Mastering Apache Camel: Building Applications Efficiently
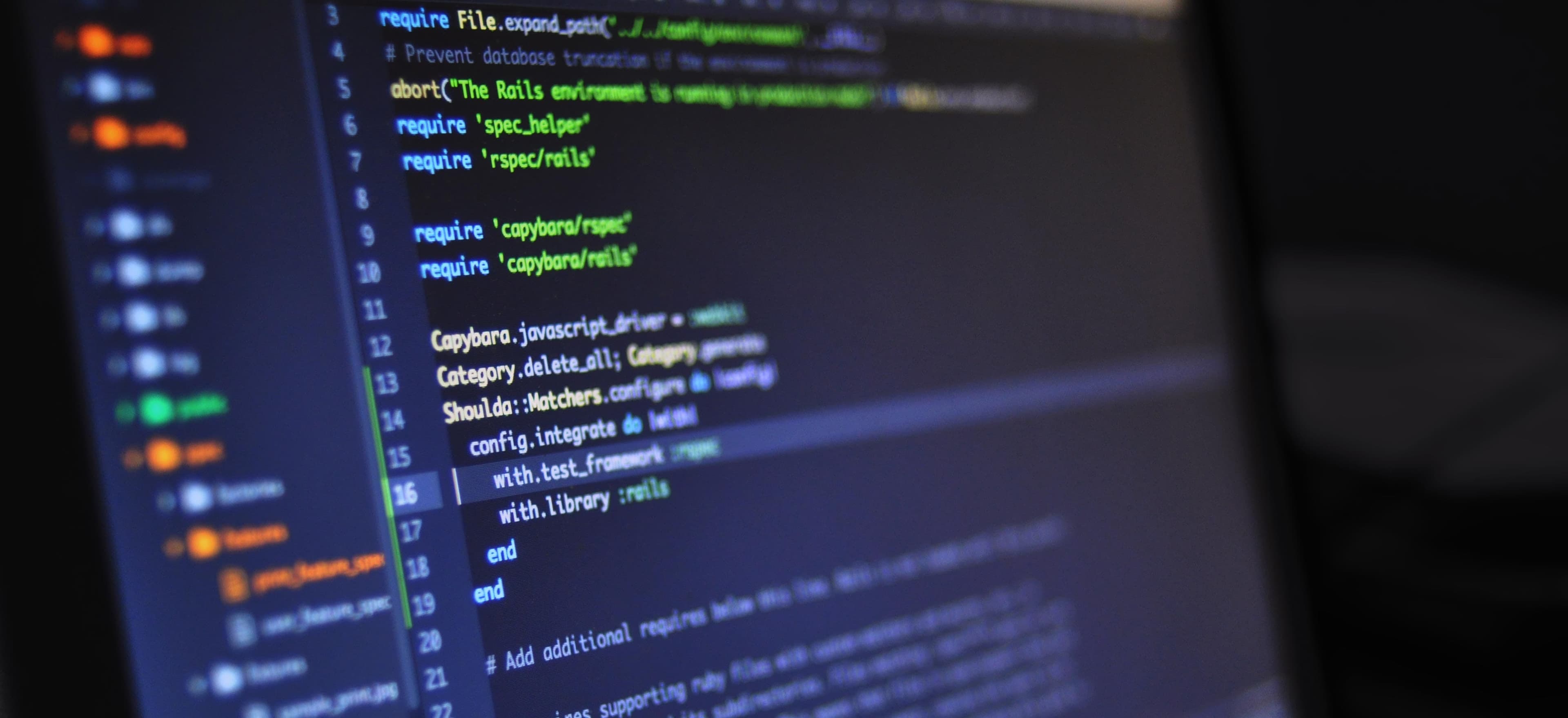
- Published on
Mastering Apache Camel: Building Applications Efficiently
Apache Camel is a powerful open-source integration framework that allows you to integrate a variety of systems using a simple and lightweight manner. In this blog post, we'll explore the key concepts of Apache Camel and how you can use it to build efficient applications.
What is Apache Camel?
