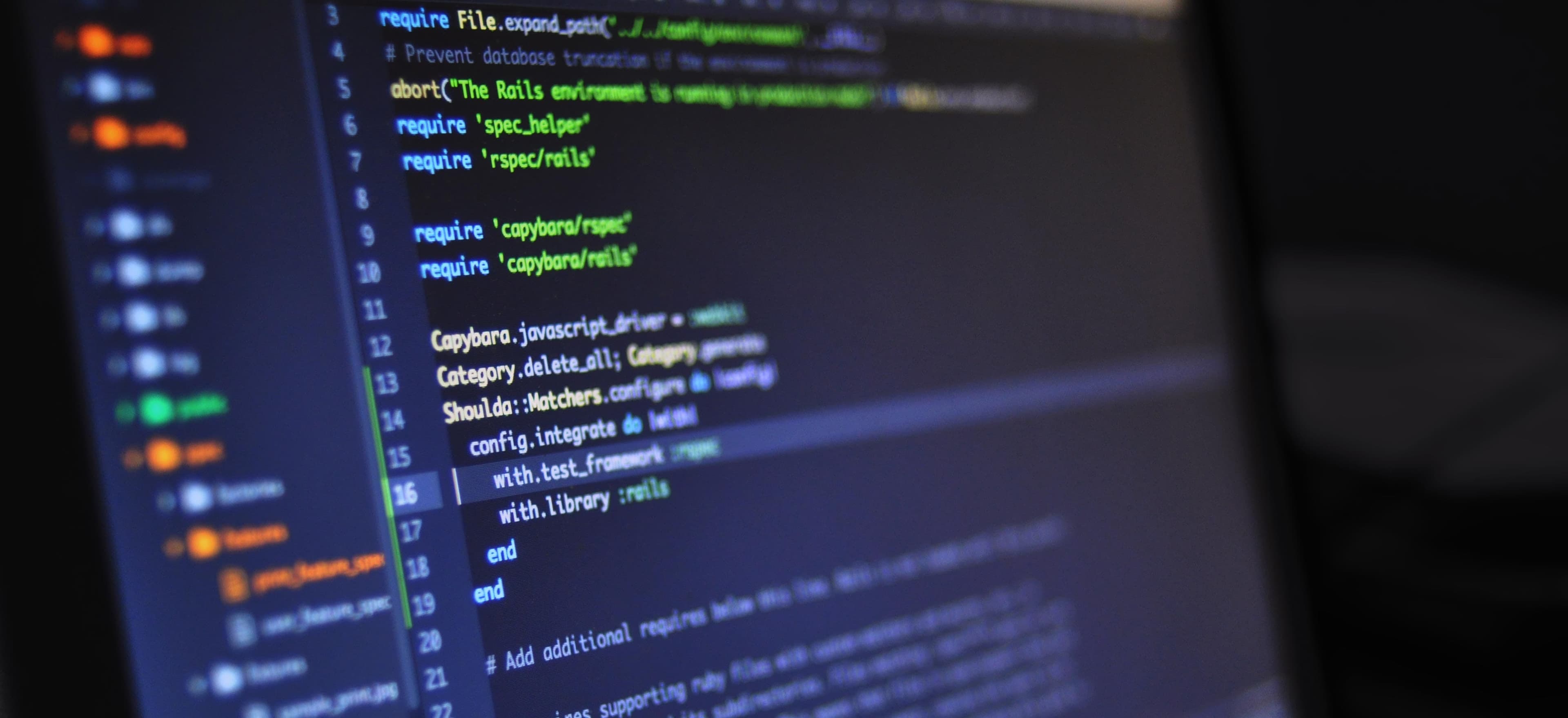
- Published on
Enhancing Password Security with Custom JSF Strength Indicator
In today's digital age, password security plays a crucial role in protecting sensitive information. With the increasing number of cyber attacks, it's more important than ever to ensure that passwords are strong and difficult to crack. One way to guide users in creating strong passwords is by providing a strength indicator that evaluates the complexity of the password in real time. In this blog post, we'll explore how to enhance password security in a JavaServer Faces (JSF) application by implementing a custom strength indicator for password fields.
Understanding Password Strength
Before delving into the implementation, it's essential to understand what contributes to the strength of a password. A strong password typically includes a combination of uppercase and lowercase letters, numbers, and special characters. The length of the password also plays a significant role in determining its strength. Additionally, using dictionary words, common phrases, or sequential characters should be discouraged to prevent easy guessing or brute-force attacks.
Implementing the Custom JSF Strength Indicator
To implement a custom strength indicator for password fields in a JSF application, you can leverage JavaScript to evaluate the password's complexity and reflect the strength visually. Here's a step-by-step guide on how to achieve this:
Step 1: Create a JSF Page
Start by creating a JSF page that includes an input field for the password and an element to display the strength indicator. Here's an example of the JSF page:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<title>Password Strength Indicator</title>
<script>
// JavaScript logic for strength indicator
</script>
</h:head>
<h:body>
<h:form id="passwordForm">
<h:outputLabel for="password" value="Password:"/>
<h:inputSecret id="password" value="#{passwordBean.password}" oninput="checkPasswordStrength()"/>
<div id="strengthIndicator"></div>
</h:form>
</h:body>
</html>
In this example, the h:inputSecret
component is used to capture the password input, and the oninput
attribute references a JavaScript function checkPasswordStrength()
that will evaluate the password's strength.
Step 2: Implement JavaScript Function
Next, create the JavaScript function checkPasswordStrength()
to assess the strength of the entered password based on predefined criteria. This function can calculate the password's strength by considering its length, complexity, and character types. Based on this evaluation, it can update the visual indicator to reflect the password's strength level.
function checkPasswordStrength() {
// Logic to evaluate password strength
// Update strength indicator based on the evaluation
}
The JavaScript function should dynamically update the strength indicator based on the user's input, providing real-time feedback on the password's strength.
Step 3: Integrate Backend Validation
While the strength indicator provides real-time feedback to the user, it's equally important to enforce strong password policies on the server side. Backend validation should be implemented to ensure that the password meets the specified criteria before being accepted. This can be achieved using JSF bean validation or custom validators to enforce complex password requirements.
By combining the front-end strength indicator with backend validation, a comprehensive approach to password security can be established within the JSF application.
The Last Word
In this blog post, we've demonstrated how to enhance password security in a JSF application by implementing a custom strength indicator for password fields. By providing real-time feedback to users regarding the strength of their passwords, they can be guided in creating stronger and more secure credentials. Additionally, the integration of backend validation ensures that strong password policies are enforced, further bolstering the application's security measures.
Ensuring robust password security is an ongoing endeavor, and staying abreast of the latest best practices and techniques is vital in safeguarding sensitive data from potential threats.
Implementing a custom strength indicator for password fields in JSF not only enhances security but also provides a seamless user experience, fostering a heightened sense of confidence in the application's security measures.
For further reading on JSF and password security, you may find the following resources helpful:
Incorporating these resources into your development practices can further strengthen your understanding of password security and its implementation in JSF applications.
By prioritizing password security and leveraging innovative methods such as custom strength indicators, developers can fortify their applications against potential security vulnerabilities while empowering users to create and maintain strong passwords.