Integrating RabbitMQ with Spring Integration: Common Challenges
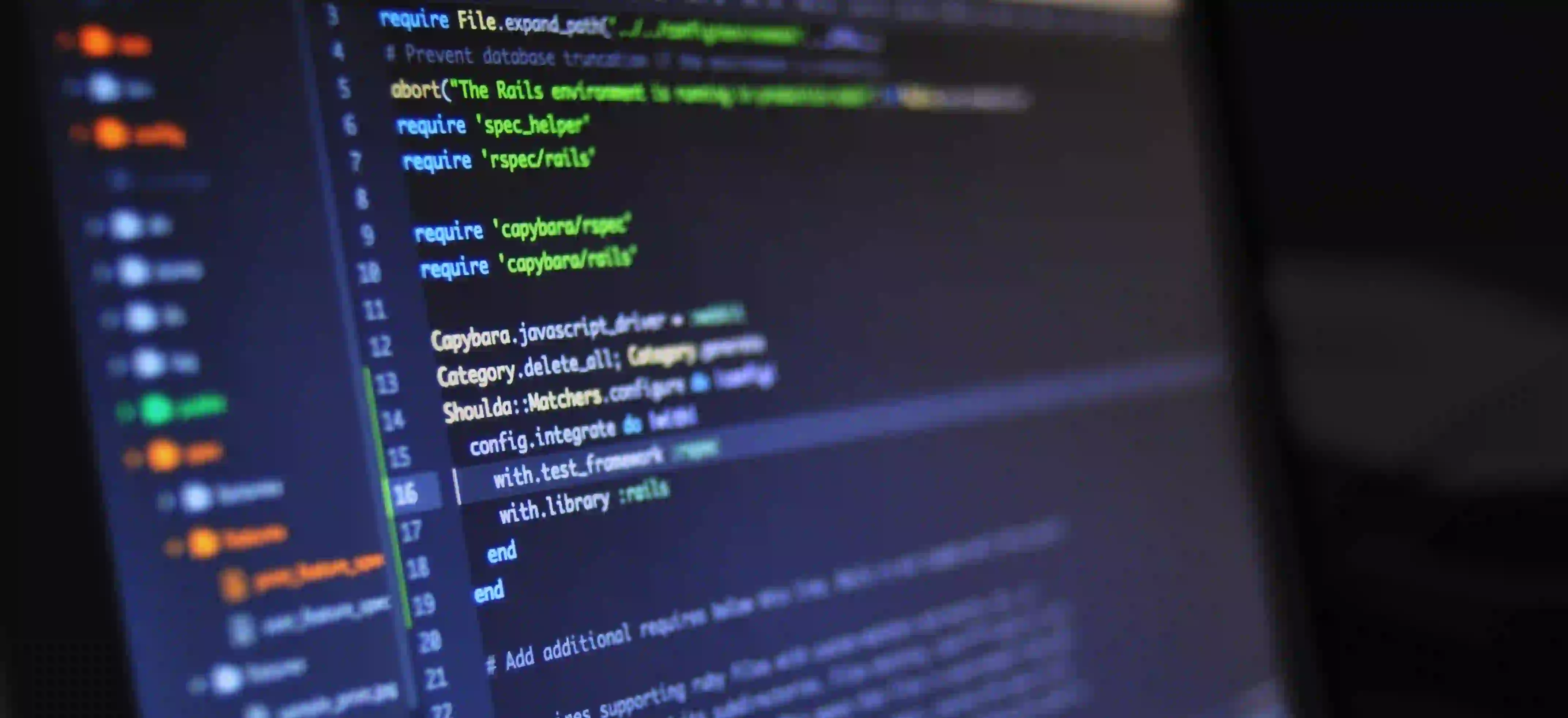
Integrating RabbitMQ with Spring Integration: Common Challenges
When it comes to building a robust and efficient messaging system in Java, RabbitMQ and Spring Integration are two popular choices. RabbitMQ, as a robust message broker, and Spring Integration, as a powerful framework for integrating enterprise systems, work seamlessly together to provide a reliable messaging solution. However, integrating RabbitMQ with Spring Integration comes with its own set of challenges. In this article, we’ll explore some of the common challenges developers may face when working with these technologies and discuss best practices for overcoming them.
