How to Handle Broken Code Due to JIT Complications
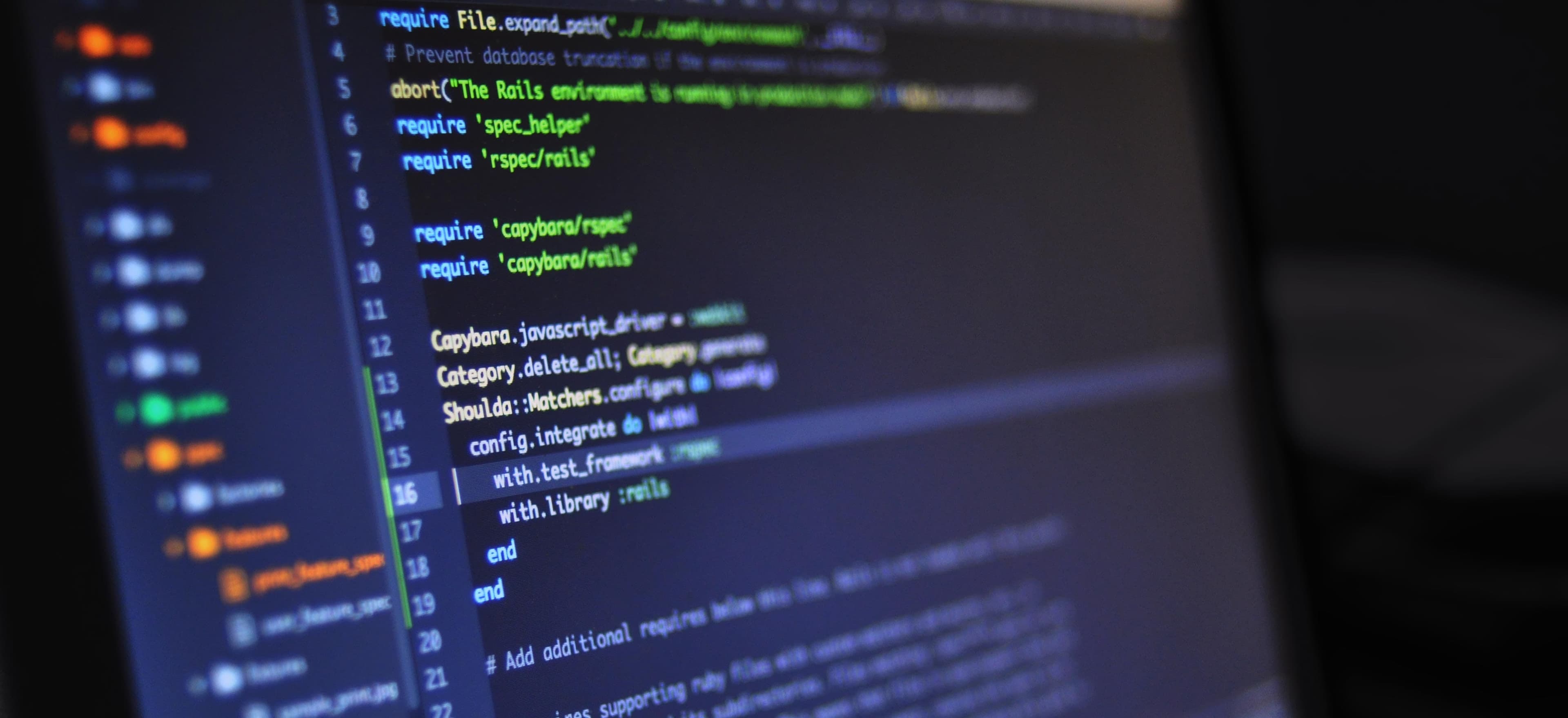
- Published on
Dealing with Broken Code Due to JIT (Just-In-Time) Complications in Java
Have you ever encountered a situation where your Java code worked perfectly on your local machine, but suddenly crashed when deployed on a production server? This frustrating and often perplexing issue may be due to JIT (Just-In-Time) complications. In this post, we'll explore what JIT is, how it can lead to broken code, and most importantly, how to handle and resolve these challenges.
Understanding JIT Compilation in Java
In Java, the JIT compiler is responsible for optimizing bytecode into native machine code at runtime. This optimization is intended to improve performance by identifying and executing hotspots in the code. However, in some cases, the JIT compilation process can introduce unforeseen issues that lead to broken code.
Common Symptoms of JIT-Related Code Breakage
When JIT complications arise, they can manifest in various ways, such as:
- Inconsistent Behavior: Code performing differently on different environments.
- Unexplained Crashes: Sudden termination of the application without clear error messages.
- Performance Degradation: Despite JIT optimizations, the application may exhibit slower performance.
Identifying the Root Cause
When faced with broken code due to JIT complications, the first step is to identify the root cause. Here are some common factors that can contribute to JIT-related issues:
- Code Dependencies: External libraries or dependencies may not be JIT-friendly, leading to conflicts.
- Platform Differences: Differences in hardware, operating systems, or JVM implementations can impact JIT behavior.
- Code Complexity: Highly complex code may confuse the JIT compiler, leading to unexpected outcomes.
Strategies for Handling JIT-Related Code Issues
1. Reproducing the Issue
Before addressing the problem, ensure that the issue is reproducible in a controlled environment. Replicating the problem locally will help in understanding and debugging the JIT-related complications effectively.
2. Analyzing Runtime Logs
Thoroughly analyze the logs from the runtime environment where the issue occurred. Look for any JIT-related warnings, errors, or unusual behaviors that might hint at the root cause.
3. JIT Tuning and Diagnostics
Modern JVM implementations offer various flags and options for tuning and diagnosing JIT behavior. Experiment with these options to understand how JIT is optimizing the code.
4. Removing Code Optimizations
Temporarily disabling JIT optimizations for specific code segments can help in identifying which parts of the code are causing issues. This can be achieved using tools like the -XX:CompileCommand
JVM option.
5. Version Compatibility Checks
Ensure that all dependencies, including external libraries and frameworks, are compatible with the version of the JVM being used. Mismatched versions can lead to unpredictable JIT behavior.
6. Collaboration with JIT Experts
In complex scenarios, collaborating with JIT experts or JVM vendors can provide valuable insights and potential solutions. They can offer deep expertise in analyzing and resolving JIT-related complications.
Example: JIT Complication in Java Code
Consider the following Java code snippet that experiences unexpected behavior due to JIT optimization:
public class JITExample {
public static void main(String[] args) {
int a = 10;
int b = 20;
int c = a + b;
System.out.println("The result is: " + c);
}
}
In this simple example, the JIT compiler might aggressively optimize the code, leading to unexpected results or performance issues, especially when integrated into a more complex codebase.
To address this, consider adding explicit memory barriers or using the -XX:CompileCommand
option to control JIT optimizations for specific methods or classes.
By incorporating these strategies and taking a methodical approach, you can effectively handle and resolve broken code due to JIT complications in Java, ensuring that your applications perform reliably across different environments.
In conclusion, JIT-related code issues in Java can be challenging to diagnose and resolve, but with a systematic approach and the right tools, it's possible to address these complications effectively. Remember to meticulously analyze runtime logs, test in controlled environments, and leverage JVM tuning options to gain deeper insights and mitigate JIT-related challenges. By doing so, you can ensure the stability and consistency of your Java applications, delivering a superior user experience across diverse deployment environments.