Handling Timezone Conversion in Custom JSR-303 Bean Validation Constraints
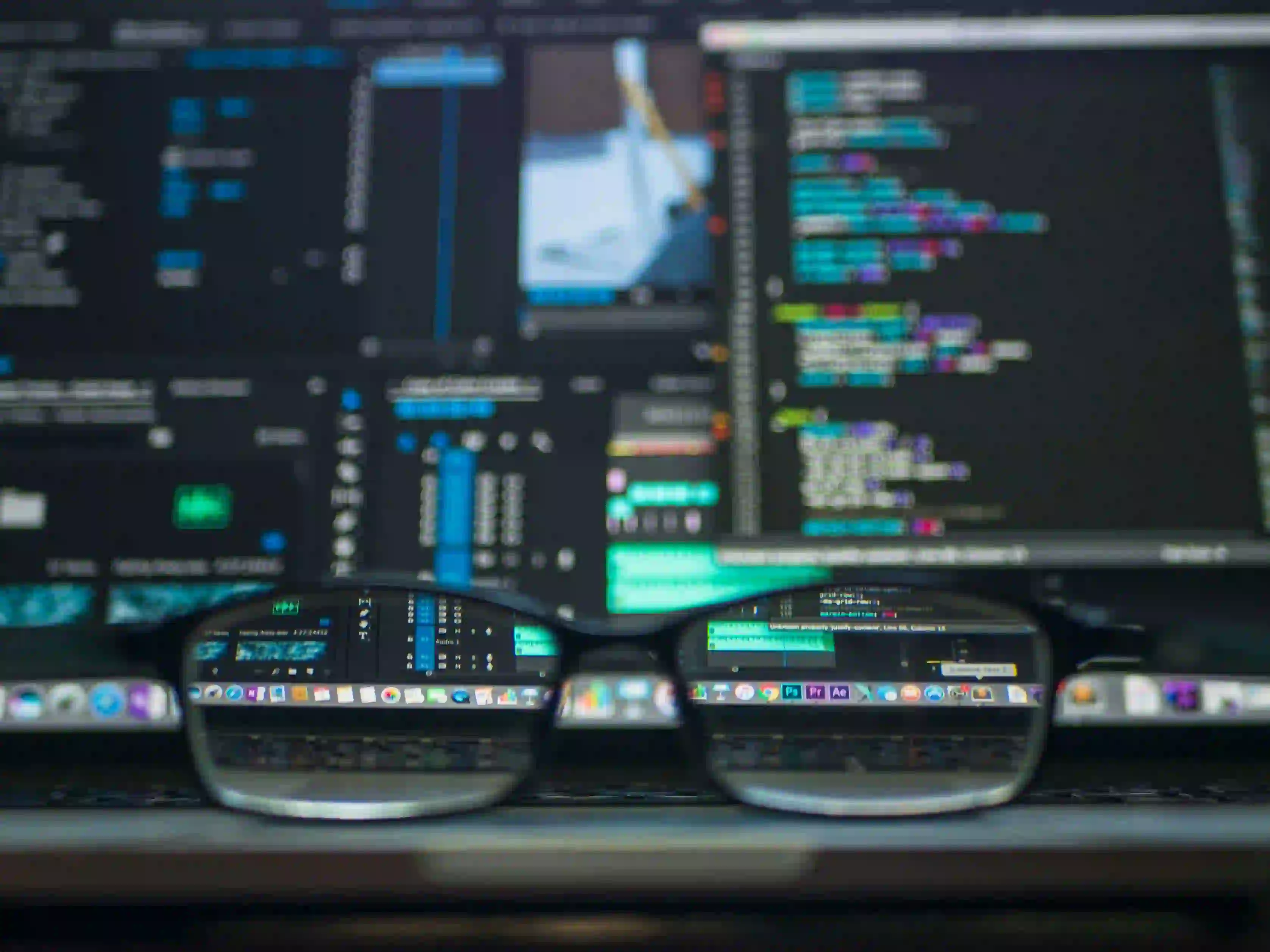
Handling Timezone Conversion in Custom JSR-303 Bean Validation Constraints
When working with date and time in Java applications, it's important to consider timezone conversions, especially when dealing with user input and validation. In this blog post, we'll discuss how to handle timezone conversion in custom JSR-303 bean validation constraints. We'll explore the challenges involved and provide practical examples to demonstrate the implementation.
