Improving Testing Efficiency with Java Logging
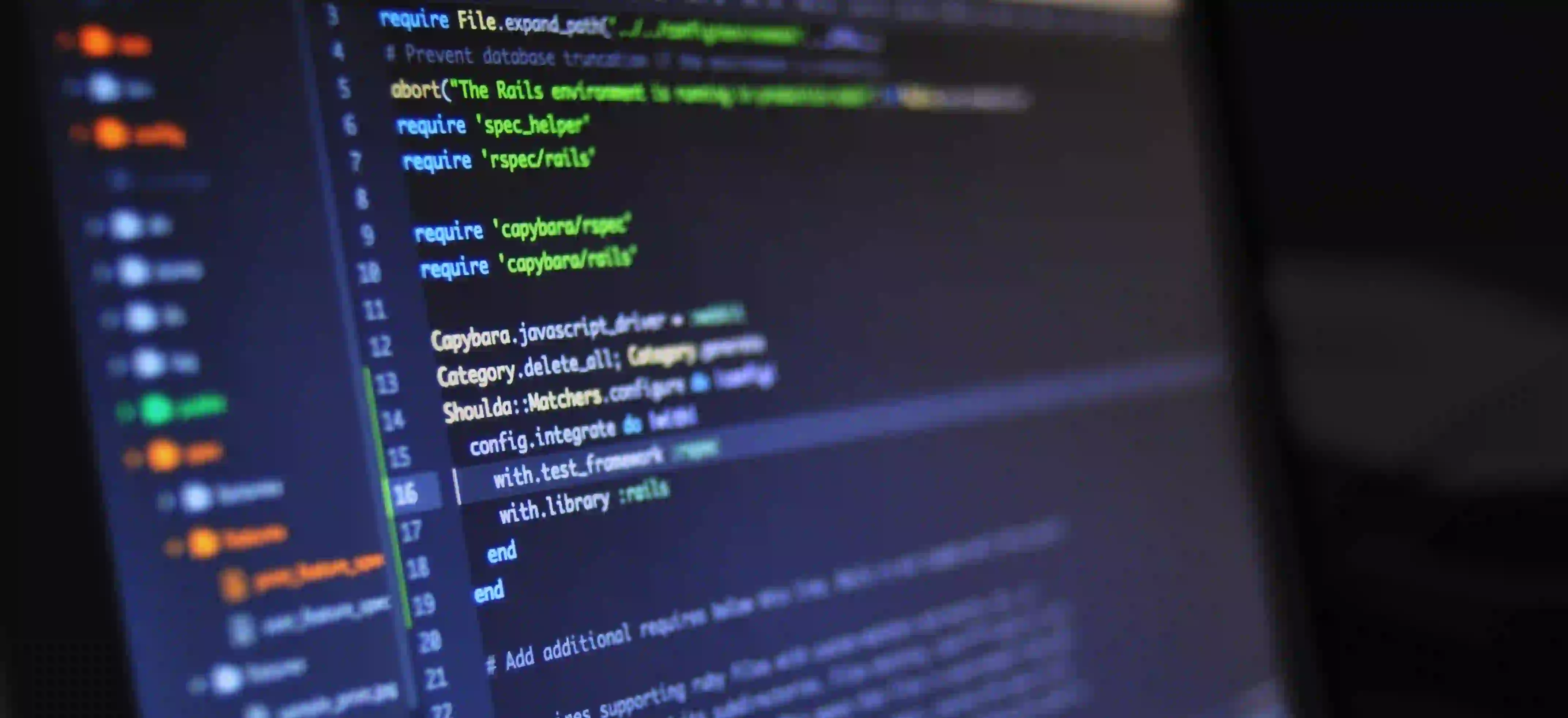
Improving Testing Efficiency with Java Logging
In the world of software development, testing is an essential process to ensure the quality and reliability of our applications. One key aspect of testing is the ability to efficiently track and monitor the behavior of our code. This is where logging comes into play. In Java, logging plays a crucial role in enabling developers to capture and analyze important information during the testing phase.
In this blog post, we will explore how Java logging can be leveraged to improve testing efficiency. We will delve into the various aspects of logging, including its importance, best practices, and how it can be effectively utilized to streamline the testing process.
Importance of Logging in Testing
Logging is the process of recording information about the runtime behavior of an application. It allows developers to track the flow of the program, capture relevant data, and identify potential issues. In the context of testing, logging serves as a powerful tool for understanding how the application behaves under different scenarios.
By incorporating logging into the testing workflow, developers can gain insights into the execution of their code, identify bugs and performance issues, and validate expected outcomes. Additionally, logs provide valuable documentation that can be used for debugging and troubleshooting during the testing phase.
Best Practices for Logging in Java
Before diving into the specifics of logging in Java, it's important to establish some best practices that can guide us in effectively leveraging logging for testing purposes.
