Optimizing Multimedia Integration in Android UI
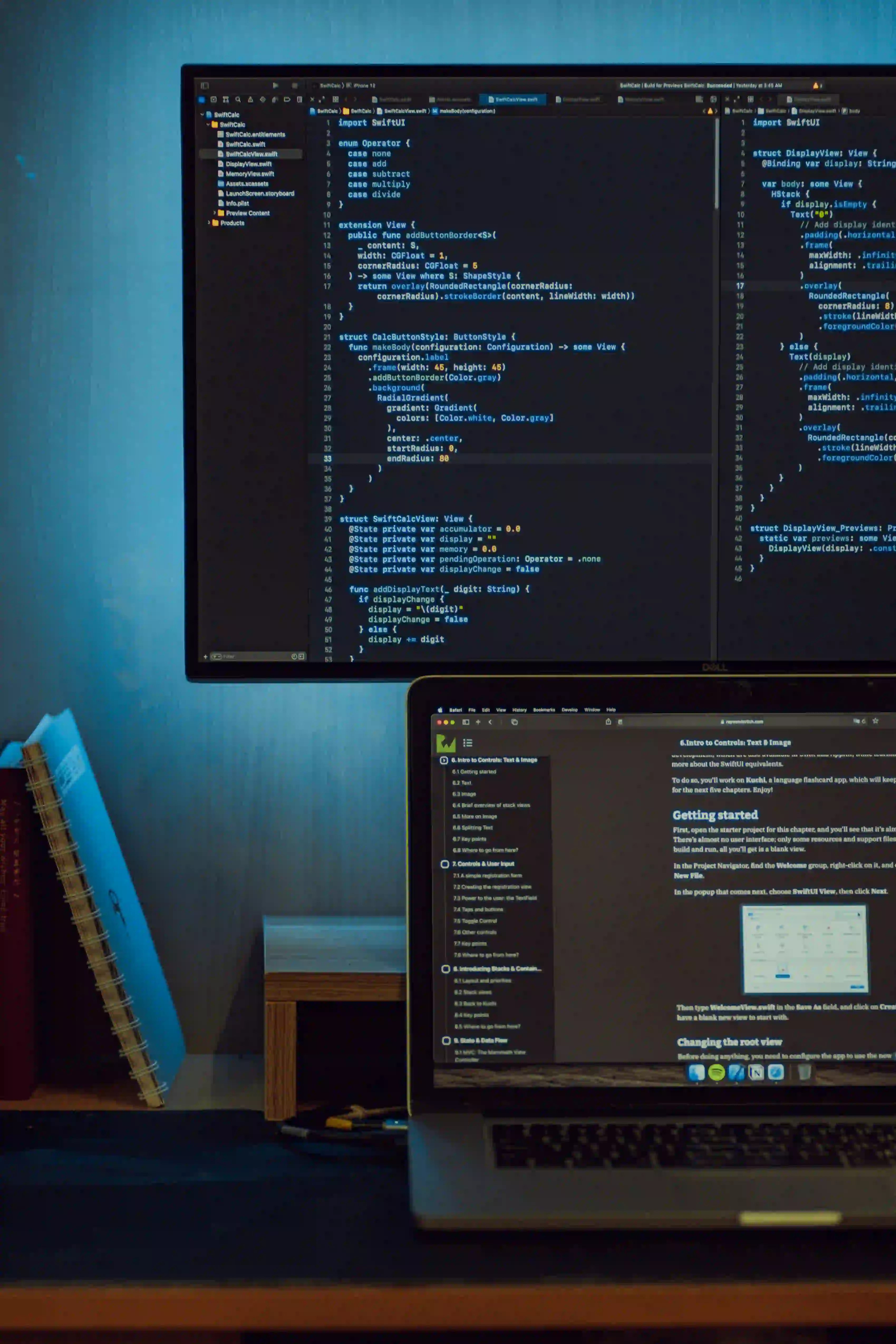
Enhancing User Experience with Multimedia Integration in Android UI
In today's multimedia-driven world, integrating audio and video elements into your Android app's user interface can significantly enhance user engagement and overall experience. Whether you are developing a music streaming app, a video-sharing platform, or a multimedia-rich social media application, effectively incorporating multimedia elements is crucial. In this article, we will explore various strategies for optimizing multimedia integration in Android UI to create a seamless and immersive user experience.
Leveraging the Media Framework in Android
Android provides a robust media framework that enables developers to integrate audio and video playback seamlessly within their applications. This framework includes classes such as MediaPlayer
and MediaRecorder
for audio and video playback and recording, as well as SurfaceView
and TextureView
for rendering video content.