Setting Up RabbitMQ with Spring Integration
Before delving into the nitty-gritty details of common challenges, let's first understand the process of setting up RabbitMQ with Spring Integration.
Dependencies
To begin, you need to include the necessary dependencies in your project's pom.xml
file if you're using Maven.
<dependency>
<groupId>org.springframework.amqp</groupId>
<artifactId>spring-rabbit</artifactId>
<version>2.2.7.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-amqp</artifactId>
<version>5.2.9.RELEASE</version>
</dependency>
Configuring RabbitMQ Connection
Next, configure the connection to the RabbitMQ server in your Spring application context.
<rabbit:connection-factory id="connectionFactory" host="localhost" username="guest" password="guest" />
Defining Message Channels
Define the input and output channels for sending and receiving messages using Spring Integration.
<int:channel id="inputChannel" />
<int:channel id="outputChannel" />
Configuring Message Endpoints
Configure the message-driven endpoints that will consume and produce messages from/to RabbitMQ.
<int-amqp:inbound-channel-adapter channel="inputChannel" queue-name="queue" connection-factory="connectionFactory" />
<int-amqp:outbound-channel-adapter channel="outputChannel" exchange-name="exchange" routing-key="routingKey" connection-factory="connectionFactory" />
With the basic setup in place, let’s now dive into the common challenges faced when integrating RabbitMQ with Spring Integration.
Common Challenges and Best Practices
1. Handling Message Serialization/Deserialization
One of the most common challenges when working with RabbitMQ and Spring Integration is ensuring seamless message serialization and deserialization. When messages are being transmitted between systems, it’s crucial to serialize them into a format that can be easily transmitted and deserialized at the receiving end.
In Spring Integration, this is typically handled using MessageConverters
. When configuring the outbound adapter, it’s important to specify the appropriate MessageConverter
to serialize the outbound message. Similarly, for the inbound adapter, the correct MessageConverter
should be configured to deserialize the incoming messages.
2. Managing Message Acknowledgment
Another challenge developers often encounter is managing message acknowledgment. In a messaging system, it’s important to ensure that messages are acknowledged only once they have been successfully processed. This prevents message loss and ensures message reliability.
In Spring Integration, the acknowledgment mode can be configured using the AcknowledgeMode
property. Setting the acknowledgment mode to AUTO
allows automatic acknowledgment, while setting it to MANUAL
requires explicit acknowledgment for each message. Choosing the appropriate acknowledgment mode depends on the specific requirements of your messaging system.
3. Dealing with Fault Tolerance
Fault tolerance is a critical aspect of any messaging system. In the context of RabbitMQ and Spring Integration, ensuring fault tolerance involves handling scenarios such as network interruptions, message processing failures, and broker outages.
One best practice for addressing fault tolerance is to implement retry and error-handling mechanisms. Spring Integration provides built-in components such as RequestHandlerRetryAdvice
and ExpressionEvaluatingRequestHandlerAdvice
to handle message processing retries and errors.
4. Scalability and Performance Optimization
Scalability and performance optimization are essential considerations when integrating RabbitMQ with Spring Integration. As message volumes and system loads increase, it’s crucial to ensure that the messaging system can scale seamlessly and maintain optimal performance.
To address scalability and performance concerns, developers should consider implementing features such as message prefetching, concurrent consumers, and proper resource management. These optimizations can ensure efficient message processing and overall system performance.
The Bottom Line
Integrating RabbitMQ with Spring Integration offers a powerful messaging solution for Java-based enterprise applications. However, it's important to be aware of and address the common challenges that may arise when working with these technologies. By understanding and implementing best practices for handling message serialization, acknowledgment, fault tolerance, scalability, and performance, developers can build robust and reliable messaging systems that meet the demands of modern enterprise environments.
In conclusion, the integration of RabbitMQ with Spring Integration presents an exciting opportunity to build efficient and scalable messaging solutions in Java, and with careful consideration of the common challenges and best practices discussed in this article, developers can overcome these hurdles and leverage the full potential of these technologies.
For further exploration of RabbitMQ and Spring Integration, check out the official RabbitMQ documentation and the Spring Integration reference guide. These valuable resources provide in-depth insights and additional information to enhance your understanding of these technologies.