Optimizing API Success through Effective API Modeling
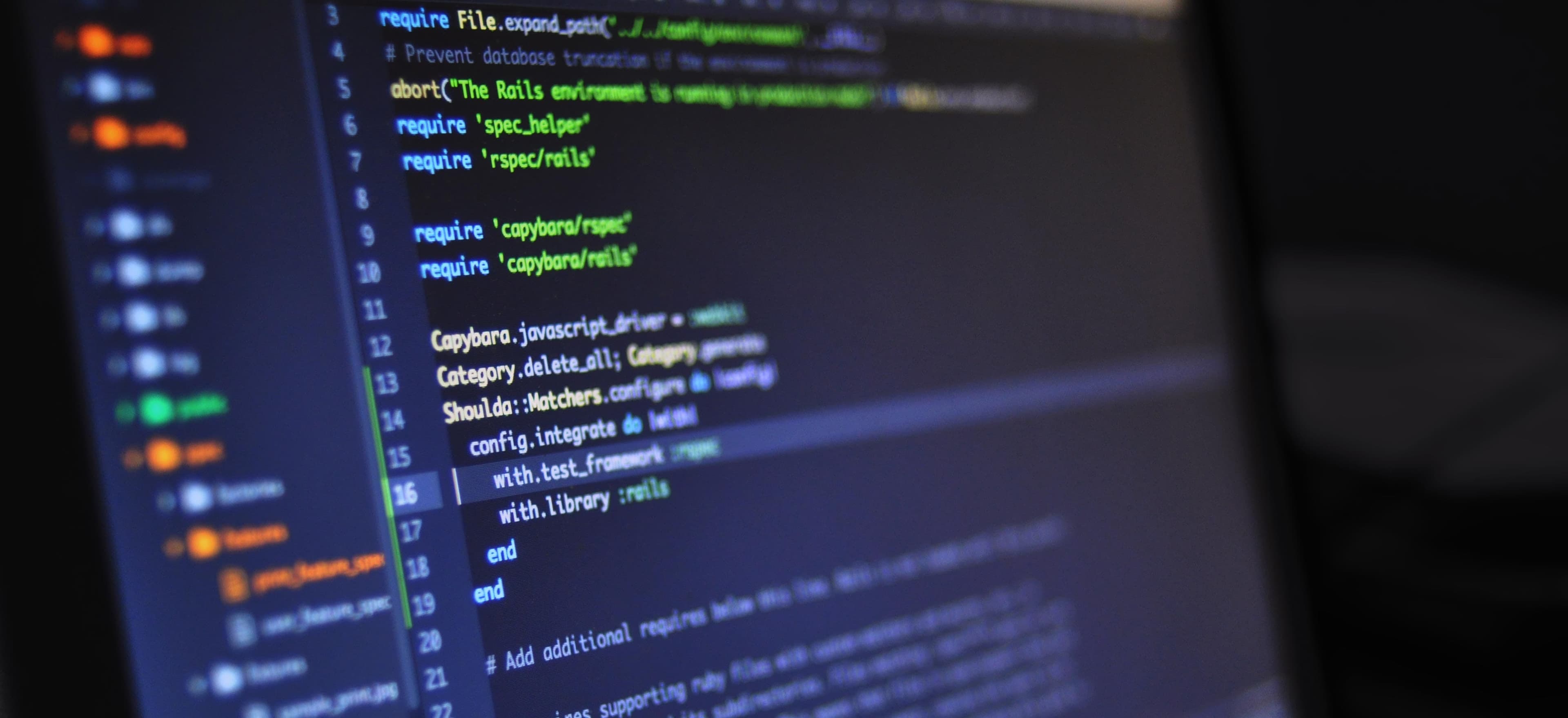
- Published on
Optimizing API Success through Effective API Modeling
In the world of software development, APIs (Application Programming Interfaces) play a crucial role in enabling various software systems to communicate and interact with each other. Creating an effective API requires careful planning and consideration of various aspects such as design, functionality, and usability. This is where API modeling comes into play.
What is API Modeling?
API modeling is the process of creating a blueprint that defines the structure, behavior, and interactions of an API. It involves designing the endpoints, request-response payloads, data models, authentication mechanisms, and error handling. API modeling serves as a fundamental step in API development as it allows developers to visualize and plan the API's functionality before any code is written.
Benefits of Effective API Modeling
1. Improved Communication and Collaboration
API models serve as a common language that can be understood by developers, testers, product managers, and other stakeholders. By creating a clear and concise API model, teams can communicate effectively, ensuring everyone has a shared understanding of the API's features and behavior.
2. Early Issue Identification
By modeling an API before implementation, potential issues and design flaws can be identified early in the development process. This proactive approach helps in avoiding costly rework and redesign as the API progresses towards implementation.
3. Better Developer Experience
Well-modeled APIs provide a better developer experience by offering clear documentation, predictable behavior, and intuitive design. Developers consuming the API can easily understand its capabilities and integrate it into their applications seamlessly.
