Big Data Implementation Challenges: Overcoming Data Volume Limits
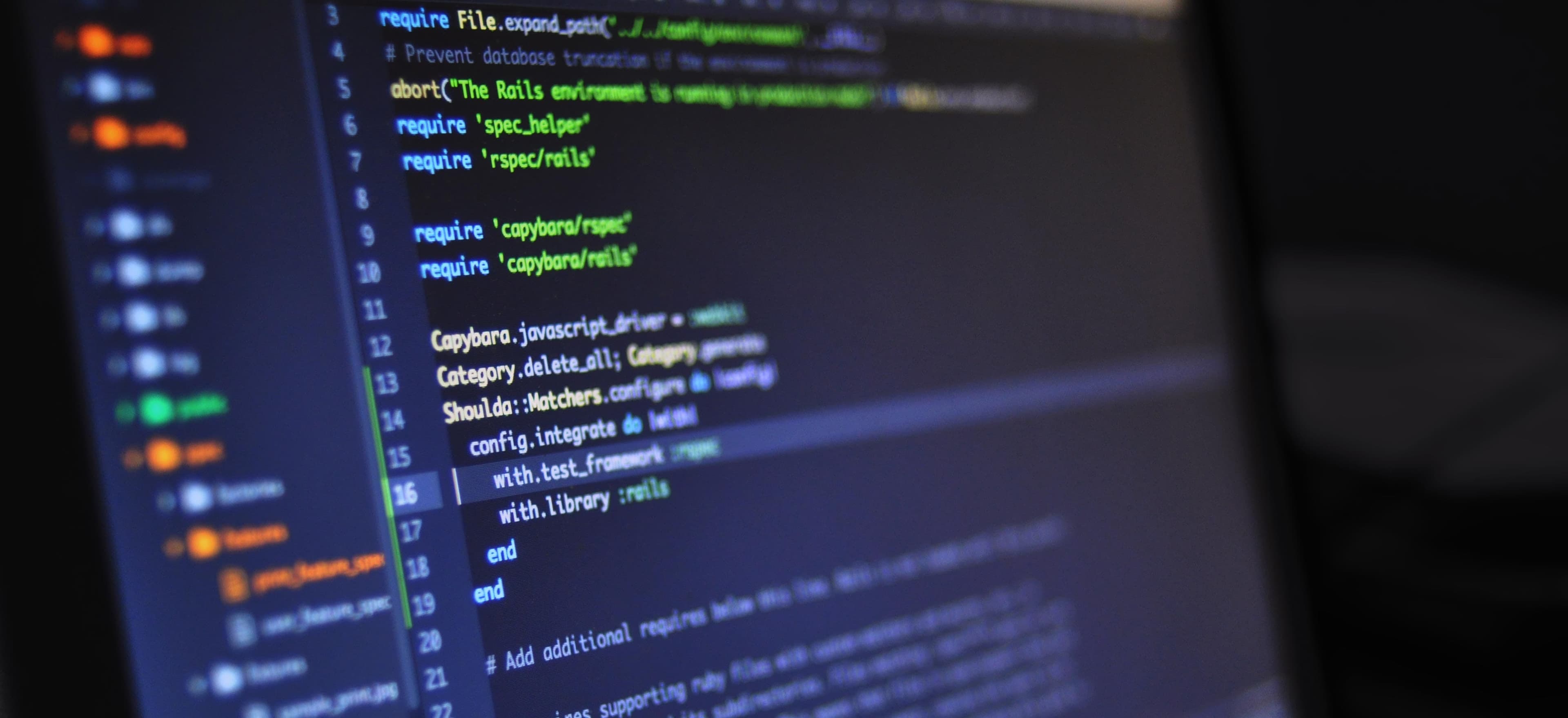
- Published on
Big Data Implementation Challenges: Overcoming Data Volume Limits
In the realm of big data, one of the most pressing challenges that organizations face is dealing with the ever-increasing volume of data. As the amount of data generated continues to soar, traditional systems are struggling to keep up, leading to a host of implementation hurdles. In this article, we will delve into the complexities of managing large volumes of data and explore effective strategies to overcome these limitations.
