Handling Common Issues with Groovy CLIBuilder
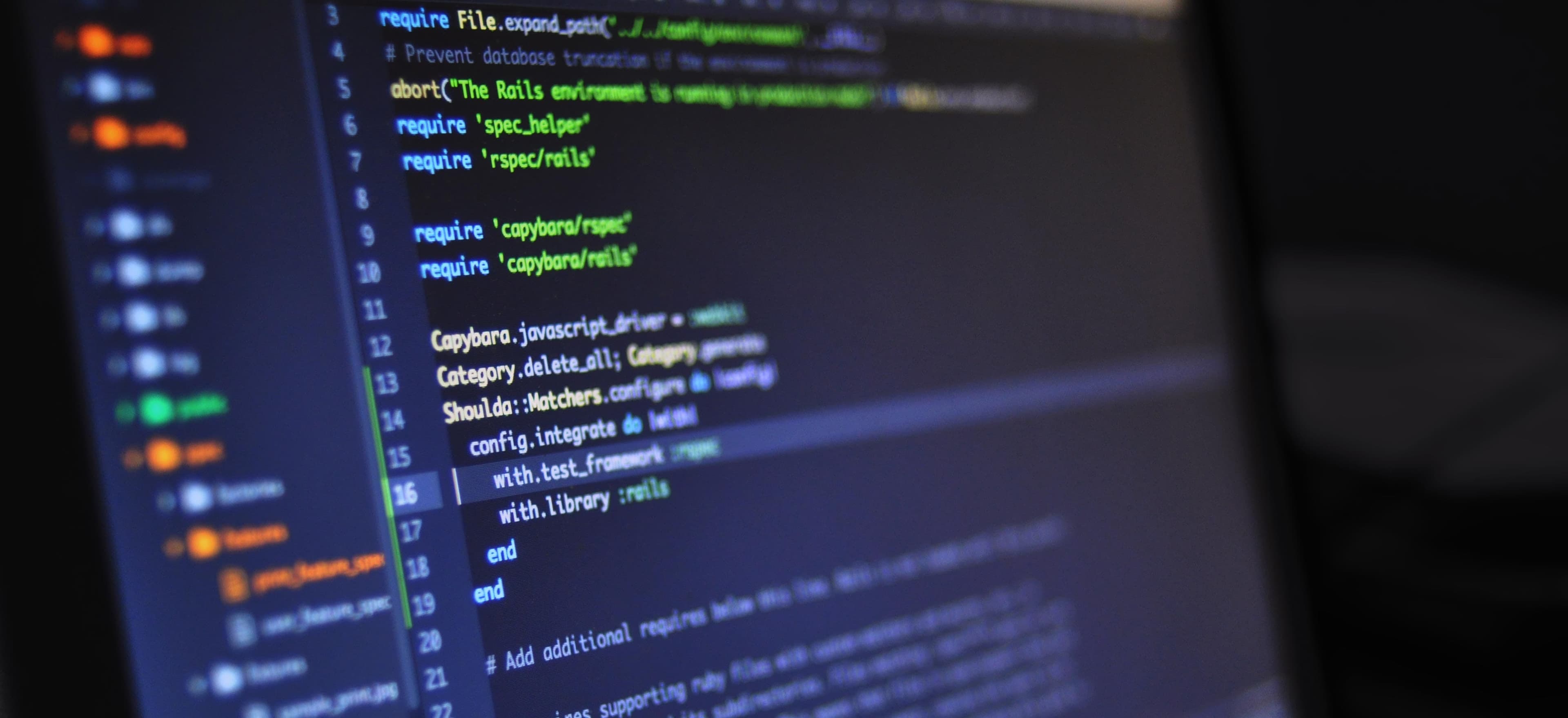
- Published on
A Complete Guide to Handling Common Issues with Groovy CLIBuilder
Groovy's CLIBuilder
is a powerful tool for creating command-line interfaces (CLI) in your Java applications. It provides a convenient way to define command-line options and arguments, validate user input, and execute commands based on the input provided. However, like any tool, CLIBuilder
is not without its challenges. In this article, we will explore common issues that developers may encounter when using CLIBuilder
and how to effectively address them.
1. Understanding the CLI Syntax
When working with CLIBuilder
, it's crucial to understand the syntax it uses for defining commands and options. The CLIBuilder
follows a convention similar to other popular CLI tools, such as git
or docker
, where commands and options are specified using a specific format.
