Customizing Exit Codes in Spring Boot: A Guide
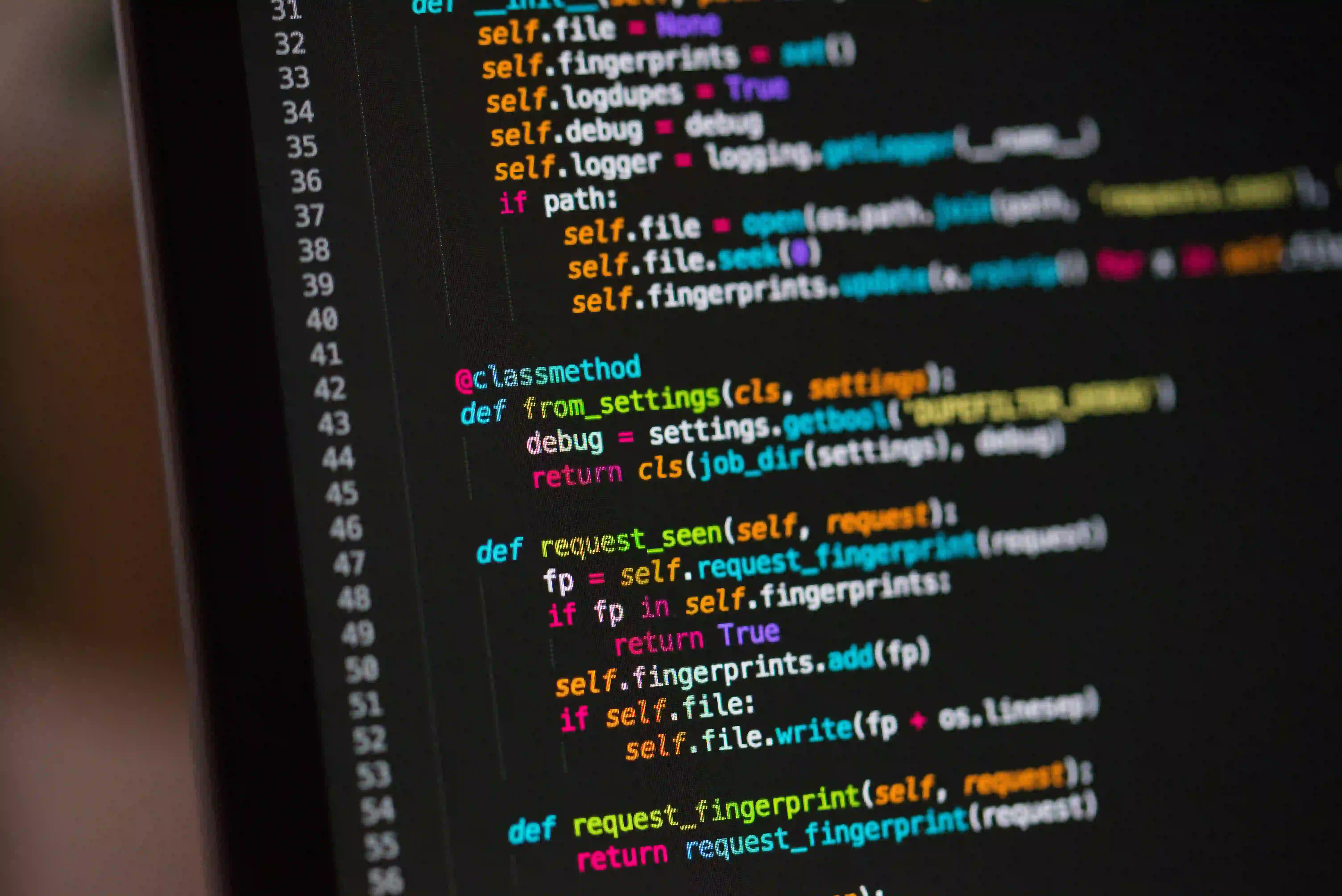
Customizing Exit Codes in Spring Boot: A Guide
In a Spring Boot application, using exit codes can be a valuable tool for conveying the status of the application's termination to external processes. While Spring Boot provides default exit codes, customizing them can offer a more nuanced understanding of why the application terminated and can aid in troubleshooting issues.
What are Exit Codes?
Exit codes are numeric values returned by a program to the calling process, representing the termination status of the program. In the context of a Spring Boot application, exit codes are essential for indicating the reason for its shutdown.
Default Exit Codes in Spring Boot
Spring Boot assigns default exit codes for different scenarios, such as successful termination, startup failure, and command line parsing errors. For instance, the exit code 0 is returned when the application exits successfully, while non-zero codes indicate specific types of errors.
Customizing Exit Codes in Spring Boot
Customizing exit codes in Spring Boot involves creating a custom ExitCodeGenerator
bean and using it to provide bespoke exit codes that align with the application's termination scenarios.
Creating a Custom ExitCodeGenerator
To create a custom exit code generator in Spring Boot, simply implement the ExitCodeGenerator
interface and override the getExitCode()
method. Here's an example:
import org.springframework.boot.ExitCodeGenerator;
import org.springframework.stereotype.Component;
@Component
public class CustomExitCodeGenerator implements ExitCodeGenerator {
@Override
public int getExitCode() {
// Custom logic to determine the exit code
return 42;
}
}
In this example, the CustomExitCodeGenerator
implements the ExitCodeGenerator
interface and provides a custom exit code of 42.
Using the Custom Exit Code
To trigger the custom exit code, invoke the SpringApplication.exit()
method and pass the Spring context, along with the custom exit code as arguments.
SpringApplication.exit(context, new CustomExitCodeGenerator());
By calling this method, the application will terminate with the custom exit code provided by the CustomExitCodeGenerator
.
Why Customize Exit Codes?
Customizing exit codes in Spring Boot can be beneficial for several reasons:
-
Clarity: Custom exit codes can convey specific information about the reason for the application's termination, aiding in troubleshooting and diagnostics.
-
Integration: They enable better integration with external processes or scripts that rely on exit codes to determine the next course of action.
-
Granularity: By using custom exit codes, developers can define a broader range of termination scenarios, allowing for more detailed feedback on the application's state.
A Final Look
In summary, customizing exit codes in Spring Boot offers developers a way to provide more nuanced feedback on the application's termination. By creating a custom ExitCodeGenerator
and using it to define bespoke exit codes, developers can enhance the clarity, integration, and granularity of their application's termination status.
For more information on exit codes in Spring Boot, refer to the official Spring Boot documentation.
We hope this guide has been helpful in understanding the importance of customizing exit codes in Spring Boot applications. Happy coding!