Troubleshooting Custom Data Source Integration in Apache Spark
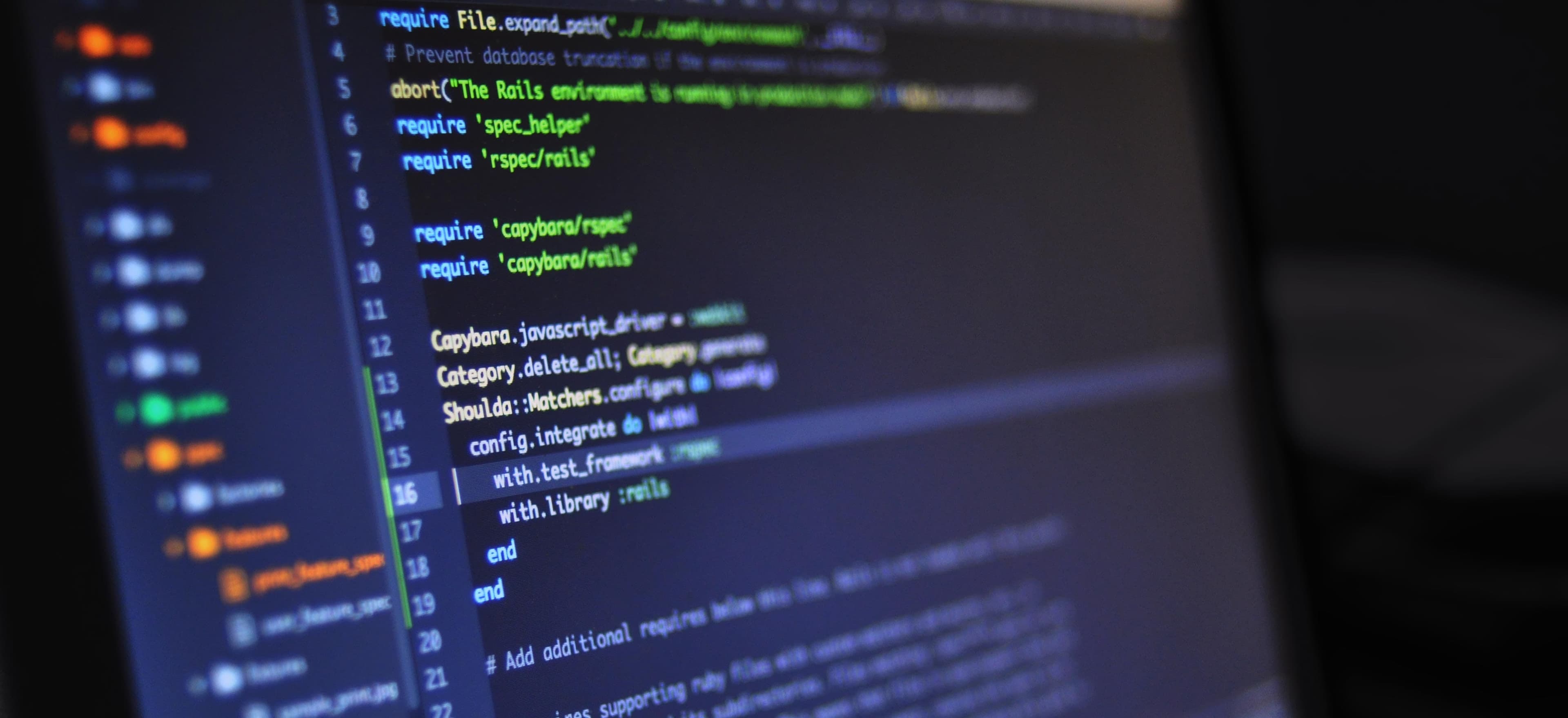
- Published on
Troubleshooting Custom Data Source Integration in Apache Spark
Apache Spark is a powerful open-source distributed computing system that provides an easy-to-use interface for processing a vast amount of data. One of the key features of Spark is its ability to work with various data sources. Although Spark comes with built-in support for many data formats and storage systems, there may be situations where you need to integrate Spark with a custom data source.
In this article, we will explore the process of integrating a custom data source with Apache Spark and discuss some common issues that may arise during this process. We will also provide troubleshooting tips and best practices to help you overcome these challenges.
Understanding Custom Data Source Integration
When integrating a custom data source with Apache Spark, you typically need to develop a custom data source API that conforms to Spark's data source API. This involves implementing the DataSourceRegister
interface and providing implementations for the BaseRelation
, PrunedFilteredScan
, and InsertableRelation
traits as needed.
Example of Custom Data Source Integration
Let's consider a hypothetical scenario where you need to integrate Spark with a custom data source that stores log data in a proprietary format. You would need to create a custom data source API that allows Spark to read and write data from this source efficiently.
