Understanding Cron and Simple Scheduling in Quartz
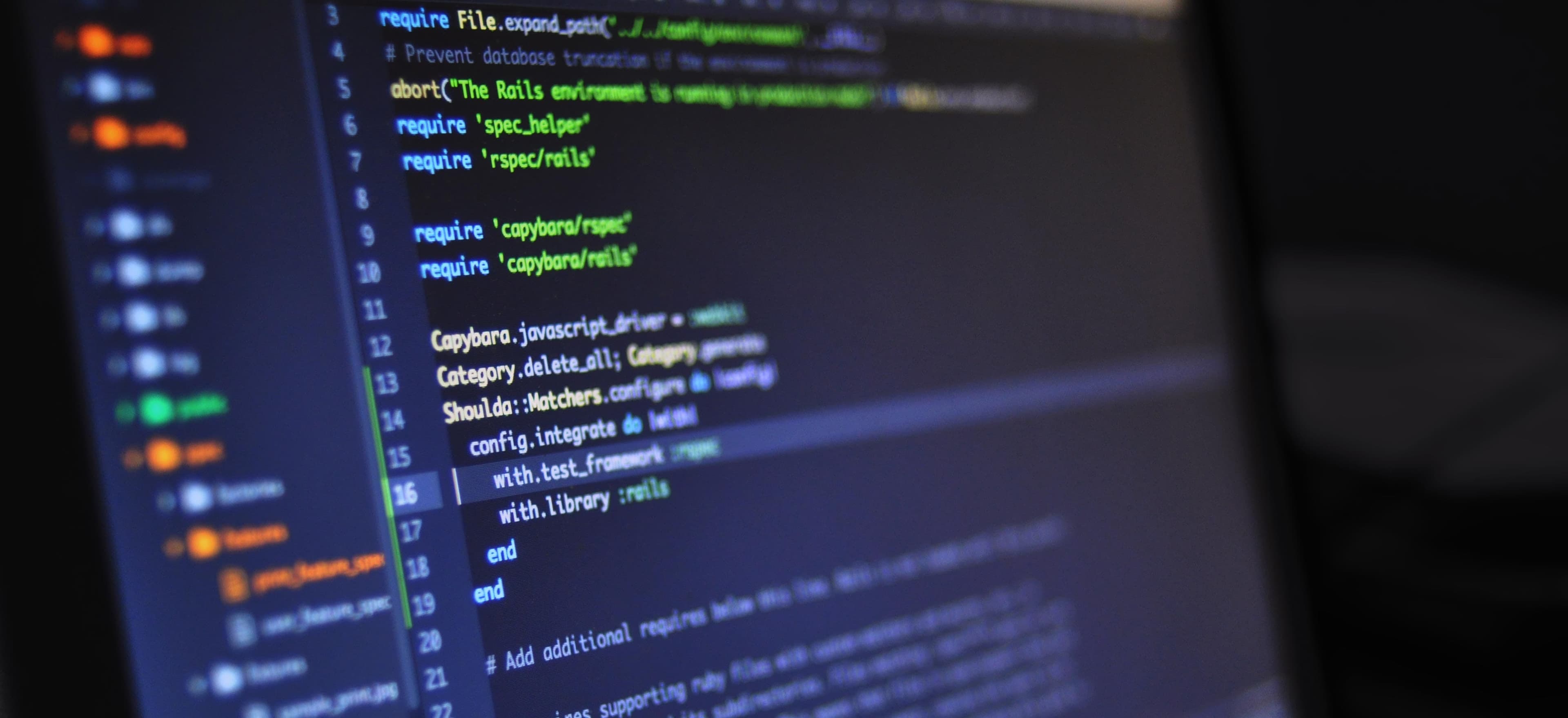
- Published on
Understanding Cron and Simple Scheduling in Quartz
Scheduling tasks in Java is a crucial aspect of many applications. Whether it's triggering a job at a specific time, running a job periodically, or defining complex schedules, having a reliable scheduling mechanism is essential. In the world of Java, Quartz is one of the most popular and efficient scheduling libraries. In this blog post, we will delve into the concepts of cron expressions and simple scheduling in Quartz, accompanied by code examples to solidify your understanding.
What is Quartz?
Quartz is a feature-rich, open-source job scheduling library that can be integrated into virtually any Java application. It provides support for scheduling jobs, including simple triggers and cron-based expressions, allowing developers to define complex schedules for their tasks. With Quartz, developers can create jobs that execute at specified intervals, on specific dates, or according to complex cron-based schedules.