Understanding Timezone Conversions in Bean Validation
Before diving into the implementation, it's essential to understand the significance of timezone conversions in bean validation. When users input dates and times in a web application, they may be in different timezones. This presents a challenge when validating these inputs against predefined constraints, as the validation should consider the timezone and ensure that the input meets the specified criteria regardless of the timezone from which it originated.
Custom JSR-303 Constraints for Timezone-Aware Validation
To address the timezone conversion in bean validation, we can create custom constraints by leveraging the JSR-303 validation framework. This allows us to define validation rules that incorporate timezone adjustments. Let's consider an example of validating a future date input while accounting for the user's timezone.
Creating the Custom Constraint Annotation
First, we define a custom constraint annotation @FutureInTimeZone
to mark the target date field for validation. We'll specify the timezone ID as a parameter for the constraint to indicate the timezone in which the validation should occur.
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Constraint(validatedBy = FutureInTimeZoneValidator.class)
public @interface FutureInTimeZone {
String message() default "must be a future date in the specified timezone";
String timezone() default "UTC";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
In this annotation, @FutureInTimeZone
defines the custom constraint and specifies the default timezone as UTC. This allows us to set the timezone when applying the constraint to a field.
Implementing the Custom Constraint Validator
Next, we implement the custom constraint validator FutureInTimeZoneValidator
to enforce the validation logic with timezone conversion. In the validator, we convert the input date to the specified timezone and compare it with the current time to determine if it's a future date.
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
import java.time.*;
import java.time.temporal.ChronoUnit;
public class FutureInTimeZoneValidator implements ConstraintValidator<FutureInTimeZone, ZonedDateTime> {
private String timezone;
@Override
public void initialize(FutureInTimeZone constraintAnnotation) {
this.timezone = constraintAnnotation.timezone();
}
@Override
public boolean isValid(ZonedDateTime value, ConstraintValidatorContext context) {
LocalDateTime convertedDateTime = value.withZoneSameInstant(ZoneId.of(timezone)).toLocalDateTime();
LocalDateTime currentDateTime = LocalDateTime.now(ZoneOffset.UTC);
return convertedDateTime.isAfter(currentDateTime);
}
}
In this validator, we use the java.time
API to perform the conversion of the input date to the specified timezone and compare it with the current time. This ensures that the validation takes into account the timezone specified by the @FutureInTimeZone
constraint.
Applying the Custom Constraint in Java Entities
Once the custom constraint is defined, we can apply it to Java entity fields for timezone-aware validation. Here's an example of using the @FutureInTimeZone
constraint in an entity class.
public class Event {
@FutureInTimeZone(timezone = "America/New_York")
private ZonedDateTime eventDateTime;
// Other fields and methods
}
In this example, the @FutureInTimeZone
constraint is applied to the eventDateTime
field of the Event
class, specifying the timezone as America/New_York
. When an instance of Event
is validated, the eventDateTime
field will be validated against the future date constraint in the specified timezone.
Final Considerations
Handling timezone conversion in custom JSR-303 bean validation constraints is crucial for ensuring accurate and consistent validation of date and time inputs in Java applications. By creating custom constraints and validators, we can address the challenges posed by timezone variations and facilitate proper validation irrespective of the user's timezone.
In this blog post, we've discussed the importance of timezone conversions in bean validation, demonstrated the creation of custom constraints and validators for timezone-aware validation, and illustrated the application of these constraints in Java entities. By following these guidelines, you can effectively manage timezone-related validation scenarios in your Java applications.
For further information on JSR-303 bean validation and the java.time
API, refer to the following resources:
By integrating timezone-aware validation into your applications, you can enhance the robustness and accuracy of date and time inputs, providing a seamless user experience across different timezones.