Cracking the JVM: A Troubleshooting Guide
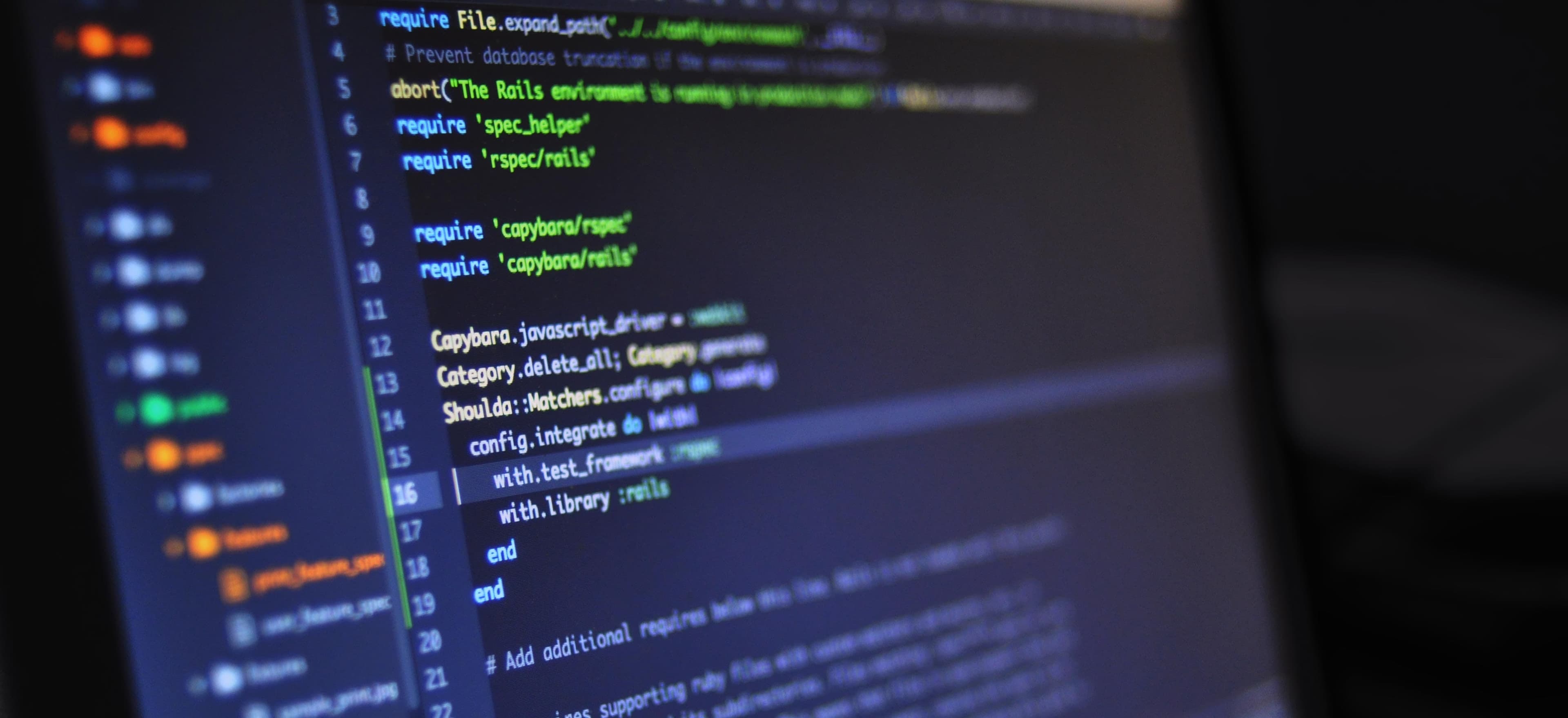
- Published on
Cracking the JVM: A Troubleshooting Guide
If you're working with Java, chances are you've encountered issues related to the Java Virtual Machine (JVM) at some point. Troubleshooting JVM problems can be challenging, but fear not! This comprehensive guide will equip you with the knowledge and tools to diagnose and solve common JVM issues.