Example: Using MediaPlayer for Audio Playback
MediaPlayer mediaPlayer = MediaPlayer.create(context, R.raw.audio_file);
mediaPlayer.start();
In the above code snippet, we utilize the MediaPlayer
class to create and start audio playback from a raw resource file. This straightforward approach allows for quick integration of audio playback functionality into the app's UI.
Implementing Responsive User Interfaces
When integrating multimedia elements, it is essential to ensure that the user interface remains responsive and maintains a smooth user experience. Long-running multimedia operations, such as loading and buffering large audio or video files, should be handled asynchronously to prevent blocking the main UI thread.
Example: Asynchronous Video Loading with AsyncTask
private class VideoLoader extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
// Load video in background
return null;
}
@Override
protected void onPostExecute(Void aVoid) {
// Update UI with loaded video
}
}
// Trigger video loading
new VideoLoader().execute();
In this example, we use an AsyncTask
to load a video in the background, preventing UI blocking while ensuring a responsive user interface.
Enhancing User Interaction with Gestures
Incorporating touch gestures for multimedia controls can greatly improve user interaction and navigation within the app. Gesture-based controls for functionalities such as seeking through a video timeline, adjusting volume, or switching between audio tracks can elevate the overall user experience.
Example: Implementing Gesture Controls for Video Playback
videoView.setOnTouchListener(new View.OnTouchListener() {
private GestureDetector gestureDetector = new GestureDetector(getContext(),
new GestureDetector.SimpleOnGestureListener() {
@Override
public boolean onSingleTapConfirmed(MotionEvent e) {
// Toggle video playback
return true;
}
@Override
public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) {
// Implement video seeking based on scroll
return true;
}
});
@Override
public boolean onTouch(View v, MotionEvent event) {
gestureDetector.onTouchEvent(event);
return true;
}
});
In this code snippet, we attach an OnTouchListener
to a VideoView
to detect various touch gestures, enabling functionalities such as toggling video playback on single tap and implementing video seeking based on scroll gestures.
Ensuring Compatibility and Performance
While implementing multimedia integration, it is crucial to consider device compatibility and performance optimizations. Utilizing hardware acceleration for video playback, audio focus management, and leveraging the latest media APIs can significantly enhance performance and ensure consistent behavior across various Android devices.
Example: Enabling Hardware Acceleration for Video Playback
<VideoView
android:id="@+id/videoView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hardwareAccelerated="true" />
By setting the hardwareAccelerated
attribute to true
, we enable hardware acceleration for video playback in the VideoView
, leveraging the device's hardware capabilities for improved performance.
Implementing Seamless Transitions and Visual Feedback
Smooth transitions between different multimedia elements and providing visual feedback during playback operations can significantly enhance the user experience. Utilizing transitions and animations when switching between audio tracks, displaying buffering indicators for video loading, and incorporating playback state indicators can create a polished and immersive multimedia experience.
Example: Adding Transition Animation for Audio Track Change
ObjectAnimator alphaAnimator = ObjectAnimator.ofFloat(audioPlayerView, "alpha", 1f, 0f);
alphaAnimator.setDuration(300);
alphaAnimator.addListener(new AnimatorListenerAdapter() {
@Override
public void onAnimationEnd(Animator animation) {
// Switch audio track and update UI
ObjectAnimator fadeInAnimator = ObjectAnimator.ofFloat(audioPlayerView, "alpha", 0f, 1f);
fadeInAnimator.setDuration(300);
fadeInAnimator.start();
}
});
alphaAnimator.start();
In this example, we use ObjectAnimator
to create a smooth alpha transition when switching between audio tracks, providing a visually appealing transition effect for the user.
The Last Word
Incorporating multimedia elements into your Android app's user interface can greatly enrich the user experience, provided it is implemented thoughtfully and optimized for performance. By leveraging the Android media framework, ensuring responsiveness, incorporating gesture controls, optimizing compatibility and performance, and implementing seamless transitions, developers can create truly immersive and engaging multimedia experiences for their users.
In conclusion, effective multimedia integration not only enhances the functionality of the app but also contributes to user satisfaction and retention. By following these best practices and continuously seeking opportunities for improvement, developers can elevate their app's multimedia integration to deliver a superior user experience.
Remember, a well-integrated and responsive multimedia UI can be the differentiator that sets your app apart in today's competitive landscape.
References: