Troubleshooting Issues with AChartEngine in Android Apps
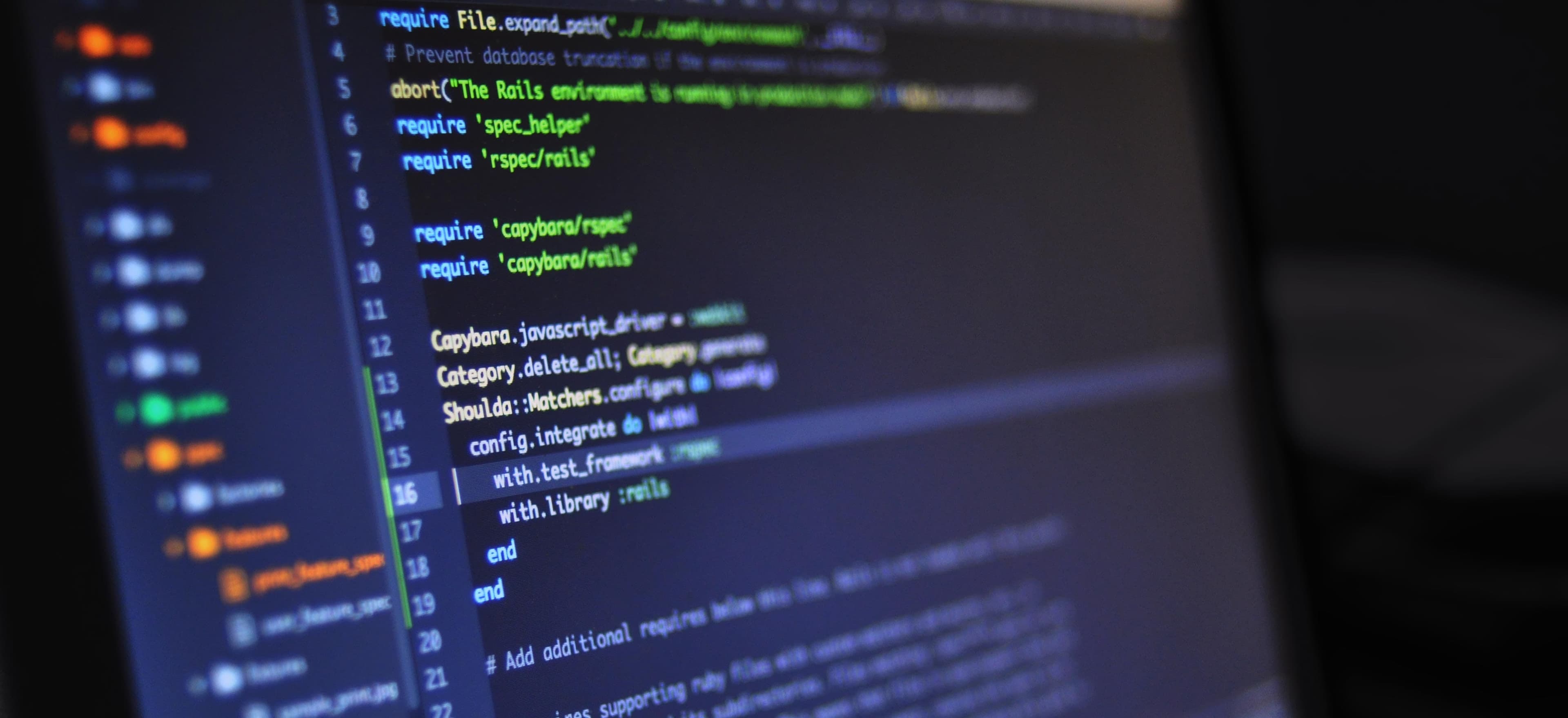
- Published on
Troubleshooting Issues with AChartEngine in Android Apps
AChartEngine is a popular charting library for Android apps, known for its ease of use and customization options. However, like any other technology, it is not immune to issues that might arise during development and integration. In this blog post, we will discuss some common problems developers encounter when using AChartEngine in their Android apps, and provide solutions to address these issues.
