Efficiently Implement Bootstrap Pagination in Spring Data
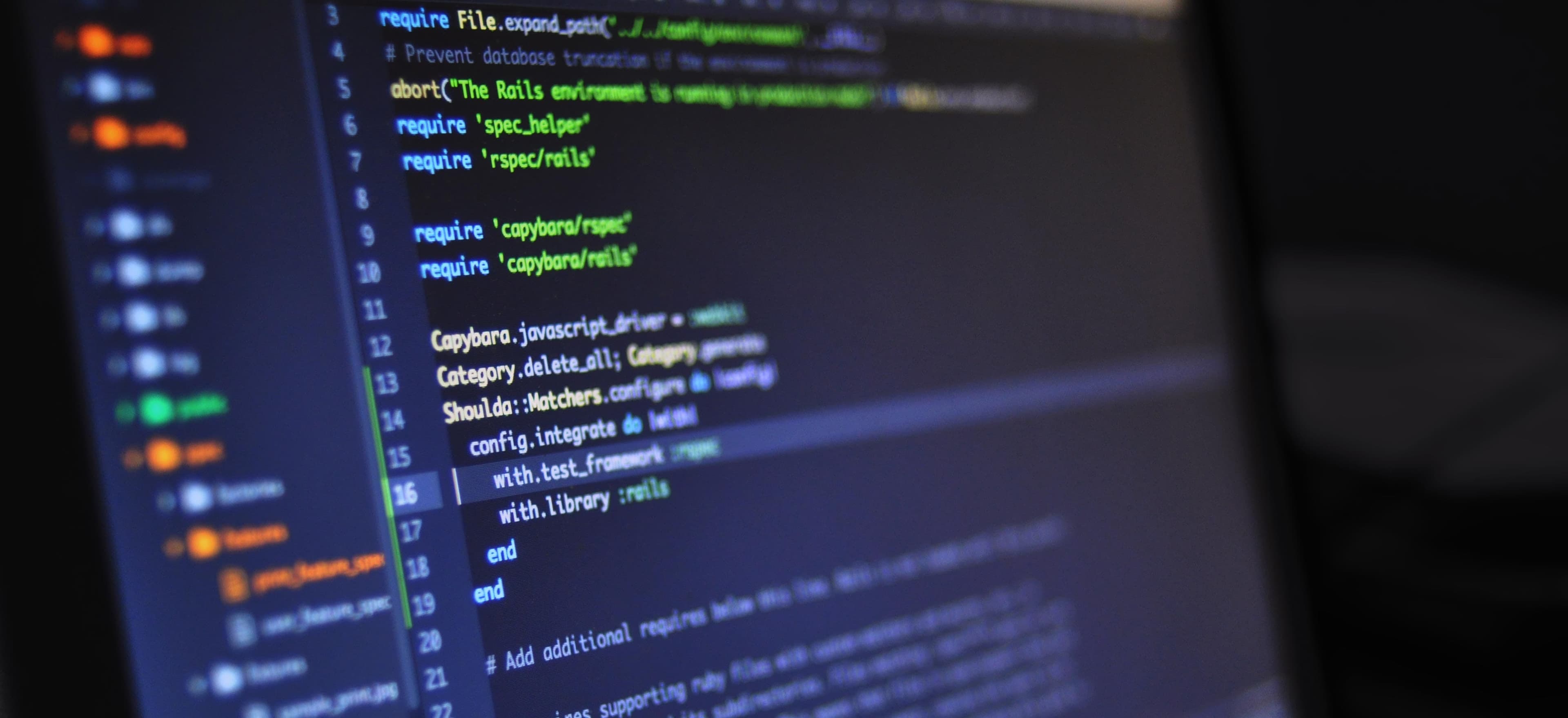
- Published on
Efficiently Implement Bootstrap Pagination in Spring Data
Pagination is a crucial aspect of web development, especially when dealing with large datasets. Implementing pagination in a Spring Data application can significantly enhance the user experience by breaking down a large dataset into smaller, more manageable chunks. In this blog post, we will explore how to efficiently implement Bootstrap pagination in a Spring Data application to achieve a seamless and responsive user interface.
