Troubleshooting Common Spring Framework Issues
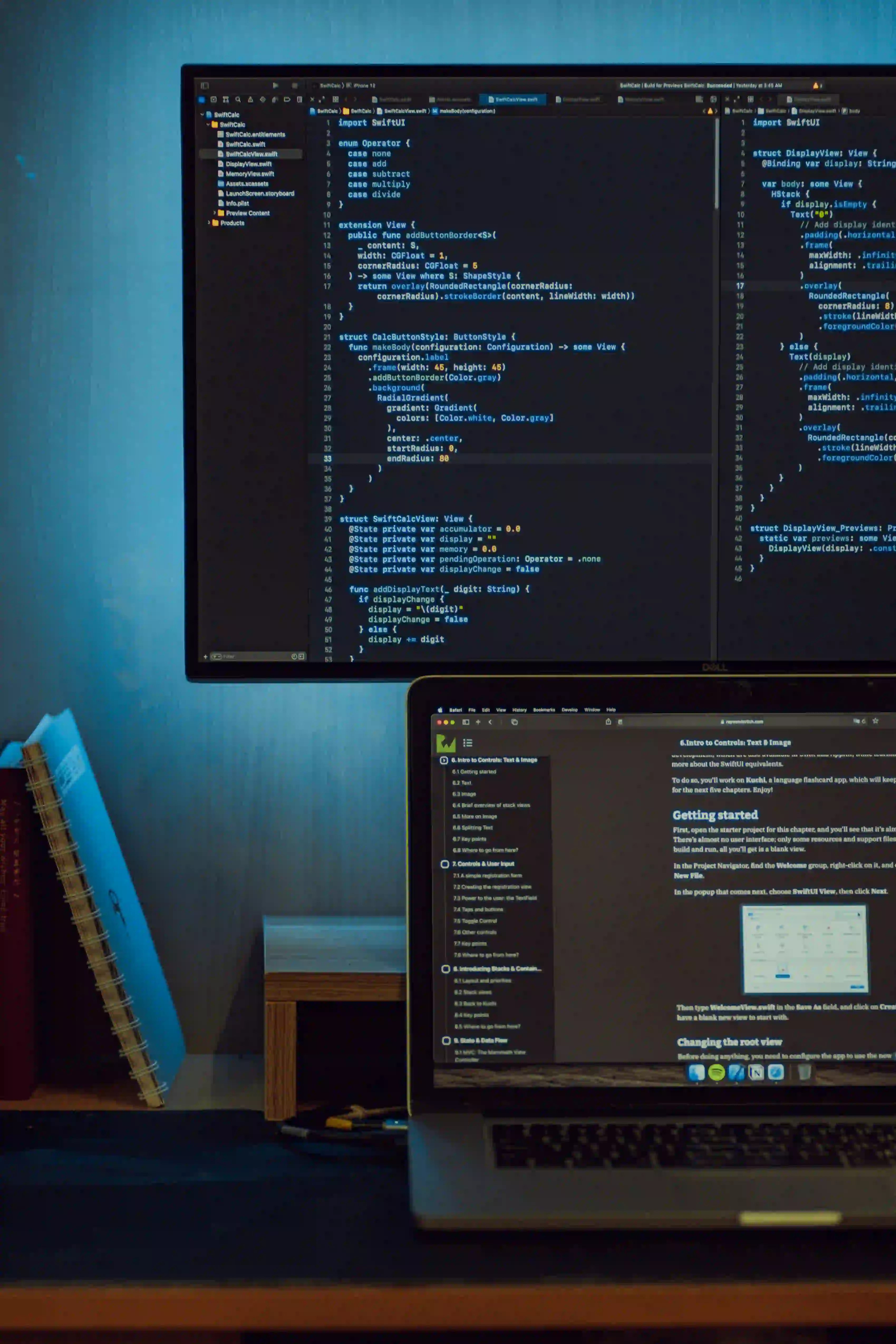
Troubleshooting Common Spring Framework Issues
When working with the Spring Framework, developers may encounter various issues that can hinder the development process. Some of these issues can be puzzling and time-consuming to resolve. In this article, we will explore some common Spring Framework issues and how to troubleshoot them effectively.