Apache Camel is an open-source Java framework that focuses on making integration easier by providing a clean and consistent DSL for routing and mediating messages between different systems. It allows you to easily implement enterprise integration patterns and supports a wide range of transport protocols and data formats.
Getting Started with Apache Camel
To get started with Apache Camel, you'll first need to set up a project with the required dependencies. You can use tools like Maven or Gradle to manage your project dependencies. Here's an example of a simple Maven pom.xml
file that includes the Camel core dependency:
<dependencies>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>3.14.0</version>
</dependency>
</dependencies>
Once you have your project set up, you can start writing Camel routes. A Camel route is a sequence of processing steps that define how messages should be routed and processed. Routes are defined using the Camel DSL, which provides a fluent and expressive way to describe integration logic.
Here's an example of a simple Camel route that consumes messages from a JMS queue and logs them:
import org.apache.camel.builder.RouteBuilder;
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
from("jms:queue:myQueue")
.log("Received message: ${body}");
}
}
In this example, from("jms:queue:myQueue")
specifies that the route should consume messages from the myQueue
JMS queue, and .log("Received message: ${body}")
logs the message body.
Enterprise Integration Patterns
Apache Camel is based on the concept of enterprise integration patterns (EIP), which are a set of design patterns used to describe common integration problems. Camel provides implementations for many of these patterns, making it easier to solve complex integration challenges.
For example, the Content-Based Router pattern allows you to route messages based on their content. Here's an example of how you can use the Content-Based Router pattern in Camel:
from("direct:input")
.choice()
.when(header("type").isEqualTo("important"))
.to("jms:queue:importantMessages")
.when(header("type").isEqualTo("lowPriority"))
.to("jms:queue:lowPriorityMessages")
.otherwise()
.to("jms:queue:defaultQueue");
In this example, messages are routed to different JMS queues based on their type
header.
Testing Apache Camel Routes
Testing Camel routes is an important part of building robust integration applications. Camel provides a testing framework that allows you to easily write unit tests for your routes. You can use tools like JUnit to write tests for your Camel routes.
Here's an example of a simple unit test for the previous Camel route:
import org.apache.camel.builder.AdviceWithRouteBuilder;
import org.apache.camel.test.blueprint.CamelBlueprintTestSupport;
import org.junit.Test;
public class MyRouteBuilderTest extends CamelBlueprintTestSupport {
@Test
public void testRoute() throws Exception {
context.getRouteDefinition("myRoute")
.adviceWith(context, new AdviceWithRouteBuilder() {
@Override
public void configure() throws Exception {
replaceFromWith("direct:input");
}
});
template.sendBodyAndHeader("direct:input", "Test message", "type", "important");
assertMockEndpointsSatisfied();
}
}
In this example, we're using Camel's AdviceWithRouteBuilder
to modify the route for testing purposes. We then send a test message using the Camel ProducerTemplate
and assert that the message is routed correctly.
Monitoring and Management
Apache Camel provides support for monitoring and management through JMX. You can monitor route performance, gather statistics, and manage Camel contexts using JMX tools such as JConsole or VisualVM.
To enable JMX in Camel, you can use the ManagementStrategy
configuration. Here's an example of how you can enable JMX in a Camel context:
CamelContext context = new DefaultCamelContext();
context.getManagementStrategy().addEventNotifier(new JmxNotificationEventNotifier());
context.getManagementStrategy().setManagementNamePattern("MyCamelContext");
context.start();
With JMX enabled, you can then use JConsole or other JMX tools to monitor and manage your Camel routes.
Bringing It All Together
Apache Camel is a powerful integration framework that allows you to build efficient and reliable integration applications. By leveraging its DSL, enterprise integration patterns, testing framework, and JMX support, you can build robust applications that seamlessly integrate different systems.
With its extensive documentation and active community, mastering Apache Camel can greatly enhance your ability to build and maintain complex integration solutions.
So, are you ready to dive into the world of Apache Camel and supercharge your integration projects?
Start your journey by exploring the official Apache Camel documentation and getting involved in the Apache Camel community. Happy integrating!
Checkout our other articles