-frequency and time-consuming queries to target optimization efforts effectively.
Why Profile SQL Statements with JProfiler?
Profiling SQL statements with JProfiler provides detailed insights into the performance characteristics of database interactions. This approach is invaluable for identifying hotspots, understanding query execution times, and fine-tuning database access for optimal performance.
My Closing Thoughts on the Matter
Asserting Hibernate SQL statement count is crucial for optimizing application performance and ensuring efficient database interactions. By enabling SQL logging, leveraging Hibernate Statistics, verifying SQL statement count in unit tests, and profiling SQL statements with tools like JProfiler, developers can proactively address performance bottlenecks related to database access.
In summary, maintaining visibility and control over SQL statement count in Hibernate is essential for building high-performance, scalable Java applications.
By adopting the best practices outlined in this article, developers can effectively manage SQL statement count and drive optimizations to enhance their Hibernate-based applications.
Remember, asserting SQL statement count is not just about monitoring; it's about actively improving the efficiency and performance of your Hibernate-powered Java applications.