Securing Java EE 8 Applications
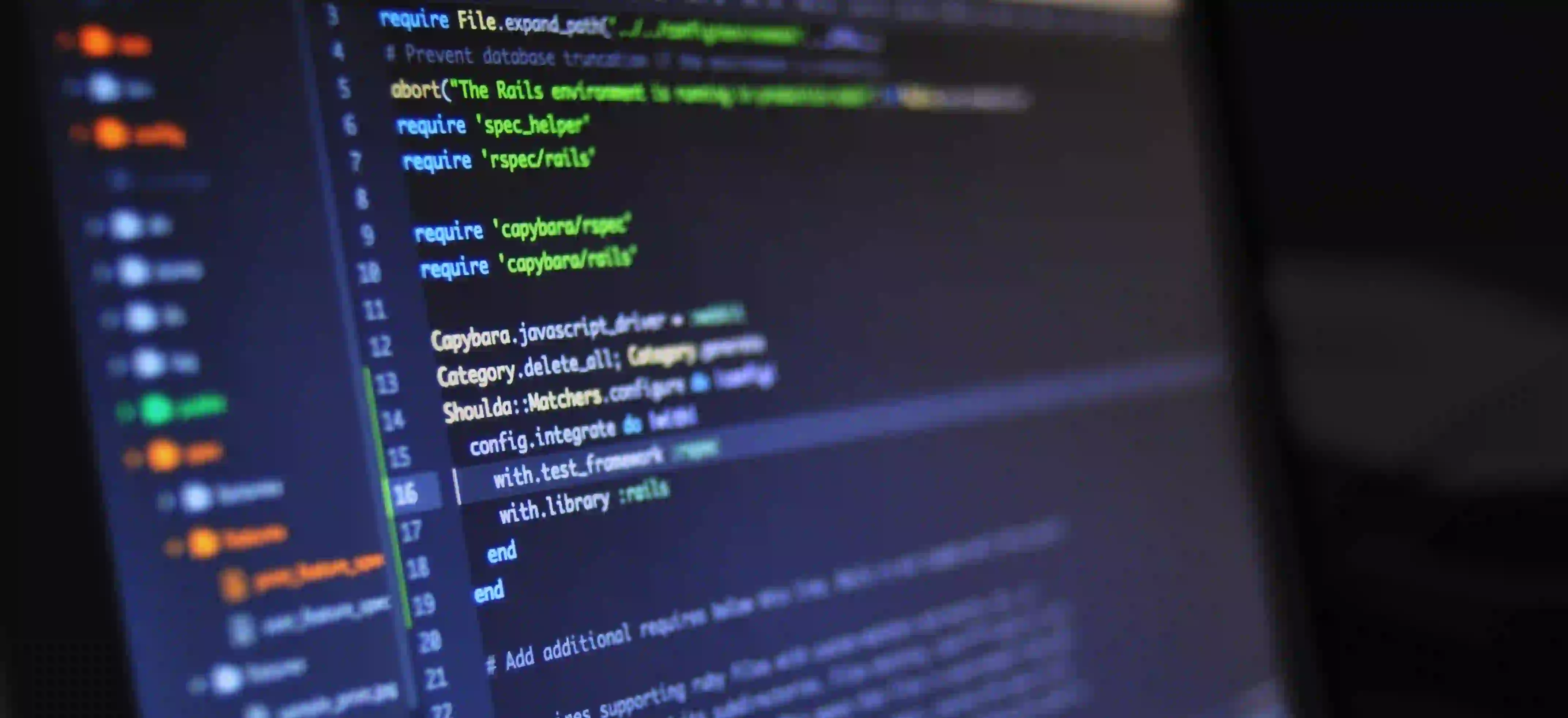
Securing Java EE 8 Applications
Securing a Java EE 8 application is crucial in protecting sensitive data and ensuring that only authorized users have access to certain resources. In this blog post, we will explore various security features and best practices for securing Java EE 8 applications.
Authentication and Authorization
Authentication and authorization are fundamental aspects of application security. Authentication is the process of verifying the identity of a user, while authorization determines what actions a user is allowed to perform within the application.
Java EE 8 provides robust support for authentication and authorization through mechanisms such as JAAS (Java Authentication and Authorization Service), Servlet security constraints, and CDI (Contexts and Dependency Injection) security.
Implementing Authentication with JAAS
Java EE 8 applications can leverage JAAS for authentication, which allows the use of pluggable authentication modules for different authentication mechanisms such as username/password, certificate-based, LDAP, etc.
Let's take a look at how to configure a simple JAAS login module in a Java EE 8 application:
public class CustomLoginModule implements LoginModule {
// Implementation of login module
}
In this example, we define a custom login module that implements the LoginModule
interface. This module can be configured in the web.xml
or using annotations to specify the authentication mechanism.
Implementing Authorization with Servlet Security Constraints
Servlet security constraints allow developers to specify fine-grained access control rules for web resources. This can be done declaratively using the web.xml
deployment descriptor or programmatically using annotations.
@ServletSecurity(
@HttpConstraint(rolesAllowed = {"ADMIN"})
)
public class AdminServlet extends HttpServlet {
// Servlet implementation
}
In this example, the AdminServlet
is restricted to users with the "ADMIN" role. This ensures that only authorized users can access the servlet.
Contextual Security with CDI
CDI provides contextual security to Java EE applications, allowing developers to apply security to beans and methods using annotations such as @RolesAllowed
, @PermitAll
, and @DenyAll
.
@Stateless
@DeclareRoles({"USER", "ADMIN"})
public class UserService {
@RolesAllowed("ADMIN")
public void deleteUser(User user) {
// Method implementation
}
}
In this example, the deleteUser
method is restricted to users with the "ADMIN" role. This ensures that only authorized users can invoke this method.
Transport Layer Security (TLS)
Transport Layer Security (TLS) is essential for securing the communication between clients and servers. Java EE 8 applications can leverage TLS by configuring the server for HTTPS and enforcing secure communication.
public class SecureServerConfig {
public void configureSSL() {
// Configure SSL context for HTTPS
}
}
By configuring the server for HTTPS and enforcing secure communication, Java EE 8 applications can ensure that all data exchanged between the client and the server is encrypted and secure.
Cross-Site Scripting (XSS) Protection
Cross-Site Scripting (XSS) is a common security vulnerability that can be mitigated through proper input validation and output encoding. Java EE 8 applications can utilize libraries like OWASP ESAPI to prevent XSS attacks.
String userInput = // User input
String safeOutput = ESAPI.encoder().encodeForHTML(userInput);
In this example, the user input is properly encoded using OWASP ESAPI to prevent XSS attacks. By implementing proper input validation and output encoding, Java EE 8 applications can protect against XSS vulnerabilities.
Resource Access Control
Controlling access to resources such as files, databases, and services is crucial for application security. Java EE 8 applications can use the Java EE Security API to enforce access control policies on resources.
@DeclareRoles("ADMIN")
@RolesAllowed("ADMIN")
public class AdminResource {
// Resource implementation
}
In this example, the AdminResource
class is restricted to users with the "ADMIN" role, enforcing access control policies on the resource.
The Closing Argument
Securing Java EE 8 applications is essential for protecting sensitive data and ensuring a secure user experience. By implementing robust authentication and authorization mechanisms, leveraging TLS for secure communication, mitigating common vulnerabilities like XSS, and enforcing access control policies on resources, Java EE 8 applications can achieve a high level of security.
For further reading on Java EE 8 security features, check out the official Oracle documentation and the Java EE Security API specification.
That concludes our discussion on securing Java EE 8 applications. Stay tuned for more Java-related content!