Maximizing Efficiency in Producer-Consumer Communication
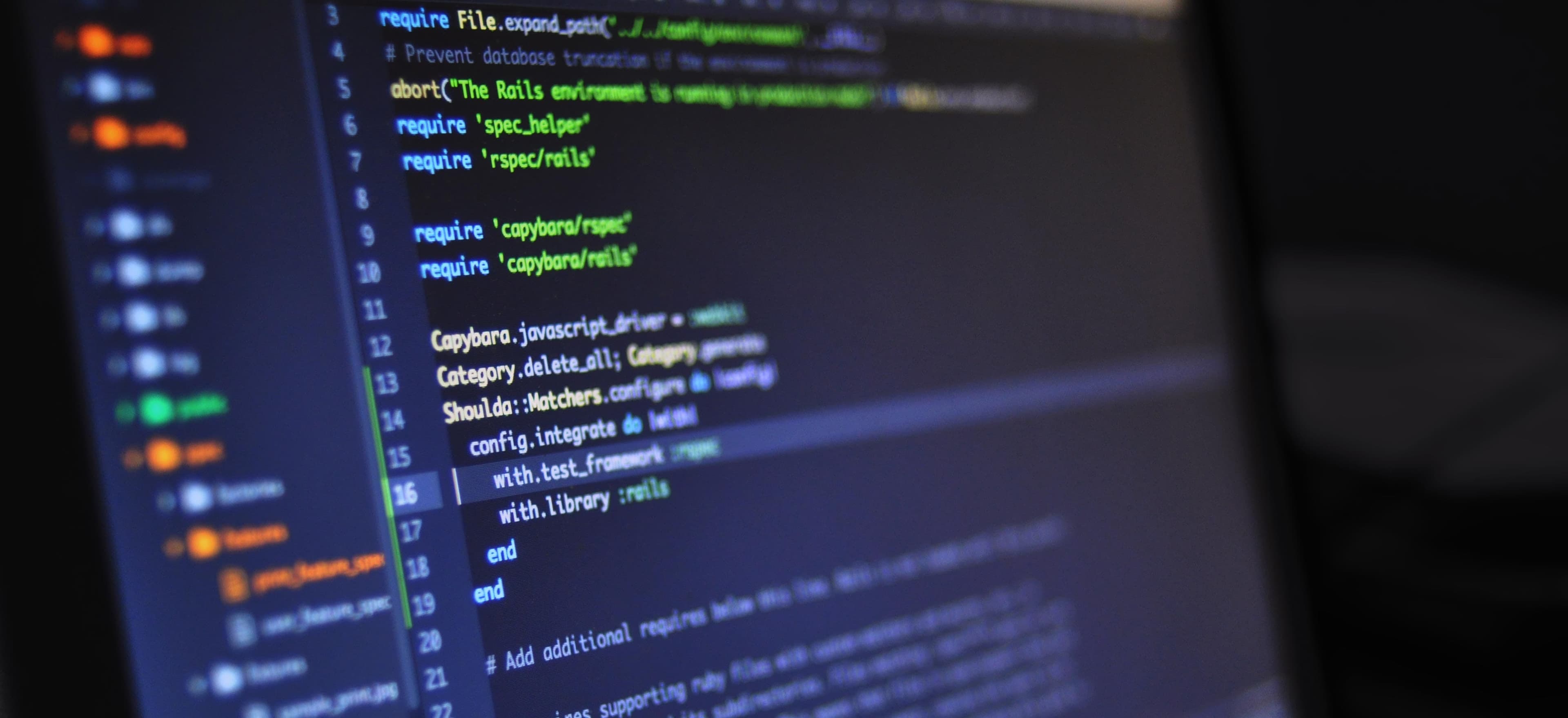
- Published on
Maximizing Efficiency in Producer-Consumer Communication
In concurrent programming, one of the common scenarios is when multiple threads need to communicate and share data. The producer-consumer problem is a classic example of such a scenario. In this problem, there are two entities - a producer that produces data, and a consumer that consumes the data. The challenge is to ensure that the producer and consumer operate efficiently, without data races, deadlocks, or resource wastage.
In Java, this can be achieved using various mechanisms such as wait-notify, locks, and concurrent data structures. In this blog post, we'll explore how to maximize efficiency in producer-consumer communication in Java, along with code examples and best practices.
