Maximizing Software Testing ROI: Overcoming Budget Constraints
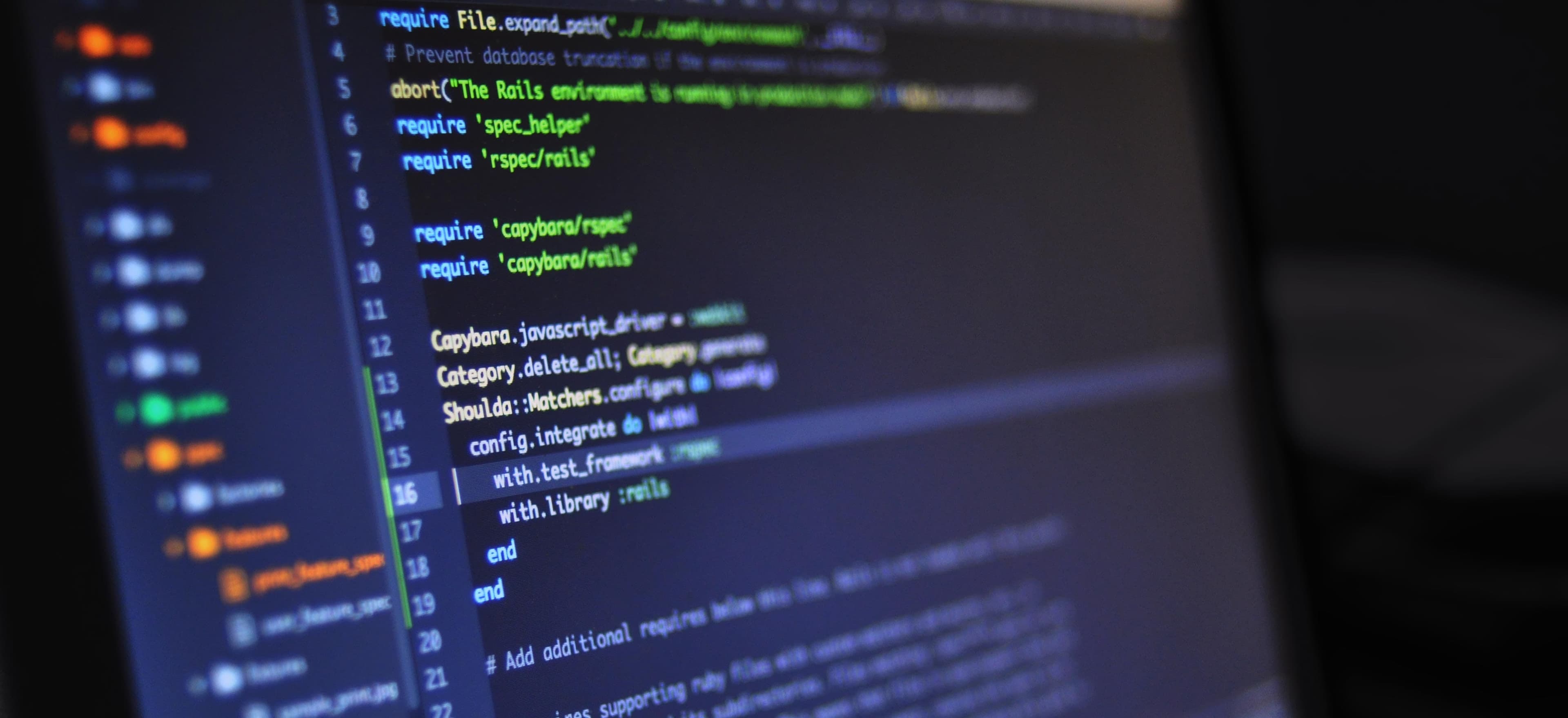
- Published on
Maximizing Software Testing ROI: Overcoming Budget Constraints
In today's software development landscape, there is an inherent pressure to deliver high-quality software within tight budget and time constraints. This creates a challenge for testing teams to effectively maximize their return on investment (ROI) while ensuring comprehensive test coverage. In this article, we'll explore strategies for overcoming budget constraints and maximizing the ROI of software testing, with a focus on Java.
