Maximizing Java Performance with Efficient Memory Management
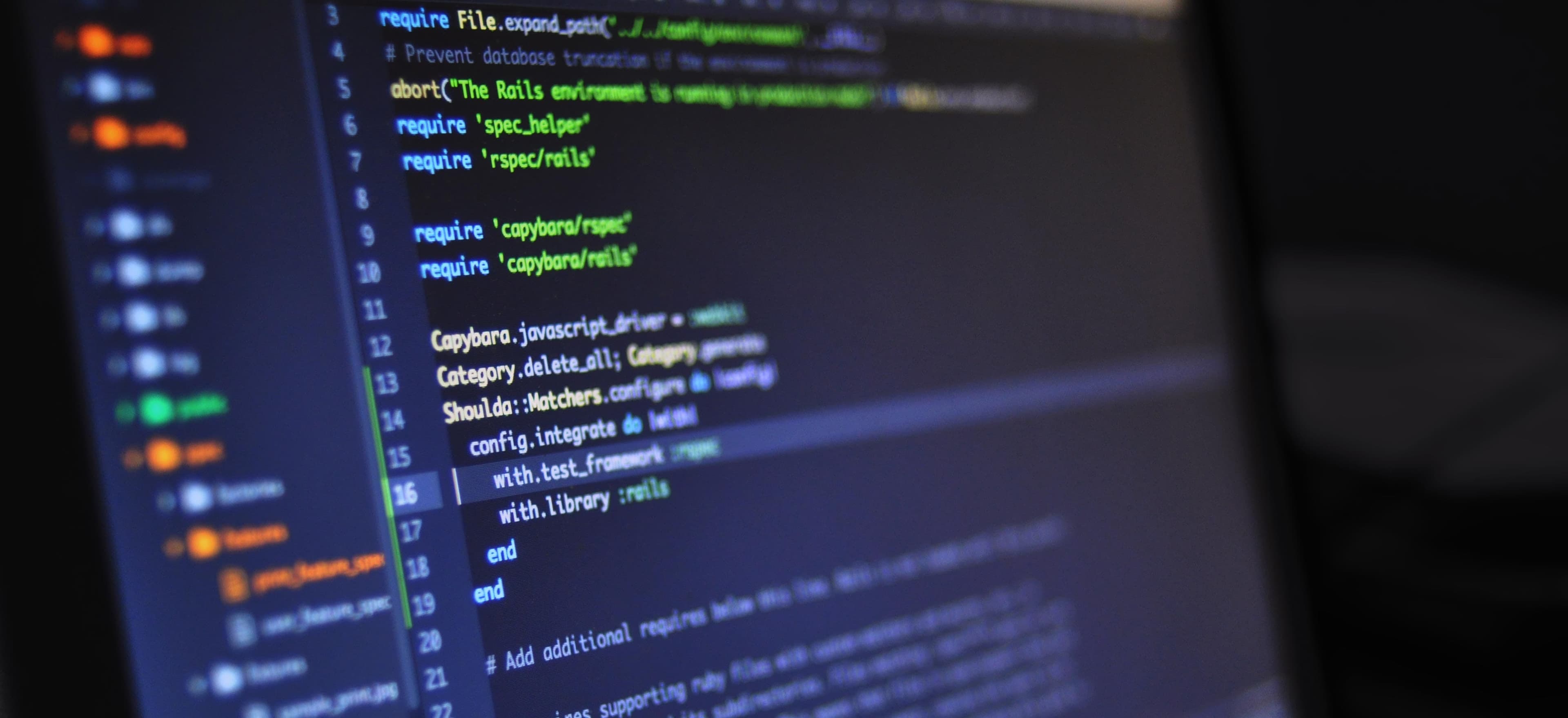
- Published on
Maximizing Java Performance with Efficient Memory Management
When it comes to Java programming, efficient memory management can significantly impact the performance of your application. In this article, we will explore various techniques to maximize Java performance through effective memory management.
Understanding Java Memory Management
Java uses automatic memory management through a process called garbage collection. The Java Virtual Machine (JVM) automatically allocates and deallocates memory, allowing developers to focus on writing code without worrying about memory leaks or manual memory management.
Garbage Collection in Java
Garbage collection is the process of automatically freeing up memory by deallocating objects that are no longer referenced by the program. Java employs several garbage collection algorithms, such as the Serial Garbage Collector, Parallel Garbage Collector, Concurrent Mark Sweep (CMS) Garbage Collector, and G1 Garbage Collector. Each algorithm has its own characteristics, and the selection of the appropriate garbage collector depends on the specific requirements of the application.
Java also provides a way to explicitly request garbage collection using System.gc()
. However, it's important to note that this is just a hint to the JVM, and the actual garbage collection process is still decided by the JVM.
Tips for Efficient Memory Management in Java
1. Use Primitive Data Types Instead of Objects
When possible, use primitive data types such as int
, float
, double
, and boolean
instead of their corresponding wrapper classes (Integer
, Float
, Double
, Boolean
). Primitive data types consume less memory and incur less overhead compared to their object counterparts.
// Object version
Integer number = new Integer(10);
// Primitive version
int count = 10;
By using primitive data types, you can reduce memory consumption and improve performance.
2. Minimize Object Creation
Frequent object creation leads to increased pressure on the garbage collector. When designing your classes, consider reusing objects instead of creating new ones. For example, you can utilize object pooling to manage a pool of reusable objects.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.setLength(0); // Reset the StringBuilder instead of creating a new one
// Perform operations using the StringBuilder
}
By reusing objects, you can reduce memory allocation overhead and improve the overall performance of your application.
3. Use Effective Data Structures
Choosing the right data structure can have a significant impact on memory usage and performance. For example, using ArrayList
may be more efficient than LinkedList
in certain scenarios due to differences in memory allocation and access patterns.
List<String> names = new ArrayList<>(); // Prefer ArrayList when frequent access by index is required
Understanding the performance characteristics of different data structures can help you make informed decisions about memory management in your Java applications.
4. Optimize String Handling
Strings are immutable objects in Java, which means every operation that appears to modify a string actually creates a new string instance. This can lead to unnecessary memory allocation and increased overhead.
String message = "Hello, " + name + "!"; // This results in additional string objects being created
To optimize string handling, consider using StringBuilder
for mutable string operations and being mindful of excessive string concatenations.
5. Monitor Memory Usage and Performance
Utilize tools like JVisualVM, Java Mission Control, or YourKit to monitor memory usage, garbage collection behavior, and overall performance of your Java applications. These tools provide valuable insights into memory allocation, object lifecycles, and potential performance bottlenecks.
Monitoring memory usage and performance allows you to identify areas for improvement and optimize memory management strategies in your Java applications.
Wrapping Up
Efficient memory management is integral to maximizing the performance of Java applications. By understanding garbage collection, minimizing object creation, using effective data structures, optimizing string handling, and monitoring memory usage, developers can significantly improve the memory efficiency and overall performance of their Java applications.
Implementing these memory management techniques can lead to more responsive and scalable Java applications, providing a better user experience and reducing operational costs in resource-constrained environments.
Remember, optimizing memory management is an ongoing process, and continuous monitoring and refinement are essential for achieving optimal performance in Java applications.
Optimize your Java applications today by implementing efficient memory management techniques and achieving peak performance!
References
- Java Garbage Collection Basics
- Understanding Java Garbage Collection
- Java Memory Management Best Practices
- Effective Java Memory Management
- Java Performance Tuning Tips
By following these Java memory management best practices, you can ensure that your applications run smoothly and efficiently. Do you have any other tips for optimizing Java performance? Let's discuss in the comments!