// CustomDataSourceReader.scala
class CustomDataSourceReader extends DataSourceReader {
// Implement methods to support data reading from the custom source
}
// CustomDataSourceWriter.scala
class CustomDataSourceWriter extends DataSourceWriter {
// Implement methods to support data writing to the custom source
}
// CustomDataSourceProvider.scala
class CustomDataSourceProvider extends DataSourceRegister {
// Implement methods to register the custom data source provider
}
// CustomDataSourceRelation.scala
class CustomDataSourceRelation extends BaseRelation with PrunedFilteredScan with InsertableRelation {
// Implement methods to define the schema and scan/filter/writing operations
}
In this example, you would need to define the reader, writer, provider, and relation components that interface with your custom data source. This allows Spark to seamlessly interact with your proprietary log data format.
Common Issues and Troubleshooting Tips
Integrating a custom data source with Apache Spark can be challenging, and various issues may arise during the development and deployment stages. Let's explore some of the common issues and how to troubleshoot them effectively.
Issue 1: Schema Inference and Data Types
Problem: Spark may have difficulty inferring the schema and data types when reading data from a custom source, leading to incorrect data interpretation.
Troubleshooting Tip: Explicitly define the schema inference and data types by implementing the schema
method in your CustomDataSourceRelation
. Provide accurate metadata and data types to ensure Spark interprets the data correctly.
Issue 2: Data Pushdown and Predicate Pushdown
Problem: Limited or inefficient support for data pushdown and predicate pushdown can lead to poor query performance when working with a large dataset in a custom data source.
Troubleshooting Tip: Implement the buildScan
method in your CustomDataSourceRelation
to support predicate pushdown and data filtering at the data source level. This can significantly improve query performance by reducing the amount of data transferred to Spark for processing.
Issue 3: Partitioning and Parallelism
Problem: Inadequate partitioning and parallelism strategies can result in suboptimal data processing and resource utilization within Apache Spark.
Trouleshooting Tip: Implement partitioning and parallelism techniques within the CustomDataSourceRelation
to optimize data distribution and processing. Utilize Spark's partitioning mechanisms to parallelize data retrieval and processing tasks effectively.
Issue 4: Data Writing and Integrity
Problem: Writing data back to a custom data source while ensuring data integrity and consistency can be challenging, especially in distributed processing environments.
Trouleshooting Tip: Implement the necessary checks and mechanisms in your CustomDataSourceWriter
to ensure data integrity during write operations. Utilize transactional capabilities if the data source supports them to maintain consistency when writing data.
Issue 5: Error Handling and Logging
Problem: Inadequate error handling and logging practices can make it difficult to diagnose and troubleshoot issues when interacting with a custom data source.
Trouleshooting Tip: Implement comprehensive error handling and logging within your custom data source components to capture and report errors effectively. Use Spark's logging facilities to provide detailed information about data source interactions and potential issues.
Best Practices for Custom Data Source Integration
In addition to troubleshooting specific issues, it's essential to follow best practices when integrating a custom data source with Apache Spark. These best practices can help streamline the development process and ensure the robustness and performance of your custom data integration solution.
Best Practice 1: Test-Driven Development
Adopt a test-driven development (TDD) approach when implementing your custom data source integration. Write comprehensive unit tests to validate the functionality and behavior of your custom data source components. This helps identify and address issues early in the development lifecycle.
Best Practice 2: Performance Benchmarking
Conduct performance benchmarking and profiling to evaluate the efficiency of data retrieval, processing, and writing operations with your custom data source. Identify potential performance bottlenecks and optimize your integration based on empirical data and metrics.
Best Practice 3: Documentation and Examples
Provide thorough documentation and usage examples for developers who will interact with your custom data source integration. Clear documentation and practical examples can facilitate seamless adoption and troubleshooting for users integrating Spark with your custom data source.
Best Practice 4: Community Engagement
Engage with the Spark community and seek feedback on your custom data source integration. Participate in relevant forums, mailing lists, and community events to gather insights, share experiences, and collaborate with other developers working on similar integrations.
The Last Word
Integrating a custom data source with Apache Spark can unleash the full potential of Spark's data processing capabilities. While the process may pose challenges, understanding common issues, troubleshooting effectively, and following best practices can ensure a seamless and robust integration.
By implementing a custom data source API that conforms to Spark's data source API, addressing schema inference, pushdown strategies, partitioning, data integrity, error handling, and following best practices, you can overcome hurdles and enhance the compatibility and performance of Spark with your custom data source.
To delve deeper into custom data source integration and troubleshoot specific issues, refer to Spark's official documentation, community forums, and relevant resources. Embracing best practices and a proactive approach to troubleshooting can pave the way for a successful integration that leverages the full power of Apache Spark with your custom data source.
In conclusion, effective custom data source integration in Apache Spark demands persistence, attention to detail, and a deep understanding of Spark's capabilities and the intricacies of the custom data source. Through rigorous troubleshooting, adherence to best practices, and engagement with the Spark community, you can elevate your integration to deliver exceptional performance and functionality within the context of Apache Spark.
Remember to stay proactive, keep learning, and leverage the wealth of available resources to conquer any challenges in integrating custom data sources with Apache Spark.
Start integrating your custom data sources with Apache Spark today, and embark on a journey of unparalleled data processing and analytics prowess!
Checkout our other articles