Understanding Bootstrap Pagination
Bootstrap offers a powerful and flexible pagination component that seamlessly integrates with web applications. Bootstrap pagination provides a sleek and responsive way to navigate through multiple pages of content. It allows users to easily jump to specific pages or navigate forward and backward through the dataset.
Integrating Bootstrap Pagination with Spring Data
When working with Spring Data, integrating Bootstrap pagination involves leveraging the capabilities of both Spring Data and Bootstrap to create a seamless pagination experience. Here, we will walk through the process of implementing Bootstrap pagination in a Spring Data application.
Setting Up the Spring Data Repository
public interface UserRepository extends JpaRepository<User, Long> {
Page<User> findAll(Pageable pageable);
}
In the above code snippet, we define a Spring Data repository interface UserRepository
with a method that returns a Page
of users. The Pageable
parameter allows us to specify the pagination configuration, such as the page size and the current page number.
Implementing the Controller
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public Page<User> getUsers(@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
return userRepository.findAll(PageRequest.of(page, size));
}
}
In the UserController
, we inject the UserRepository
and define a method to fetch users with pagination support. The @RequestParam
annotation allows us to dynamically specify the page number and page size when making a request.
Creating the HTML View with Bootstrap Pagination
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Bootstrap CSS -->
<link href="https://stackpath.bootstrapcdn.com/bootstrap/5.1.1/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1>User List</h1>
<table class="table">
<!-- User data display goes here -->
</table>
<nav>
<ul class="pagination">
<li class="page-item">
<a class="page-link" href="#" aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<!-- Pagination links dynamically generated here -->
<li class="page-item">
<a class="page-link" href="#" aria-label="Next">
<span aria-hidden="true">»</span>
</a>
</li>
</ul>
</nav>
</div>
<!-- Bootstrap JS -->
<script src="https://stackpath.bootstrapcdn.com/bootstrap/5.1.1/js/bootstrap.bundle.min.js"></script>
</body>
</html>
In the HTML view, we utilize Bootstrap's pagination component to render the pagination links. The <ul>
element with the class pagination
dynamically generates the pagination links based on the total number of pages.
Making it Interactive with JavaScript
To enhance the user experience, we can make the pagination interactive by leveraging JavaScript to handle page navigation and updates to the user list.
Fetching Users via AJAX
function getUsers(page, size) {
$.ajax({
url: '/users?page=' + page + '&size=' + size,
method: 'GET',
success: function(data) {
// Update the user list in the UI
}
});
}
Using jQuery's Ajax function, we make a GET request to the /users
endpoint with the specified page and size parameters. Upon success, we update the user list in the UI with the fetched data.
Handling Click Events
$(document).on('click', '.page-link', function(e) {
e.preventDefault();
var page = $(this).data('page');
getUsers(page, 10); // Assuming a default page size of 10
});
By attaching a click event listener to the pagination links, we intercept user clicks and dynamically fetch the appropriate user data based on the selected page.
Key Takeaways
In conclusion, implementing Bootstrap pagination in a Spring Data application can significantly improve the user experience by efficiently organizing and presenting large datasets. By leveraging Spring Data's pagination support and integrating Bootstrap's sleek pagination component, we can create a responsive and user-friendly interface. Additionally, incorporating JavaScript to handle interactive pagination further enhances the overall usability of the application.
By following the steps outlined in this blog post, you can efficiently implement Bootstrap pagination in your Spring Data application, providing a seamless and intuitive user experience.
For further insights into Spring Data and Bootstrap pagination, you may find it beneficial to explore the documentation for Spring Data JPA and Bootstrap Pagination.
Happy coding!