Use Appropriate Logging Levels
Java offers different logging levels such as INFO
, DEBUG
, WARN
, and ERROR
to categorize the severity of logged messages. It's crucial to use the appropriate logging level for different types of information. For example, informational messages can be logged at the INFO
level, while error conditions should be logged at the ERROR
level.
Employ Descriptive and Consistent Logging Messages
When logging information, it's important to use descriptive and consistent messages that provide context and clarity. This facilitates easier interpretation and analysis of the logs during testing. Additionally, maintaining a consistent logging format across the codebase can simplify the aggregation and interpretation of logs.
Leverage Parameterized Logging
Parameterized logging allows developers to log messages with placeholders for parameters, which are then replaced by actual values at runtime. This approach eliminates the need for string concatenation and improves performance by deferring string construction when the log level is disabled.
Utilize Logging Frameworks
Java provides several logging frameworks such as Log4j, Logback, and java.util.logging. These frameworks offer powerful features for configuring and managing logs, including the ability to define log appenders, loggers, and log formats. Choosing a suitable logging framework based on the project requirements is essential for efficient logging in Java.
Implementing Logging for Testing Efficiencies
Now that we understand the significance and best practices of logging in Java, let's explore how logging can be implemented to improve testing efficiency.
Logging Test Execution Flow
When conducting tests, logging can be used to track the execution flow of the test cases. By strategically placing log statements within the test methods, developers can monitor the sequence of operations, the input data, and the expected outcomes. This provides a detailed overview of the test execution, facilitating the identification of any deviations from the expected behavior.
Here's an example of how logging can be used to capture the test execution flow:
@Test
public void testCalculation() {
LOGGER.info("Starting testCalculation");
// Perform calculations
LOGGER.debug("Input values: " + inputValue1 + ", " + inputValue2);
int result = calculator.add(inputValue1, inputValue2);
// Validate the result
LOGGER.debug("Expected result: " + expectedValue + ", Actual result: " + result);
assertEquals(expectedValue, result);
LOGGER.info("testCalculation completed successfully");
}
In this example, the LOGGER
is used to record the start of the test, log input values, and compare the expected and actual results. This approach provides comprehensive insights into the test execution, enabling efficient analysis and debugging.
Logging Test Environment Configuration
During testing, it's common to execute tests in different environments such as development, staging, and production. Logging can be employed to capture the configuration and specifics of the test environment, including database connections, external service integrations, and environment variables. This information is valuable for understanding the context in which the tests are being performed and allows for quick identification of environment-related issues.
@Before
public void setUp() {
LOGGER.info("Setting up test environment");
// Initialize database connection
LOGGER.debug("Database URL: " + dbUrl);
database.initializeConnection(dbUrl, username, password);
// Set up mock services
LOGGER.debug("Setting up mock services");
serviceMock.setup();
LOGGER.info("Test environment setup completed");
}
In this setup method, logging is used to capture the initialization of the test environment, including database URL, service mocks, and completion of the setup. This logging practice aids in ensuring that the test environment is configured as expected and provides visibility into the environment-specific operations.
Capturing Test Failures and Errors
When tests fail or encounter errors, logging plays a crucial role in capturing relevant information about the failure. By logging detailed error messages, stack traces, and the context in which the failure occurred, developers can quickly diagnose the root cause of the issue. This accelerates the debugging process and enables efficient resolution of test failures.
@Test
public void testInvalidInput() {
LOGGER.info("Starting testInvalidInput");
try {
// Invoke the method with invalid input
LOGGER.debug("Invoking method with invalid input");
calculator.performOperation(null);
fail("Expected IllegalArgumentException was not thrown");
} catch (IllegalArgumentException e) {
LOGGER.info("Expected exception caught: " + e.getMessage());
// Additional error handling if required
}
LOGGER.info("testInvalidInput completed");
}
In this example, logging is utilized to capture the attempt to invoke the method with invalid input, as well as the handling of the expected exception. This level of logging detail provides visibility into the test failure scenario and aids in understanding the conditions leading to the failure.
Key Takeaways
In this blog post, we've explored the significance of logging in testing, established best practices for logging in Java, and delved into the implementation of logging for testing efficiencies. Logging serves as a fundamental tool for tracking and monitoring the behavior of our code during testing, enabling developers to gain valuable insights, identify issues, and streamline the debugging process.
By integrating logging practices such as capturing test execution flow, logging test environment configuration, and handling test failures, developers can significantly improve testing efficiency and accelerate the identification and resolution of issues.
In conclusion, logging is not just a means of capturing information; it's a powerful ally in ensuring the reliability and quality of our software through effective testing practices.
To further enhance your understanding of logging in Java, you can explore Log4j and Logbacklogging frameworks.
Happy logging and testing!