4. Facilitates Testing
API models can be utilized to create mock endpoints for testing purposes, allowing developers to validate their integration logic even before the actual API implementation is completed. This significantly streamlines the testing phase of API development.
API Modeling Tools
Several tools are available for creating API models. Some of the prominent ones include:
-
Swagger (OpenAPI Specification): Swagger is a widely used framework for designing, building, and documenting APIs. It provides a comprehensive toolset for creating API models in the OpenAPI Specification format.
-
RAML (RESTful API Modeling Language): RAML is a human-readable language for describing APIs. It allows for easy creation of models and documentation, making it simple for both developers and non-developers to understand the API.
-
API Blueprint: API Blueprint is a high-level API description language that uses markdown to write a description of an API's resources and actions.
-
Postman: Postman is not just a popular API development tool but also offers features to create and share API specifications using the Postman Collection format.
The Role of API Modeling in Java Development
When it comes to Java development, API modeling is particularly crucial due to Java's widespread usage in building enterprise-level applications and web services. The clarity and precision provided by API modeling can make a significant impact on the success of Java-based APIs. Let's delve into the key aspects of API modeling in the context of Java development.
1. Designing Clear Data Models
In Java development, defining clear and consistent data models is paramount for building robust APIs. API modeling allows developers to specify data structures using JSON or XML representations, ensuring that the data exchanged between the API and its consumers is well-defined and easily understandable.
public class User {
private String username;
private String email;
// Getters and setters
}
In the above example, the User
class represents a basic data model for a user entity, containing attributes such as username
and email
. Through API modeling, the structure of such data models can be documented and shared across the development team, fostering better alignment.
2. Defining Resource Endpoints
Java-based APIs often follow the REST (Representational State Transfer) architectural style, which revolves around resources and their interactions. API modeling enables the clear definition of resource endpoints, including the HTTP methods they support and the expected request and response payloads.
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
// Retrieve user logic
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
// Create user logic
}
// Other endpoint definitions
}
In the above snippet, the UserController
class in a Spring-based Java application defines endpoints for retrieving and creating user resources. Effective API modeling ensures that such endpoints align with the intended functionality and adhere to industry best practices.
3. Documenting API Contracts
Java developers extensively rely on documentation to understand how to consume and integrate external APIs. API modeling tools allow the generation of interactive documentation that details the API's contract, including endpoint URLs, request parameters, response payloads, and error codes.
Implementing Effective API Modeling in Java with SpringFox
SpringFox, integrated with the Spring framework, provides an elegant solution for API modeling in Java-based applications. It simplifies the generation of API models in the OpenAPI Specification format and offers seamless integration with Swagger UI for interactive documentation.
Setting Up SpringFox
To integrate SpringFox for API modeling, add the following dependencies to your Maven project's pom.xml
:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
For Gradle projects, include the following dependency in the build.gradle
file:
implementation 'io.springfox:springfox-boot-starter:3.0.0'
Generating API Models
Once SpringFox is set up, annotate your API controllers and models using SpringFox annotations to generate API models automatically. For example:
@RestController
@RequestMapping("/users")
@Api(tags = "users")
public class UserController {
@Operation(summary = "Get user by ID", description = "Retrieve user details by ID")
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Successful operation",
content = @Content(schema = @Schema(implementation = User.class))),
@ApiResponse(responseCode = "404", description = "User not found")
})
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
// Retrieve user logic
}
@Operation(summary = "Create user", description = "Create a new user")
@ApiResponses(value = {
@ApiResponse(responseCode = "201", description = "User created",
content = @Content(schema = @Schema(implementation = User.class))),
@ApiResponse(responseCode = "400", description = "Invalid input")
})
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
// Create user logic
}
// Other endpoint definitions
}
In the above example, annotations such as @Api
, @Operation
, @ApiResponse
, and @Schema
are used to specify the API's details, which SpringFox leverages to automatically generate the OpenAPI Specification.
Enabling Swagger UI
SpringFox comes with Swagger UI, which provides an interactive web interface for visualizing and testing the API. To enable Swagger UI, add the following configuration to your project:
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.controllers"))
.paths(PathSelectors.any())
.build();
}
}
With the above configuration, accessing http://localhost:8080/swagger-ui/
in your web browser displays an interactive documentation page containing the API model generated by SpringFox.
Final Considerations
Effective API modeling is a cornerstone of successful API development, and its importance is amplified in the realm of Java. By incorporating API modeling tools such as SpringFox into Java development workflows, teams can streamline the process of creating well-designed APIs, communicate more effectively, and ultimately enhance the overall developer experience. Embracing API modeling as an integral part of Java API development is key to delivering robust and interoperable APIs that align with industry best practices.
API modeling not only fosters clarity in API design but also empowers developers to build and consume APIs with confidence, driving the success of software systems interconnected through APIs.
References: