Handling XML Parsing in Android: Best Practices
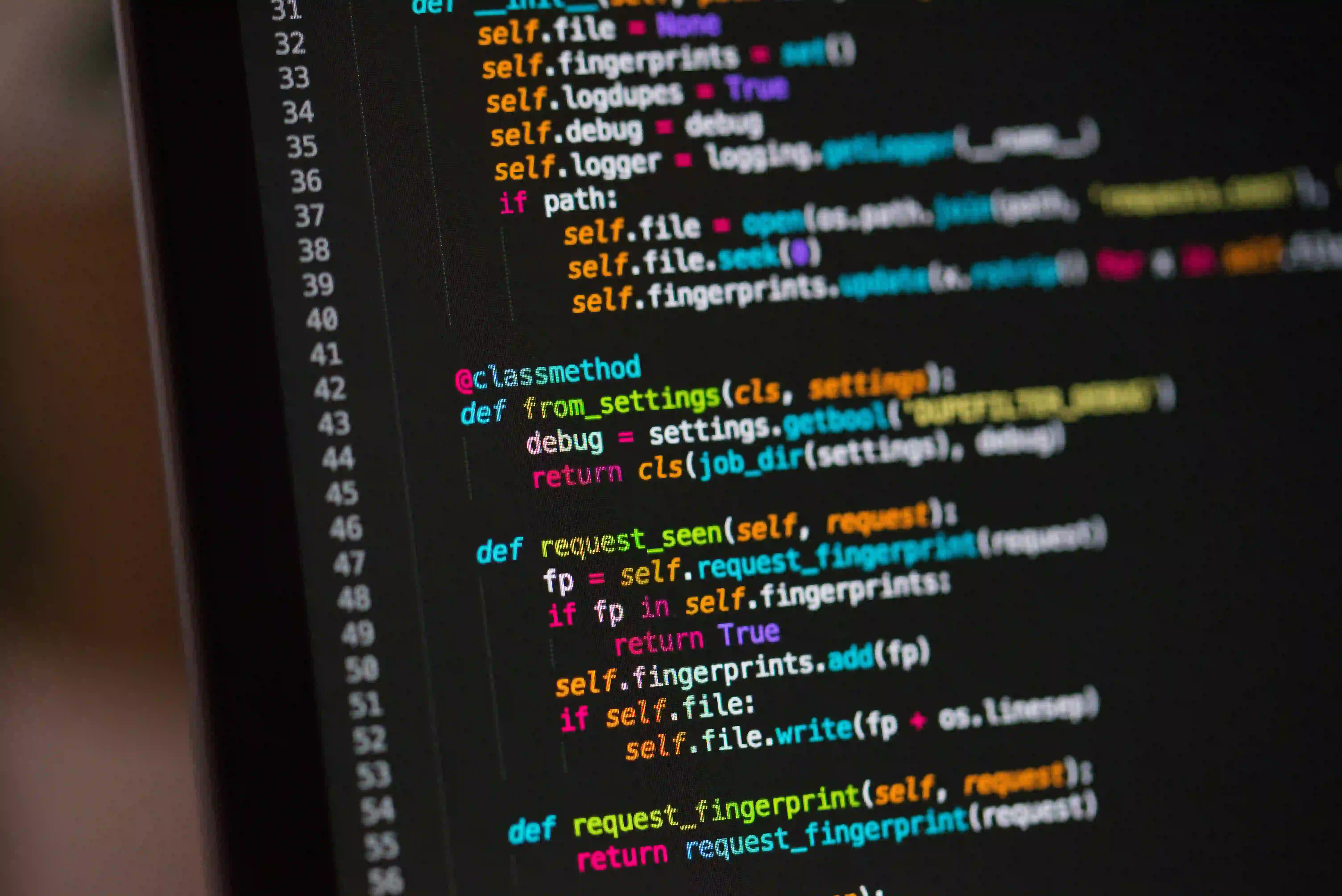
How to Handle XML Parsing in Android: Best Practices
XML parsing is an essential aspect of Android development, especially when dealing with web services or data exchange between different platforms. In this blog post, we'll explore the best practices for XML parsing in Android to ensure efficient, reliable, and maintainable code. We'll cover the various parsing methods, libraries, and tips for handling XML data effectively in your Android applications.