Problem 1: Customizing Chart Appearance
One common issue developers face is customizing the appearance of charts to meet specific design requirements. AChartEngine provides a wide range of customization options, including colors, line styles, fonts, and more. However, figuring out the correct way to apply these customizations can be challenging.
Solution:
When customizing the appearance of a chart, it's important to familiarize yourself with the AChartEngine documentation to understand the available customization options. Additionally, carefully review the provided code examples and sample projects to see how different customizations are applied.
Here's an example of customizing the appearance of a line chart:
// Creating a dataset to hold series
XYMultipleSeriesDataset dataset = new XYMultipleSeriesDataset();
// Adding a series to the dataset
XYSeries series = new XYSeries("Sample Chart");
dataset.addSeries(series);
// Customizing the appearance of the series
XYSeriesRenderer renderer = new XYSeriesRenderer();
renderer.setColor(Color.BLUE);
renderer.setPointStyle(PointStyle.CIRCLE);
renderer.setFillPoints(true);
// Creating a renderer for the dataset
XYMultipleSeriesRenderer mRenderer = new XYMultipleSeriesRenderer();
mRenderer.addSeriesRenderer(renderer);
In this example, we create a dataset and customize the appearance of the series using a renderer. We set the color to blue, choose a circular point style, and specify that the points should be filled.
Problem 2: Handling Performance Issues with Large Datasets
Another common challenge is dealing with performance issues when working with large datasets. Rendering and interacting with large amounts of data can lead to sluggish behavior and decreased responsiveness in the app.
Solution:
To address performance issues with large datasets, consider implementing the following techniques:
-
Data Sampling: If the dataset is excessively large, consider implementing data sampling to reduce the number of data points displayed on the chart without sacrificing the overall representativeness of the data.
-
Asynchronous Rendering: Implement asynchronous rendering to offload the chart rendering process to a background thread, ensuring that the main UI thread remains responsive.
-
Optimizing Rendering Code: Review the code responsible for rendering the chart and look for opportunities to optimize performance, such as minimizing redundant calculations or drawing operations.
// Implement asynchronous rendering
new AsyncTask<Void, Void, Bitmap>() {
@Override
protected Bitmap doInBackground(Void... params) {
// Code to render the chart in the background and return the resulting bitmap
return chart.toBitmap();
}
@Override
protected void onPostExecute(Bitmap bitmap) {
// Update the UI with the rendered chart bitmap
imageView.setImageBitmap(bitmap);
}
}.execute();
Problem 3: Handling Chart Interactions and Gestures
Integrating interactive features such as zooming, panning, and tapping on data points can be challenging, especially for developers new to AChartEngine.
Solution:
AChartEngine provides built-in support for chart interactions and gestures, allowing users to zoom, pan, and interact with data points effortlessly. By enabling these features, you can enhance the user experience and make the charts more engaging.
// Enabling zoom and pan interactions
mRenderer.setZoomButtonsVisible(true);
mRenderer.setZoomEnabled(true);
mRenderer.setPanEnabled(true, true);
In the above code snippet, we enable zoom and pan interactions by setting the corresponding properties of the renderer. This allows users to zoom in and out of the chart and pan across different sections.
Problem 4: Dealing with Deprecated Features
As with any software library, AChartEngine undergoes updates and improvements, leading to deprecation of certain features. Developers may encounter issues when using deprecated methods or classes in their existing codebase.
Solution:
When dealing with deprecated features, it's essential to stay updated with the latest releases and migration guides provided by the AChartEngine team. These resources offer insights into deprecated features, alternative approaches, and best practices for migrating to newer versions.
Additionally, leverage the Android developer documentation to explore alternative libraries or built-in charting capabilities provided by newer Android SDK versions.
The Closing Argument
In this blog post, we discussed some common issues developers encounter when using AChartEngine in their Android apps, along with practical solutions to address these challenges. By understanding how to customize chart appearance, handle performance issues, enable chart interactions, and deal with deprecated features, developers can harness the full potential of AChartEngine while delivering compelling charting experiences in their Android apps.
Remember, thorough understanding of the library's documentation, regular updates, and exploration of alternative approaches are essential for effectively troubleshooting and optimizing AChartEngine in Android app development.
For further information and detailed documentation, visit the official AChartEngine website and the Android Developer Guides.