Understanding the JVM
Before diving into troubleshooting, let's briefly understand the JVM. The JVM is the cornerstone of Java's "write once, run anywhere" principle. It provides a platform-independent execution environment that converts Java bytecode into machine code. Understanding this underlying process is crucial for effective troubleshooting.
Common JVM Issues
OutOfMemoryError
One of the most common issues Java developers face is the dreaded OutOfMemoryError
. This error occurs when the JVM runs out of memory to allocate new objects. It can manifest in different forms, such as java.lang.OutOfMemoryError: Java heap space
or java.lang.OutOfMemoryError: Metaspace
.
Troubleshooting OutOfMemoryError
To troubleshoot OutOfMemoryError
, start by analyzing heap dumps using tools like VisualVM or Eclipse MAT. Heap dumps provide insights into memory allocation and help identify potential memory leaks. Additionally, reviewing garbage collection logs can reveal patterns of memory consumption and GC activity.
// Example of increasing heap size
java -Xmx4G MyApp
Increasing the heap size using -Xmx
flag is a quick fix, but understanding memory usage patterns is crucial for long-term resolution.
ClassNotFound / NoClassDefFoundError
Classpath-related issues often lead to ClassNotFoundException
or NoClassDefFoundError
. These errors indicate that the JVM cannot find the required class files.
Troubleshooting Classpath Issues
Always double-check the classpath configuration, whether it's set through environment variables, command-line arguments, or build tools like Maven or Gradle. Tools like jdeps
can help analyze class dependencies and identify missing classes.
// Example of setting classpath
java -cp /path/to/classdir MyApp
Performance Degradation
Performance issues can stem from inefficient code, improper JVM configurations, or hardware limitations. Identifying the root cause of performance degradation is crucial for maintaining a responsive application.
Troubleshooting Performance Issues
Profiling tools like YourKit and JProfiler can pinpoint performance bottlenecks and CPU/memory hotspots. Analyzing thread dumps and using tools like jstack
can uncover thread contention and deadlocks.
// Example of generating thread dump
jstack <pid>
JVM Tuning and Optimization
Effective JVM tuning can significantly improve application performance and resource utilization. Understanding the available JVM options and their impact is essential for optimization.
Garbage Collection Optimization
Fine-tuning garbage collection settings can alleviate memory pressure and reduce pause times. Options like -XX:G1HeapRegionSize
, -XX:ConcGCThreads
, and -XX:G1ReservePercent
can be adjusted based on memory characteristics and workload patterns.
Just-In-Time (JIT) Compilation
JIT compilation optimizes bytecode at runtime, but its behavior can be customized using flags like -XX:CompileThreshold
and -XX:+PrintCompilation
. Monitoring JIT activity provides insights into which methods are being compiled and helps in identifying compilation-related issues.
Dealing with Native Memory Leaks
Native memory leaks, often caused by improper usage of external libraries or JNI, can lead to gradual resource exhaustion.
Troubleshooting Native Memory Leaks
Tools like jcmd
and jmap
can be used to analyze native memory consumption and track native memory leaks. It's also essential to review native code, native libraries, and JNI usage for potential memory leak sources.
// Example of using jcmd to collect native memory allocation
jcmd <pid> VM.native_memory summary
In Conclusion, Here is What Matters
Mastering JVM troubleshooting and optimization is crucial for delivering robust Java applications. By understanding common JVM issues, leveraging diagnostic tools, and embracing optimization techniques, you can conquer even the most complex JVM challenges. Remember, the key to effective troubleshooting lies in continuous learning and proactive problem-solving.
Now armed with the knowledge and strategies outlined in this guide, you're well-equipped to crack the JVM and elevate your Java development skills to new heights.
For further reference and advanced reading, consider exploring Oracle's JVM documentation and JVM ergonomics.
Happy troubleshooting, and may your Java applications run flawlessly!
Checkout our other articles