Optimizing Code: Best Practices for Using Enums and Annotations
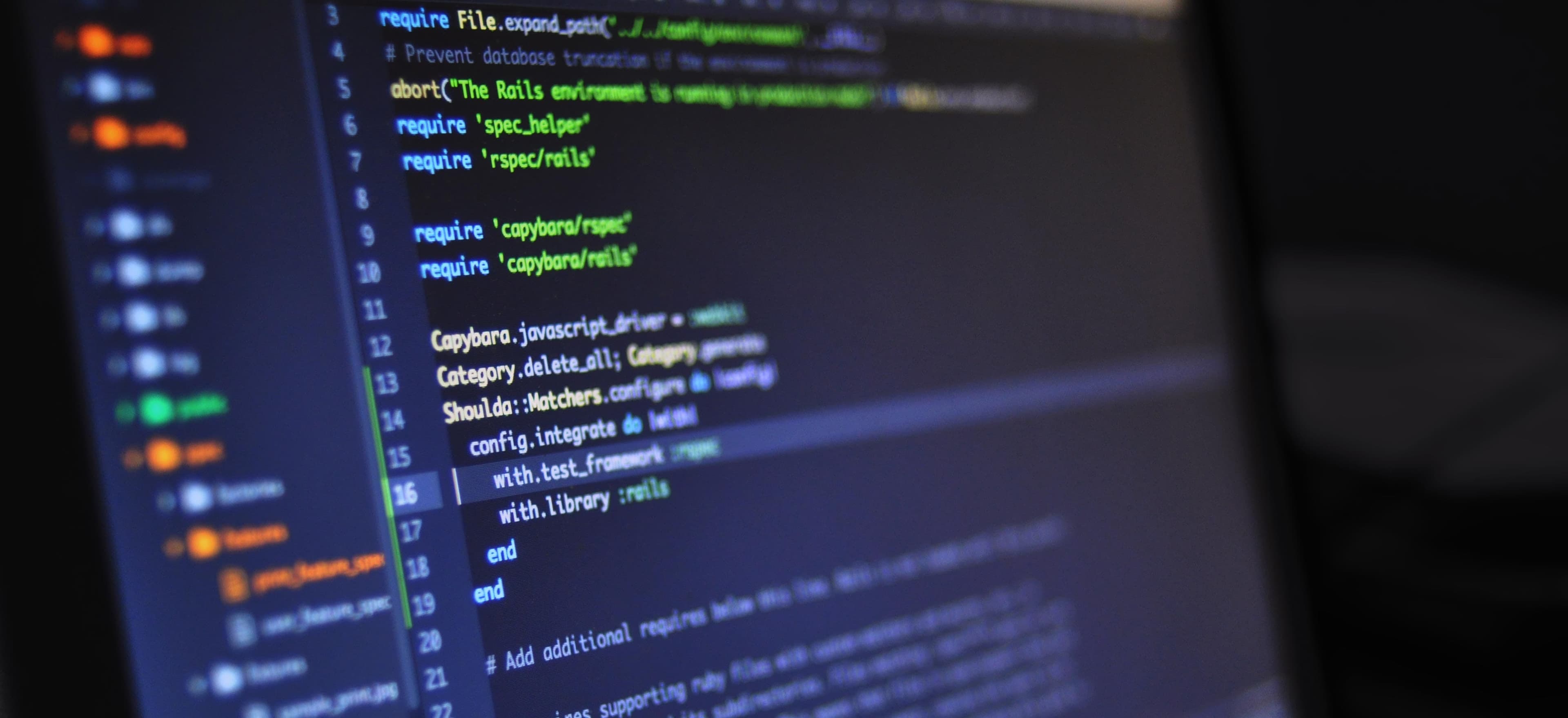
- Published on
Optimizing Code: Best Practices for Using Enums and Annotations
In the world of Java programming, making the right choices in utilizing language features can significantly impact the performance and maintainability of your code. Two key features that play a crucial role in writing efficient and maintainable Java code are enums and annotations. In this article, we will explore best practices for using enums and annotations, and how to optimize their usage.
Enums: Use Them Wisely
Enums in Java are a powerful way to define a fixed set of constants. They are used to represent a fixed number of states or options. While enums offer type safety and readability, improper usage can lead to inefficiencies.
1. Use Enums for Constant Values
When you have a fixed set of constant values that are known at compile time, enums are the way to go. For example, consider a set of directions: North, South, East, and West. Instead of using string literals or integer constants, defining an enum provides clarity and type safety.
public enum Direction {
NORTH, SOUTH, EAST, WEST
}
2. Avoid Excessive Enums
Avoid defining enums that represent a large or variable set of values. Enumerating a large number of items can lead to increased memory usage and longer initialization times. If the values are not known at compile time or can change dynamically, consider using a different data structure, such as a database or configuration file.
3. Use EnumMap and EnumSet for Efficiency
When dealing with a fixed set of enum constants and their corresponding data, prefer using EnumMap
and EnumSet
for efficient storage and retrieval. They are specifically designed to work with enums and provide better performance than using other collections in combination with enums.
EnumMap<Direction, String> directionMap = new EnumMap<>(Direction.class);
directionMap.put(Direction.NORTH, "N");
directionMap.put(Direction.SOUTH, "S");
4. Enum Constructors, Methods, and Fields
Enums can have constructors, methods, and fields like regular classes. Use these features when the enum constants require additional behavior or data. For example, you can provide a method to retrieve a display name for each enum constant.
public enum Direction {
NORTH("N"), SOUTH("S"), EAST("E"), WEST("W");
private String abbreviation;
Direction(String abbreviation) {
this.abbreviation = abbreviation;
}
public String getAbbreviation() {
return abbreviation;
}
}
Annotations: Make Their Intent Clear
Annotations provide a way to add metadata to Java code, allowing for additional information to be conveyed. When used effectively, annotations can improve code readability, reduce boilerplate code, and enable better tooling support.
1. Clearly Document Annotation Usage
When creating custom annotations, always document their purpose, usage, and any required elements or attributes. Clear documentation helps other developers understand the intent and proper usage of the annotation.
/**
* Annotation to indicate that a method is deprecated and should be replaced with a newer implementation.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface DeprecatedReplacement {
String newImplementation();
}
2. Use Built-In Annotations
Java provides a set of built-in annotations that serve various purposes, such as @Override
, @Deprecated
, and @SuppressWarnings
. Familiarize yourself with these annotations and use them where appropriate to convey specific meanings to the compiler, tools, or other developers.
3. Apply Annotations Sparingly
While annotations can be powerful, overusing them can clutter the code and reduce readability. Apply annotations judiciously, focusing on essential scenarios where they provide significant value, such as marking methods as deprecated or injecting dependencies.
4. Leverage Annotation Processors
Annotation processors allow for code generation and additional validation at compile time based on annotated elements. Utilize annotation processors to automate repetitive tasks, validate code, and generate boilerplate code, thus improving code quality and reducing manual effort.
The Closing Argument
By following best practices for using enums and annotations, you can write more efficient, maintainable, and readable Java code. Enums should be used for representing fixed sets of constants, while annotations should convey clear and useful metadata. Make wise choices in utilizing these language features, and your Java code will be optimized for performance and maintainability.
Remember, while enums and annotations are powerful tools, the key to effective and optimized code lies in using them judiciously and with clear intent.
For further reading, you can explore the official documentation on Enums and Annotations.
Checkout our other articles