The Growing Pains of Big Data
In today's data-driven world, the proliferation of digital devices, IoT sensors, and online transactions has resulted in an unprecedented deluge of information. The sheer volume of data being generated on a daily basis has outpaced the capabilities of traditional data management tools. This exponential growth presents a myriad of challenges for organizations looking to harness the power of big data for actionable insights.
Understanding Data Volume Limits
The limitations of traditional database management systems become glaringly evident when confronted with the mammoth scale of big data. These systems are typically designed to handle structured data within predefined schemas, and they struggle to cope with the unstructured and semi-structured data that characterizes big data. As a result, organizations are confronted with storage, processing, and performance bottlenecks, impeding their ability to extract value from the wealth of data at their disposal.
Overcoming Data Volume Limits with Java
Java, with its robust ecosystem and scalability, has become a go-to choice for building big data solutions. Leveraging Java for overcoming data volume limits involves a strategic approach that encompasses various facets of data management and processing. Let's explore some key strategies and techniques for leveraging Java in the realm of big data.
Distributed Computing with Apache Hadoop
Apache Hadoop, a prominent player in the big data landscape, offers a distributed file system (HDFS) and a framework for the distributed processing of large data sets across clusters of computers. Java serves as the primary language for developing applications in the Hadoop ecosystem, making it an indispensable tool for tackling data volume limits.
public class WordCount {
public static void main(String[] args) throws Exception {
// Create a new Hadoop configuration
Configuration conf = new Configuration();
// Create a job
Job job = Job.getInstance(conf, "word count");
// Set the input and output paths
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// Specify the mapper and reducer classes
job.setMapperClass(TokenizerMapper.class);
job.setReducerClass(IntSumReducer.class);
// Set the output key and value classes
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
// Execute the job and wait for completion
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
The above code snippet showcases a basic Word Count job in Hadoop, highlighting how Java is used to define the data processing logic through mappers and reducers.
Streamlining Data Processing with Apache Spark
Apache Spark, another powerful framework in the big data domain, provides lightning-fast cluster computing. Built around the concept of resilient distributed datasets (RDDs), Spark simplifies the processing of large-scale data. Java seamlessly integrates with Spark, offering the flexibility to develop complex data processing pipelines with ease.
public class SparkExample {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("Spark Example").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
int sum = rdd.reduce((a, b) -> a + b);
System.out.println("Sum: " + sum);
}
}
In the provided code snippet, Java is used to create a simple Spark application that calculates the sum of elements in an RDD, showcasing the seamless integration of Java with Spark for data processing tasks.
Taming the Data Deluge: Summary
As organizations grapple with the formidable challenge of managing soaring data volumes, Java emerges as a linchpin in the quest to overcome data volume limits. By harnessing the capabilities of distributed computing frameworks like Hadoop and Spark, coupled with the flexibility and scalability of Java, enterprises can navigate the complexities of big data with confidence.
In conclusion, the era of big data demands a strategic blend of robust technologies and innovative approaches to effectively tackle the data volume limits. Java, with its inherent strengths in distributed computing and data processing, stands out as a formidable asset in the arsenal of tools available to organizations seeking to harness the power of big data.
By leveraging Java's prowess in distributed computing, organizations can not only address the challenges posed by burgeoning data volumes but also unlock valuable insights that drive informed decision-making. In the dynamic landscape of big data, Java remains a steadfast ally in the relentless pursuit of overcoming data volume limits.
In this comprehensive guide, we explored the challenges posed by escalating data volumes in the big data domain and elucidated how Java, alongside distributed computing frameworks like Apache Hadoop and Apache Spark, can serve as a potent antidote to these challenges. Embracing Java's capabilities for streamlined data processing empowers organizations to conquer the hurdles associated with data volume limits, paving the way for informed, data-driven strategies. If you're interested in delving deeper into Java for big data applications, consider exploring Java's official documentation. For insights into Apache Hadoop and Apache Spark, refer to the official documentation at Hadoop and Spark, respectively.
Checkout our other articles