Scala: Choosing Between Case Class and Plain Class
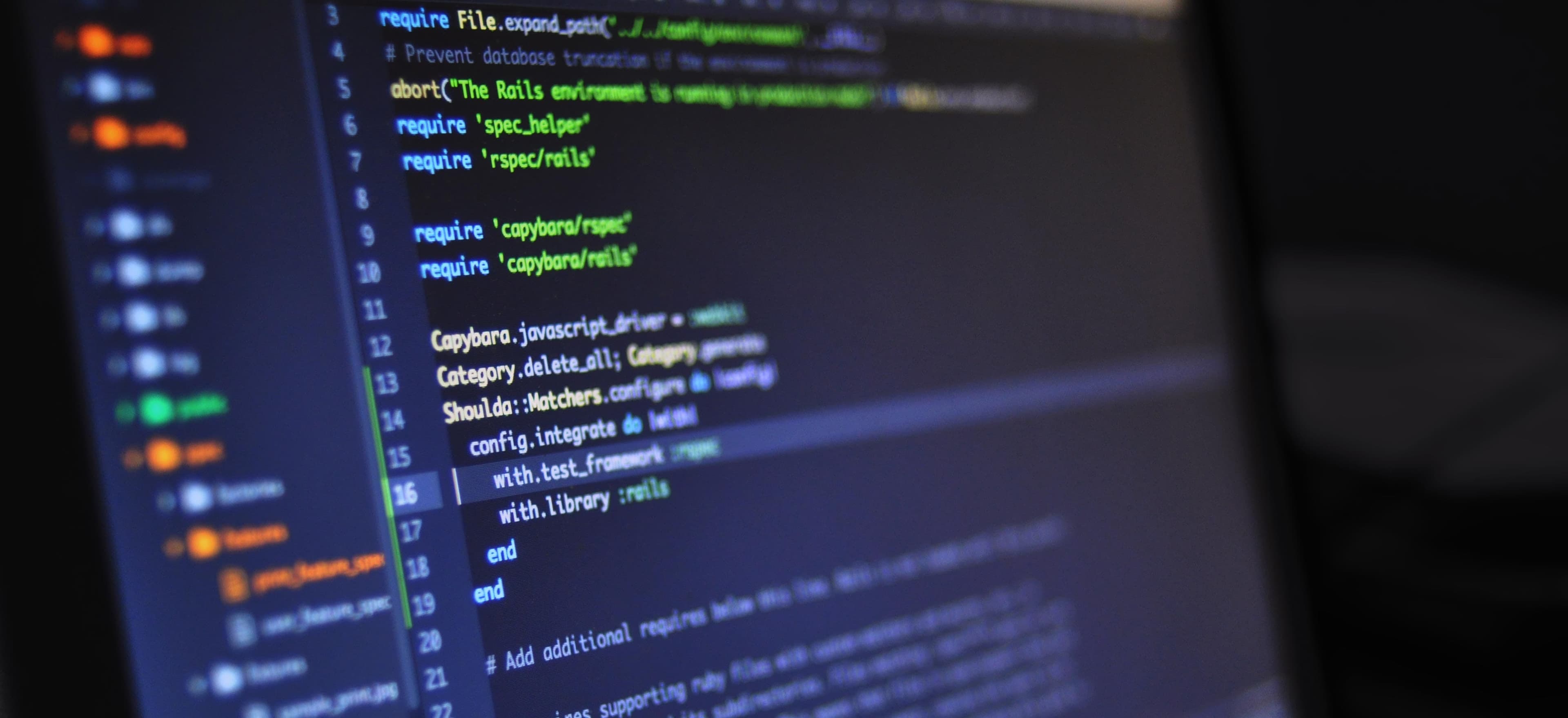
- Published on
Scala: Choosing Between Case Class and Plain Class
When working with Scala, one of the decisions developers often face is whether to use a case class or a plain class. Both options have their own advantages and use cases, and it's essential to understand the differences between the two in order to make an informed decision.
Understanding Case Classes
In Scala, a case class is a class that is immutable and lightweight. It is primarily used for modeling immutable data. When you define a case class, Scala automatically provides several useful functionalities, such as a default toString
method, equals
and hashCode
implementations, and a convenient companion object with apply methods for easy instantiation.