Using BlockingQueue for Producer-Consumer Communication
One of the most straightforward and efficient ways to implement producer-consumer communication in Java is by using the java.util.concurrent.BlockingQueue
interface. This interface represents a thread-safe queue that supports operations such as put
(to insert elements) and take
(to remove and return elements). By using a blocking queue, we can ensure that the producer and consumer work efficiently without the need for explicit synchronization.
Let's consider a simple example where a producer produces items and puts them into a blocking queue, and a consumer consumes items from the same queue.
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ArrayBlockingQueue;
public class ProducerConsumerExample {
private static final int QUEUE_SIZE = 10;
private BlockingQueue<Integer> queue = new ArrayBlockingQueue<>(QUEUE_SIZE);
// Producer
public void produce() throws InterruptedException {
while (true) {
int item = /* produce item */;
queue.put(item); // Insert item into the queue
}
}
// Consumer
public void consume() throws InterruptedException {
while (true) {
int item = queue.take(); // Remove item from the queue
/* consume item */
}
}
}
In this example, the ArrayBlockingQueue
is used as the underlying implementation of the blocking queue. The producer produces items and puts them into the queue using the put
method, while the consumer consumes items from the queue using the take
method.
By using a blocking queue, we ensure that the producer and consumer can efficiently communicate without the need for explicit synchronization, as the queue takes care of the necessary thread safety.
Leveraging Condition Variables for Fine-Grained Control
While blocking queues provide a convenient way to implement producer-consumer communication, there are scenarios where we need more fine-grained control over the communication process. This is where the java.util.concurrent.locks.Condition
interface comes into play.
A condition variable is essentially a queue of threads that are waiting for a certain condition to become true. By using condition variables in conjunction with explicit locks, we can have more control over the signaling and waiting process in producer-consumer scenarios.
Let's consider a modified version of the producer-consumer example using condition variables for signaling between the producer and consumer.
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ProducerConsumerWithCondition {
private Lock lock = new ReentrantLock();
private Condition notFull = lock.newCondition();
private Condition notEmpty = lock.newCondition();
private static final int QUEUE_SIZE = 10;
private int[] queue = new int[QUEUE_SIZE];
private int count = 0;
private int putIndex = 0;
private int takeIndex = 0;
// Producer
public void produce(int item) throws InterruptedException {
lock.lock();
try {
while (count == queue.length) {
notFull.await();
}
queue[putIndex] = item;
putIndex = (putIndex + 1) % queue.length;
count++;
notEmpty.signal();
} finally {
lock.unlock();
}
}
// Consumer
public int consume() throws InterruptedException {
lock.lock();
try {
while (count == 0) {
notEmpty.await();
}
int item = queue[takeIndex];
takeIndex = (takeIndex + 1) % queue.length;
count--;
notFull.signal();
return item;
} finally {
lock.unlock();
}
}
}
In this example, we use a ReentrantLock
along with two condition variables - notFull
and notEmpty
- to control the signaling and waiting process. The producer waits on the notFull
condition variable when the queue is full, and it signals the notEmpty
condition variable after producing an item. Similarly, the consumer waits on the notEmpty
condition variable when the queue is empty, and it signals the notFull
condition variable after consuming an item.
By using condition variables and explicit locks, we have finer control over the producer-consumer communication, allowing for more sophisticated signaling and waiting mechanisms.
Summary
Efficient producer-consumer communication is crucial in concurrent programming to avoid bottlenecks, resource wastage, and synchronization issues. In this blog post, we explored two approaches to maximize efficiency in producer-consumer communication in Java.
-
Using
BlockingQueue
: This provides a simple and efficient way to implement producer-consumer communication without the need for explicit synchronization. -
Leveraging Condition Variables: By using condition variables in conjunction with explicit locks, we can achieve more fine-grained control over the signaling and waiting process, leading to a more sophisticated producer-consumer communication mechanism.
By choosing the right mechanism for producer-consumer communication based on the specific requirements of the application, developers can ensure efficient and scalable concurrent processing.
Remember to check out the official Java documentation for more in-depth information on concurrent programming and best practices.
Happy coding!
Checkout our other articles