Example of CLI Syntax
Let's say we have a simple CLI tool that takes a command greet
and two options --message
and --name
. The syntax for using this tool from the command line would look like this:
./my-cli greet --message "Hello" --name "John"
In this example, greet
is the command, and --message
and --name
are options with their corresponding values.
2. Handling Unknown Options and Commands
One common issue when using CLIBuilder
is handling unknown options and commands. When a user provides an option or command that is not recognized by the CLI, CLIBuilder
typically throws a MissingMethodException
or a similarly related exception.
To address this issue, you can define a custom error message for unknown options and commands. This can be achieved by implementing a custom CliBuilder
and overriding the handleUnparsed
method to provide a more user-friendly error message.
def cli = new MyCustomCLIBuilder()
def options = cli.parse(args)
if (options) {
// Handle parsed options
} else {
// Print custom error message
println "Unknown command or option. Use --help for usage information."
}
class MyCustomCLIBuilder extends CliBuilder {
@Override
protected void handleUnparsed(String[] unparsed) {
// Handle unknown options or commands here
// You can also provide custom error messages based on the unparsed input
}
}
By implementing a custom CliBuilder
and handling unparsed input, you can provide a better user experience when dealing with unknown options and commands.
3. Validating Option Values
Another common issue is validating the values provided for options. With CLIBuilder
, you can specify constraints such as required options, allowed values, and data types. However, it's essential to handle validation errors gracefully and provide meaningful feedback to the user.
Example of Option Validation
Let's consider an example where we have an option --age
that must be a positive integer. We can use CLIBuilder
to define this constraint and provide a custom error message for validation failures.
def cli = new CliBuilder()
cli.with {
age(type: Integer, { it > 0 }, 'Age must be a positive integer')
}
def options = cli.parse(args)
if (options) {
// Handle parsed options
} else {
// Print validation errors
cli.usage()
}
In this example, we define a constraint for the --age
option using the type
and a custom closure. If the validation fails, CLIBuilder
will automatically print the custom error message defined.
4. Managing Complex Command Structures
As CLI tools grow in complexity, managing command structures and their interactions can become a challenging task. CLIBuilder
provides support for defining nested commands, subcommands, and command hierarchies, but it's essential to design these structures thoughtfully to avoid confusion and ambiguity.
Example of Command Hierarchy
Consider a scenario where we have a CLI tool for managing users with commands like create
, update
, and delete
. We can use CLIBuilder
to define a clear command hierarchy to organize these operations.
def cli = new CliBuilder()
cli.with {
create {
// Define options for creating a user
}
update {
// Define options for updating a user
}
delete {
// Define options for deleting a user
}
}
def options = cli.parse(args)
// Handle parsed options based on the command hierarchy
By defining a clear command hierarchy, we can effectively manage complex command structures and make the CLI tool more intuitive for users.
5. Capturing User Input Interactively
In some cases, you may want your CLI tool to capture user input interactively, prompting the user for specific information during execution. While CLIBuilder
primarily focuses on parsing command-line arguments, you can integrate interactive input capture using standard input (e.g., System.in
).
Example of Interactive Input Capture
Suppose we want to prompt the user for additional information if certain options are not provided. We can use CLIBuilder
to parse the command-line arguments and then interactively capture the missing information.
def cli = new CliBuilder()
cli.with {
name(args: 1, 'User name')
}
def options = cli.parse(args)
if (options.name) {
// Handle parsed options
} else {
// Capture missing input interactively
println "Please enter the user name:"
def userName = System.console().readLine()
options.name = userName
// Proceed with handling parsed options
}
By combining CLIBuilder
with interactive input capture, you can create a more dynamic and user-friendly experience for your CLI tool.
To Wrap Things Up
In conclusion, while Groovy's CLIBuilder
provides powerful capabilities for building CLI tools, it's important to be mindful of common issues that may arise and how to effectively address them. By understanding the syntax, handling unknown options and commands, validating option values, managing complex command structures, and capturing user input interactively, you can enhance the usability and robustness of your CLI applications.
As you continue to work with CLIBuilder
and develop CLI tools, keep these considerations in mind to ensure a seamless and intuitive experience for both developers and end users.
For further information and in-depth tutorials on working with Groovy's CLIBuilder
, you can refer to the official documentation and Groovy CLI guide.