Why Stream Data Instead of Keeping in Memory?
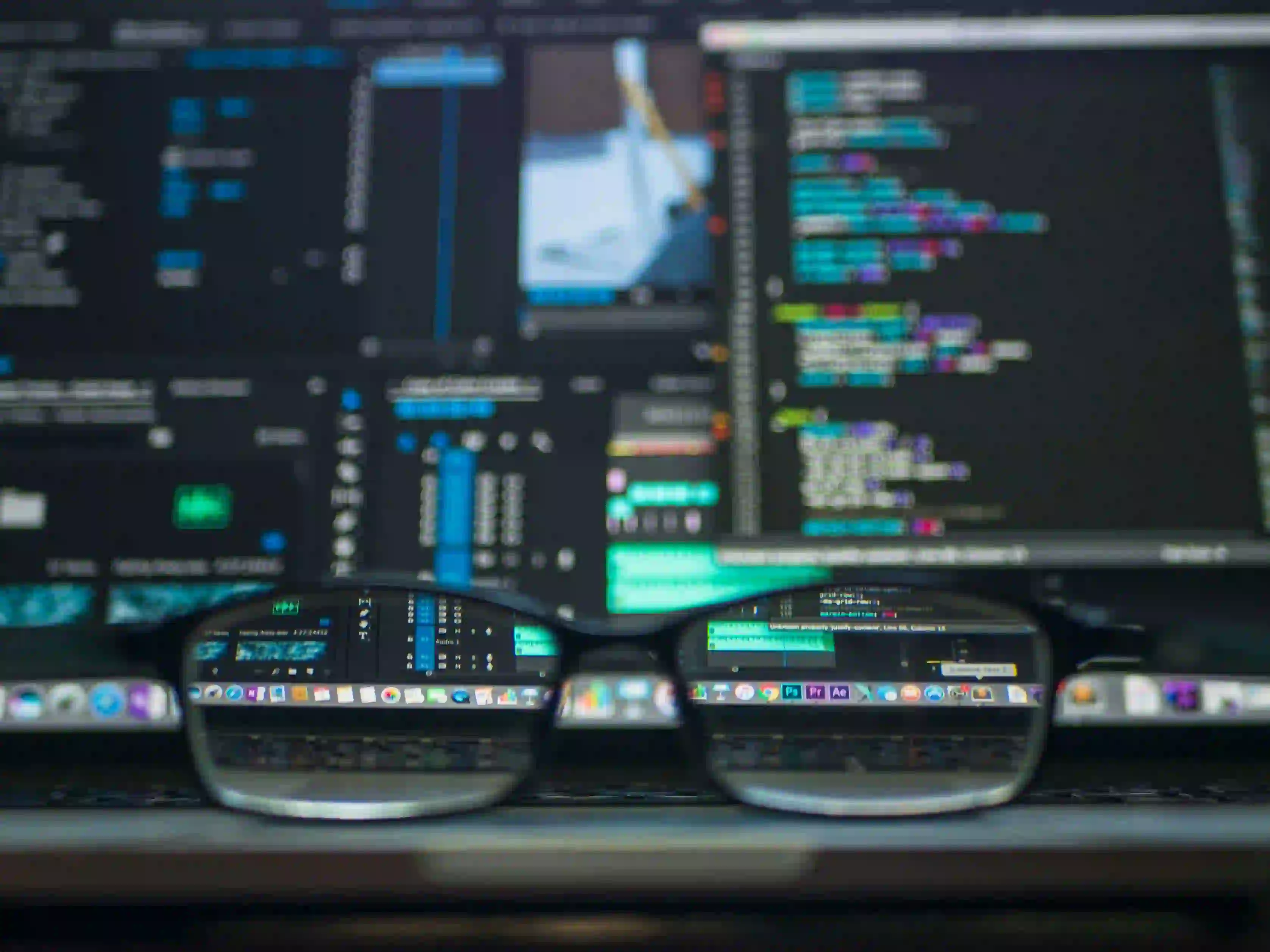
When it comes to handling data in Java, the choice between streaming data and keeping it in memory is crucial. This decision affects not only the performance and efficiency of the application but also its scalability and resource consumption. In this blog post, we'll delve into the reasons why streaming data is often preferred over keeping it in memory in Java applications.
Understanding Memory Usage
In Java, memory is managed by the Java Virtual Machine (JVM), and the amount of memory available to a Java process is limited. When data is kept in memory, it consumes valuable resources, which may lead to out-of-memory errors, especially when dealing with large datasets or when running multiple processes concurrently.