Let's take a look at an example of a simple case class:
case class Person(name: String, age: Int)
The above code defines a case class Person
with two fields: name
of type String
and age
of type Int
.
Benefits of Using Case Classes
Immutability
Case classes are immutable by default, meaning their instances cannot be modified after creation. This immutability ensures that once a case class instance is created, its state remains unchanged, making it ideal for representing immutable data.
Conciseness
Case classes eliminate the need for writing boilerplate code for commonly needed functionalities like toString
, equals
, and hashCode
methods. The compiler generates these methods for you, reducing the amount of code you need to write and maintain.
Pattern Matching
Pattern matching in Scala works seamlessly with case classes. This powerful feature allows you to destructure and match against the fields of a case class easily, making it suitable for working with complex data structures.
Value-based Equality
Case classes implement value-based equality by default, which means instances with the same field values are considered equal. This behavior aligns well with the concept of immutable data and makes comparisons straightforward.
Companion Object
Scala automatically generates a companion object for every case class, providing convenient factory methods for creating instances without using the new
keyword. This simplifies object instantiation and removes the verbosity often associated with creating new instances.
Use Cases for Case Classes
-
Data Modeling: Case classes are well-suited for representing and manipulating immutable data, making them an excellent choice for modeling domain objects, data transfer objects, and other data-centric structures.
-
Message Passing: When working with Akka or other actor-based systems, case classes are commonly used for defining messages due to their immutability and ease of serialization.
-
API Contracts: Case classes are often used to define the input and output data structures for APIs, ensuring that the data remains unchanged during processing.
Understanding Plain Classes
In contrast to case classes, plain classes in Scala do not come with the automatic generation of toString
, equals
, and hashCode
methods. These classes are mutable by default, and developers are responsible for implementing these methods and ensuring immutability as needed.
Let's consider a simple example of a plain class:
class Dog(var name: String, var age: Int)
The above code defines a plain class Dog
with two mutable fields: name
of type String
and age
of type Int
.
When to Use Plain Classes
Mutable State
If you need mutability and intend to modify the object's state after creation, plain classes may be more suitable. Unlike case classes, plain classes allow you to modify their fields, which can be advantageous in certain scenarios, such as building mutable data structures.
Explicit Control
Plain classes provide explicit control over how the class behaves and what methods are available. This level of control can be beneficial when you need to carefully manage mutability, equality, and other behaviors specific to your application's requirements.
Interoperability
In some cases, interoperability with Java classes may be a consideration. Plain classes can be more natural to work with when interacting with Java libraries that expect mutable objects and traditional getter/setter methods.
Making the Choice
When deciding between case classes and plain classes, consider the following factors:
-
Immutability: If immutability and value-based equality are essential for your use case, case classes are likely the better choice. They provide guarantees that instances will not be altered after creation, offering safety and predictability.
-
Boilerplate Code: If you aim to minimize boilerplate code and utilize the compiler's help to generate common methods, case classes can significantly reduce the amount of code you need to write and maintain.
-
Explicit Control: If you require mutable state and explicit control over class behavior, a plain class might be more appropriate. This could be the case when working with mutable data structures or closely interacting with Java code.
-
Interoperability: Consider the context in which your classes will be used. If interoperability with Java or other mutable-centric systems is a priority, a plain class may be the pragmatic choice.
The Closing Argument
In Scala, the decision to use a case class or a plain class depends on the specific requirements of your application, the nature of the data you are modeling, and your preferences regarding immutability and automatic method generation.
Case classes excel at representing immutable data and alleviating developers from writing repetitive code, while plain classes offer more explicit control over mutability and behavior. Understanding the nuances of each approach is crucial in making an informed choice that aligns with your application's needs and design principles.
By carefully evaluating the trade-offs and considering the use cases for each type of class, you can leverage the strengths of case classes and plain classes to build robust and maintainable Scala codebases.
For additional insights into Scala programming and best practices, check out Scala's official documentation.
Remember, the best choice ultimately depends on the specific context and requirements of your project. Happy coding!