The Importance of Software Testing ROI
Software testing is not just about finding and fixing bugs. It plays a crucial role in ensuring that the software meets the specified requirements, performs reliably, and provides a positive user experience. However, testing efforts need to be balanced against the resources allocated to testing, making the concept of ROI crucial in the testing process.
Leveraging Automation to Increase ROI
One of the most effective ways to maximize testing ROI is through the implementation of test automation. In the context of Java, tools like Selenium and JUnit provide a robust framework for automating tests. Let's take a look at a simple example of a JUnit test case:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyMathTest {
@Test
public void testAddition() {
MyMath myMath = new MyMath();
int result = myMath.add(2, 3);
assertEquals(5, result);
}
}
In this example, the MyMathTest
class contains a test case for the add
method of the MyMath
class. When executed, this test case automates the process of verifying the correctness of the add
method, thereby increasing testing efficiency and ROI.
Prioritizing Test Cases Based on Risk
Not all test cases are created equal. Prioritizing test cases based on risk can help optimize testing efforts and maximize ROI. By identifying critical areas of the software and allocating more testing resources to them, teams can ensure that high-impact areas are thoroughly tested, even within budget constraints.
Utilizing Mocking Frameworks for Efficient Testing
In Java development, mocking frameworks such as Mockito and PowerMockito can be invaluable in creating mock objects for testing. By simulating the behavior of complex, dependent components, these frameworks enable more targeted and efficient testing.
@Test
public void testPaymentSuccess() {
PaymentGateway paymentGateway = mock(PaymentGateway.class);
when(paymentGateway.processPayment(anyDouble())).thenReturn(true);
PaymentProcessor paymentProcessor = new PaymentProcessor(paymentGateway);
boolean result = paymentProcessor.makePayment(100.00);
assertTrue(result);
}
In this example, the test case creates a mock PaymentGateway
object to simulate a successful payment process. By isolating the PaymentProcessor
class and focusing solely on its functionality, the testing process becomes more efficient and cost-effective.
Continuous Integration and Deployment for Timely Feedback
Integrating testing into the continuous integration and deployment (CI/CD) pipeline is essential for obtaining timely feedback on the quality of the software. Jenkins and Travis CI are popular CI/CD tools that seamlessly integrate with Java projects, enabling automated testing and immediate identification of issues.
By running tests in parallel pipelines and catching issues early in the development process, teams can avoid costly reworks and enhance overall ROI.
Collaborative and Agile Testing Practices
Adopting an agile approach to testing encourages collaboration between developers, testers, and stakeholders. By involving all relevant parties throughout the development lifecycle, teams can gain a shared understanding of requirements and eliminate rework caused by misunderstandings or misinterpretations.
Furthermore, agile methodologies such as Scrum promote iterative development, allowing for frequent testing and feedback cycles. This iterative approach significantly reduces the cost of rework and enhances the overall ROI of testing efforts.
The Closing Argument
Maximizing software testing ROI within budget constraints is a challenging yet achievable goal. By leveraging automation, prioritizing test cases, utilizing mocking frameworks, integrating with CI/CD pipelines, and embracing agile practices, testing teams can effectively optimize their testing efforts and ensure a high ROI.
In the fast-paced world of software development, the ability to deliver high-quality software within budget constraints is a competitive advantage. By following the strategies outlined in this article, Java testing teams can navigate budget constraints while maximizing their ROI and contributing to the overall success of software projects.
Remember, the goal of testing is not just to find bugs, but to enhance the value of the software by delivering a reliable and high-quality product. Embracing these strategies will not only improve testing ROI but also elevate the reputation of the development team as a whole.
For further reading on ROI optimization in software testing, check out this article by TechBeacon, which provides additional insights and best practices for maximizing testing ROI in software development.