Unleashing the Power of Observer Pattern
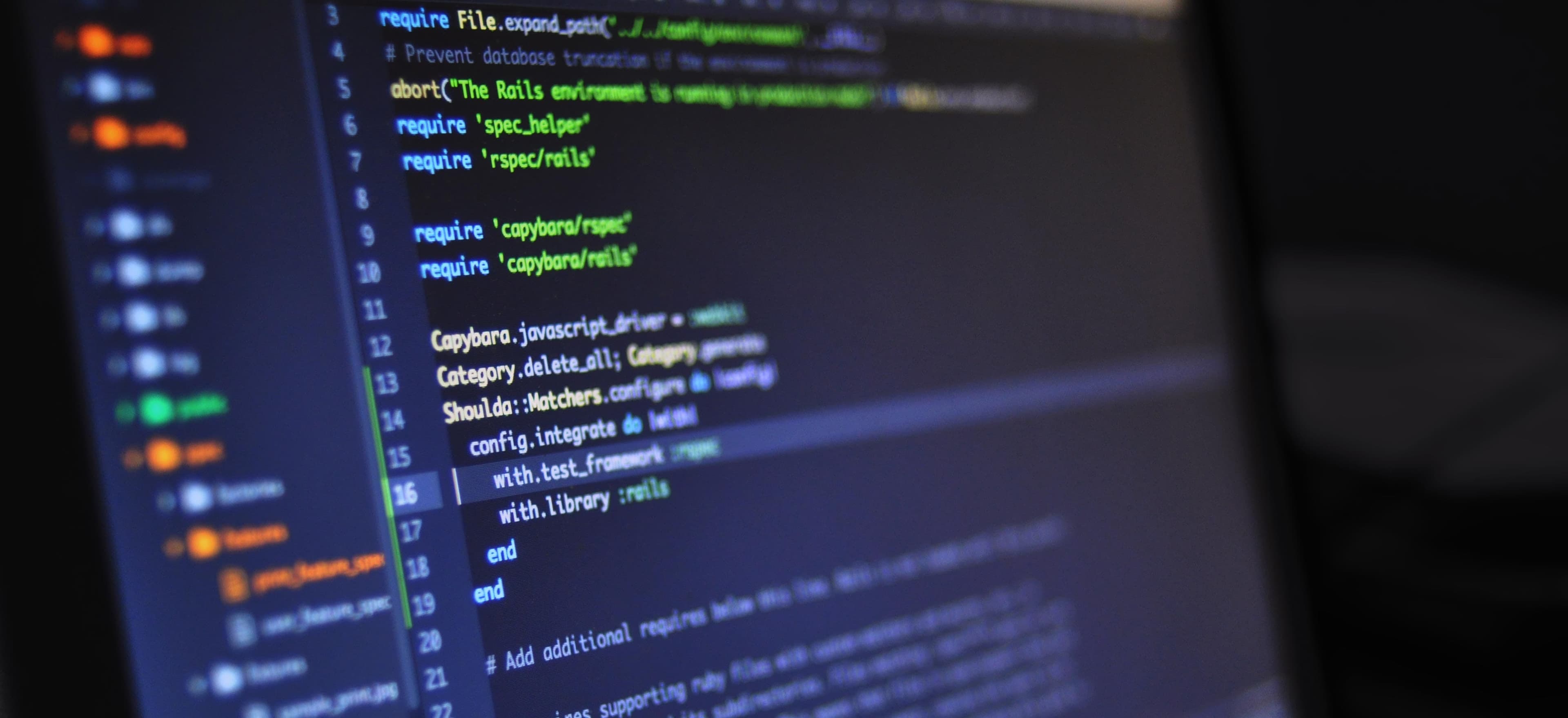
- Published on
Unleashing the Power of Observer Pattern
In the world of software development, design patterns play a crucial role in building robust and maintainable systems. One such design pattern is the Observer pattern, which is widely used to establish relationships between objects. The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. In this blog post, we will explore the Observer pattern, its implementation in Java, and how it can be leveraged to write efficient and loosely coupled code.
Understanding the Observer Pattern
At its core, the Observer pattern consists of two main components: the Subject and the Observers. The Subject is the object being observed, and it holds a list of Observers to notify when its state changes. The Observers are the dependent objects that are notified and updated when the Subject changes.
The key idea behind the Observer pattern is that the Subject does not need to know anything about its Observers, and the Observers are not tightly coupled to the Subject. This loose coupling allows for a more flexible and maintainable design, making it easier to add new Observers or modify existing ones without impacting the Subject.
Implementing the Observer Pattern in Java
Let's dive into a simple implementation of the Observer pattern in Java. We will create a basic example where a Subject (publisher) notifies its Observers (subscribers) when a new message is published.
import java.util.ArrayList;
import java.util.List;
// Subject interface
interface Subject {
void registerObserver(Observer observer);
void removeObserver(Observer observer);
void notifyObservers();
}
// Concrete subject
class MessagePublisher implements Subject {
private List<Observer> observers = new ArrayList<>();
private String message;
@Override
public void registerObserver(Observer observer) {
observers.add(observer);
}
@Override
public void removeObserver(Observer observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(message);
}
}
public void setMessage(String message) {
this.message = message;
notifyObservers();
}
}
// Observer interface
interface Observer {
void update(String message);
}
// Concrete observer
class MessageSubscriber implements Observer {
private String name;
public MessageSubscriber(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received message: " + message);
}
}
In this example, we have defined a Subject
interface with methods to register, remove, and notify observers. The MessagePublisher
class implements the Subject
interface and maintains a list of observers. When a new message is set, it notifies all registered observers by calling their update
method.
The Observer
interface declares the update
method, and the MessageSubscriber
class implements this interface to receive and display the messages.
Leveraging the Observer Pattern
The Observer pattern can be leveraged in various scenarios, including event handling, model-view-controller (MVC) architectural pattern, and reactive programming.
Event Handling
In graphical user interface (GUI) programming, the Observer pattern is commonly used for event handling. Components such as buttons, checkboxes, and text fields act as Subjects, while the event listeners (e.g., action listeners, item listeners) act as Observers. When an event occurs (e.g., a button click), the Subject notifies its Observers, triggering the appropriate actions.
Model-View-Controller (MVC) Architectural Pattern
The Observer pattern forms the cornerstone of the MVC architectural pattern. In this pattern, the model represents the Subject, and the views are the Observers. When the model's state changes, it notifies the views to update their presentation.
Reactive Programming
In modern reactive programming paradigms, such as RxJava, the Observer pattern is fundamental. Data streams (Subjects) emit events, and subscribers (Observers) react to those events, enabling a scalable and responsive application design.
Lessons Learned
The Observer pattern is a powerful tool for building loosely coupled and maintainable software systems. It promotes separation of concerns and enables objects to communicate without knowing about each other's specifics. By leveraging the Observer pattern in Java, developers can create flexible and extensible applications that are easier to maintain and extend.
In this blog post, we've explored the fundamentals of the Observer pattern, implemented a simple example in Java, and discussed its practical applications. By understanding and harnessing the power of the Observer pattern, developers can elevate their software design and architecture to new heights.
To delve deeper into design patterns, consider exploring Gang of Four (GoF) design patterns book by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides.
Now, it's time to unleash the power of the Observer pattern in your Java projects and take your software development skills to the next level.
Happy coding!