1. Understanding XML Parsing
XML (Extensible Markup Language) is a popular data format used for storing and transporting data. In Android development, XML parsing involves extracting data from XML documents and converting it into a usable format within the application. This process is crucial for integrating web services, consuming APIs, or reading configuration files.
2. Using Android's Built-in XmlPullParser
Android provides the XmlPullParser interface, which is a fast and efficient way to parse XML data. XmlPullParser allows for sequential parsing of XML documents, making it suitable for large XML files or streams. Here's an example of using XmlPullParser to parse XML data:
try {
XmlPullParserFactory factory = XmlPullParserFactory.newInstance();
XmlPullParser parser = factory.newPullParser();
parser.setInput(new StringReader(xmlData)); // xmlData is the XML content as a string
int eventType = parser.getEventType();
while (eventType != XmlPullParser.END_DOCUMENT) {
if (eventType == XmlPullParser.START_TAG) {
String tagName = parser.getName();
// Process the XML data based on the tag
}
eventType = parser.next();
}
} catch (XmlPullParserException | IOException e) {
e.printStackTrace();
}
In this code snippet, we create an XmlPullParser instance, set its input to the XML content, and iterate through the XML events to process the data accordingly. XmlPullParser is a low-level parsing method that provides fine-grained control over the parsing process.
3. Leveraging Third-Party Libraries
While XmlPullParser is a robust parsing option, using third-party libraries can simplify XML parsing and provide additional features. One such popular library is Simple XML, which offers annotation-based XML parsing and serialization. Here's an example of using Simple XML to parse XML data in Android:
@Root(name = "book")
public class Book {
@Element(name = "title")
private String title;
@Element(name = "author")
private String author;
// Getters and setters
}
// Parsing XML using Simple XML
Serializer serializer = new Persister();
try {
Book book = serializer.read(Book.class, xmlData); // xmlData is the XML content as a string
// Process the parsed book object
} catch (Exception e) {
e.printStackTrace();
}
Simple XML allows you to map XML elements to Java objects using annotations, reducing the manual parsing code and providing a more intuitive way of working with XML data.
Another popular library is DOM (Document Object Model), which represents the XML document as a tree structure in memory, allowing for easy traversal and manipulation of XML elements. However, DOM parsing can be memory-intensive and may not be suitable for large XML files.
4. Error Handling and Performance Considerations
When handling XML parsing in Android, it's crucial to implement robust error handling to deal with malformed or unexpected XML data. Additionally, consider the performance implications of XML parsing, especially when dealing with large XML files or frequent parsing operations. Here are some best practices to keep in mind:
- Use asynchronous parsing for large XML files to prevent blocking the main UI thread.
- Implement proper error handling using try-catch blocks to handle XML parsing exceptions.
- Profile the parsing performance using tools like Android Profiler to identify any performance bottlenecks.
5. Data Caching and Optimization
To optimize XML parsing in Android, consider implementing data caching mechanisms to store parsed XML data and reduce the need for frequent parsing. You can use techniques like SharedPreferences or SQLite databases to cache and retrieve parsed XML data efficiently. This approach helps improve the overall performance and responsiveness of your Android application, especially when dealing with static or semi-static XML data.
In Conclusion, Here is What Matters
In conclusion, XML parsing is a fundamental aspect of Android development, enabling seamless integration with web services and data exchange. Whether you choose to use Android's built-in XmlPullParser, third-party libraries like Simple XML, or DOM parsing, it's essential to consider error handling, performance optimization, and data caching for efficient XML parsing in Android applications.
By following best practices and leveraging appropriate parsing methods, you can ensure your Android app handles XML data effectively, providing a robust and responsive user experience.
For further insights into XML parsing in Android, check out the Android Developer documentation on Parsing XML Data and Providing Data to Other Apps. These resources offer additional guidance and best practices for handling XML parsing in Android applications.
Start implementing these best practices in XML parsing to enhance the performance and reliability of your Android applications today!