Cron Expressions
An essential feature of Quartz is its support for cron expressions. Cron expressions are strings comprised of six or seven fields separated by white space. These fields represent a schedule based on time and date. The cron expression determines the schedule for a job by specifying the seconds, minutes, hours, day of month, month, and day of week when the job should be triggered.
Let's take a look at a simple example of a cron expression for scheduling a job to run every day at 8:00 AM:
String cronExpression = "0 0 8 * * ?";
In this expression:
- The first
0
represents the seconds. - The second
0
denotes the minute. - The
8
stands for the hour. - The
*
in the fourth position means it will run every day of the month. - The
*
in the fifth position indicates it will run every month. - The
?
in the last position specifies no specific day of the week.
This cron expression triggers the job every day at 8:00 AM with a second precision of 0.
Exploring the Fields
- Seconds (0-59): The seconds field allows values from 0 to 59 and governs which second of the minute the job will execute.
- Minutes (0-59): The minutes field specifies the minute within the hour, accepting values from 0 to 59.
- Hours (0-23): The hours field controls the hour of the day when the job will run, accepting values from 0 to 23.
- Day of Month (1-31): This field indicates the specific day of the month on which the job should be triggered, accepting values from 1 to 31.
- Month (1-12 or JAN-DEC): The month field determines the month of the year when the job will run. It accepts values from 1 to 12 or the corresponding names of the months (JAN-DEC).
- Day of Week (1-7 or SUN-SAT): The day of the week field specifies the particular day of the week when the job should execute, accepting values from 1 to 7 or the corresponding names of the days (SUN-SAT).
Simple Scheduling in Quartz
Quartz provides a straightforward way to schedule jobs using simple triggers, which allow jobs to be executed at a specific time, with options for repeat intervals. This functionality is useful for scenarios where tasks need to run at fixed intervals, such as every 10 minutes or hourly.
Let's explore a simple example of scheduling a job to run every 30 seconds using a simple trigger:
import org.quartz.*;
import org.quartz.impl.StdSchedulerFactory;
public class SimpleSchedulingExample {
public static void main(String[] args) throws SchedulerException {
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
JobDetail job = JobBuilder.newJob(SimpleJob.class)
.withIdentity("simpleJob")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("simpleTrigger")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(30)
.repeatForever())
.build();
scheduler.scheduleJob(job, trigger);
scheduler.start();
}
}
In this example, SimpleJob
is a simple implementation of the Job
interface, defining the task to be executed when the trigger fires. The SimpleScheduleBuilder
is used to create a trigger that will fire every 30 seconds indefinitely.
Why Use Cron Expressions and Simple Scheduling?
Flexibility and Precision
Cron expressions offer unparalleled flexibility in defining schedules. Whether it's a specific time of day, a range of dates, or complex recurring patterns, cron expressions can accommodate a wide array of scheduling requirements. This level of precision is indispensable for jobs that need to run at specific intervals or according to complex temporal patterns.
Integration with Job Logic
Quartz seamlessly integrates with the job logic through its Job
interface, allowing developers to encapsulate the task to be performed within the execute
method of the job class. This clean separation of concerns makes it easy to manage and maintain the job logic, while the scheduling details are handled separately through triggers and cron expressions.
Ease of Use and Configuration
Quartz provides a clean and intuitive API for defining and scheduling jobs, making it easy for developers to configure and manage their scheduled tasks. The simple, expressive syntax of cron expressions and simple triggers simplifies the process of defining job schedules, reducing complexity and enhancing maintainability.
Closing the Chapter
Scheduling tasks in Java with Quartz is a powerful and versatile approach for managing job execution. Whether you need to run jobs at specific times, recurring intervals, or according to complex schedules, Quartz provides the tools to accomplish these tasks effectively. Understanding the concepts of cron expressions and simple scheduling in Quartz empowers developers to create robust and reliable scheduling mechanisms for their applications.
In this post, we've explored the fundamentals of cron expressions, delving into their syntax and usage for defining job schedules in Quartz. We've also demonstrated how simple scheduling can be achieved using Quartz's intuitive API, emphasizing the ease of configuration and integration with job logic. By mastering these scheduling techniques, developers can elevate the functionality and reliability of their Java applications.
By embracing the power of Quartz, developers can unlock a world of possibilities for automating and orchestrating tasks, bringing efficiency and precision to their applications.
So, go ahead and leverage the capabilities of Quartz to schedule your tasks with precision and ease!
To further explore Quartz and its capabilities, check out the official Quartz documentation and take a deeper dive into the advanced scheduling features it offers.
Happy scheduling!