Data Size and Performance
Streaming data is beneficial when dealing with large datasets that cannot fit into memory. By processing data in a streaming fashion, you can avoid loading the entire dataset into memory at once, thus minimizing memory usage and improving the overall performance of the application. Streaming allows you to process data in smaller, more manageable chunks, making it well-suited for tasks such as reading from or writing to files, network communication, and database operations.
Let's consider an example where we need to process a large file containing millions of records. Instead of loading the entire file into memory, we can use Java's Stream
API to process the file line by line, reducing the memory footprint and enabling efficient processing of the data.
try (Stream<String> lines = Files.lines(Paths.get("large-file.txt"))) {
lines.forEach(this::processLine);
}
In this example, the Files.lines
method returns a stream of lines from the file, allowing us to process the data without loading the entire file into memory.
Efficiency and Responsiveness
Streaming data also enhances the efficiency and responsiveness of an application. By processing data as it becomes available, rather than waiting for the entire dataset to be loaded, applications can be more responsive and can start producing results sooner. This is particularly important in scenarios such as real-time data processing, where immediate action is required as new data arrives.
Resource Utilization
In addition to memory usage, streaming data also has implications for other system resources, such as I/O operations and network bandwidth. When data is streamed, it can be processed and transferred in a more efficient manner, reducing the strain on these resources.
I/O Operations
Streaming data is closely tied to I/O operations, such as reading from or writing to files, databases, or network sockets. By streaming data, these operations can be performed incrementally, thereby reducing the need for large buffers and minimizing disk or network contention. This results in improved I/O throughput and reduced latency.
Network Bandwidth
When transmitting data over a network, streaming can significantly impact the effective use of network bandwidth. By sending data in smaller, more frequent chunks, streaming minimizes the amount of idle time on the network and can lead to more efficient utilization of the available bandwidth. This is especially relevant in scenarios involving real-time data transfers, where timely delivery of data is critical.
Scalability and Flexibility
The use of streaming in Java applications also contributes to their scalability and flexibility. By adopting a streaming approach, applications can effectively handle a wide range of data sizes and types, adapt to varying resource constraints, and accommodate diverse processing requirements.
Handling Large Datasets
In the realm of big data and distributed systems, the ability to process and analyze large datasets is paramount. Streaming enables applications to work with datasets that exceed the available memory capacity, allowing them to scale seamlessly without being hindered by memory constraints. This is particularly advantageous in cloud computing environments, where resources are dynamically provisioned and may be limited.
Dynamic Processing Requirements
Another advantage of streaming data is its ability to adapt to dynamic processing requirements. As data arrives or evolves over time, streaming allows for on-the-fly processing and transformation, enabling applications to adjust to changing input characteristics without incurring the overhead of reloading or recalculating the entire dataset.
Concurrency and Parallelism
In Java, streaming data can be leveraged to introduce concurrency and parallelism into data processing tasks. By utilizing the parallel stream capabilities provided by the Stream
API, applications can exploit multi-core processors and distributed computing environments to enhance the performance and throughput of data processing operations.
Parallel Stream Processing
The Java Stream
API offers support for parallel processing of data through the use of parallel streams. When processing large datasets, parallel streams can distribute the workload across multiple threads, tapping into the computational power of modern multi-core processors. This can lead to significant performance improvements, especially when dealing with computationally intensive tasks or data-bound operations.
Consider the following example, where a list of numbers is processed in parallel to calculate their total sum:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int totalSum = numbers.parallelStream().mapToInt(Integer::intValue).sum();
By using parallelStream
instead of stream
, the processing of the numbers can be distributed across multiple threads, resulting in improved performance for large datasets.
To Wrap Things Up
In conclusion, streaming data in Java offers numerous benefits over keeping data in memory. From reducing memory consumption and improving performance to enhancing resource utilization, scalability, and parallel processing, streaming data aligns with the demands of modern applications, especially those dealing with large, dynamic, and real-time data. By embracing a streaming-oriented approach, Java applications can achieve greater efficiency, responsiveness, and adaptability in handling diverse data processing tasks.
Incorporating streaming data processing capabilities into your Java applications can lead to more robust, scalable, and performant solutions, making it an essential consideration for developers aiming to build modern, data-centric applications.
Stream away for better data handling in your Java applications!