Issue 1: Bean Not Getting Autowired
A common issue that developers face is when a Spring bean is not getting autowired where it is expected to be. This can happen due to various reasons such as incorrect bean configuration, package scanning, or component scanning.
Troubleshooting Steps:
-
Check Component Scan Configuration: Ensure that the package containing the bean is being scanned by the component scan. You can check the component scan configuration in the main application class using the
@ComponentScan
annotation.☕snippet.java@SpringBootApplication @ComponentScan(basePackages = "com.example") public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
Here,
basePackages
should include the package where the bean is located. -
Verify Bean Annotation: Double-check that the bean you are trying to autowire is annotated properly, such as with
@Component
,@Service
, or@Repository
, depending on its role. -
Check for Multiple Implementations: If there are multiple implementations of the same interface, use the
@Qualifier
annotation to specify which implementation to inject.
By following these steps, you can effectively troubleshoot and resolve issues related to beans not getting autowired in the Spring Framework.
For more detailed information, you can refer to the Spring Framework documentation on autowiring beans.
Issue 2: Transaction Management
Another common issue that often arises in the Spring Framework is related to transaction management. When transactions are not working as expected, it can lead to data inconsistencies and integrity issues in the application.
Troubleshooting Steps:
-
Check Transactional Annotation: Verify that the
@Transactional
annotation is being used at the appropriate method or class level where the transaction management is required.☕snippet.java@Service @Transactional public class UserService { // ... }
-
Transaction Propagation: Ensure that the transaction propagation is configured correctly based on the requirement. Check if the propagation type (
REQUIRED
,REQUIRES_NEW
, etc.) aligns with the intended behavior. -
Database Configuration: Verify the database configuration in the application properties or XML configuration file. Ensure that the datasource, JPA, or Hibernate settings are correctly specified.
By meticulously examining these aspects, you can effectively troubleshoot and resolve issues related to transaction management in the Spring Framework.
Further details on transaction management can be found in the Spring documentation on Declarative Transaction Management.
Issue 3: Dependency Injection Not Working
Dependency injection is a core concept in the Spring Framework, and issues related to it can significantly impact the application's functionality.
Troubleshooting Steps:
-
Verify Bean Configuration: Double-check the bean configuration in the XML file or via Java Config. Ensure that the dependency injection is correctly defined.
-
Constructor Injection: If using constructor injection, ensure that the constructor is properly defined and annotated with
@Autowired
.☕snippet.java@Service public class UserService { private final UserRepository userRepository; @Autowired public UserService(UserRepository userRepository) { this.userRepository = userRepository; } }
-
Circular Dependencies: If there are circular dependencies, consider refactoring the code to eliminate the circular reference, or use setter injection instead.
By ensuring meticulous configuration and resolution, dependency injection issues in the Spring Framework can be effectively addressed.
For in-depth understanding, refer to the official Spring documentation on Dependency Injection and Constructor-based Dependency Injection.
My Closing Thoughts on the Matter
In conclusion, troubleshooting common Spring Framework issues requires thorough understanding and attention to detail. By following the troubleshooting steps outlined in this article, developers can effectively resolve issues related to bean autowiring, transaction management, and dependency injection in their Spring applications.
It is essential to leverage the extensive documentation and resources provided by Spring Framework to gain a deeper understanding of the concepts and best practices for troubleshooting.
By mastering the art of troubleshooting Spring Framework issues, developers can build robust and stable applications that leverage the power of the Spring ecosystem.
Remember, clear understanding and persistence are key in overcoming obstacles when working with the Spring Framework!
Happy coding!