Optimizing Query Performance in JPA Entities
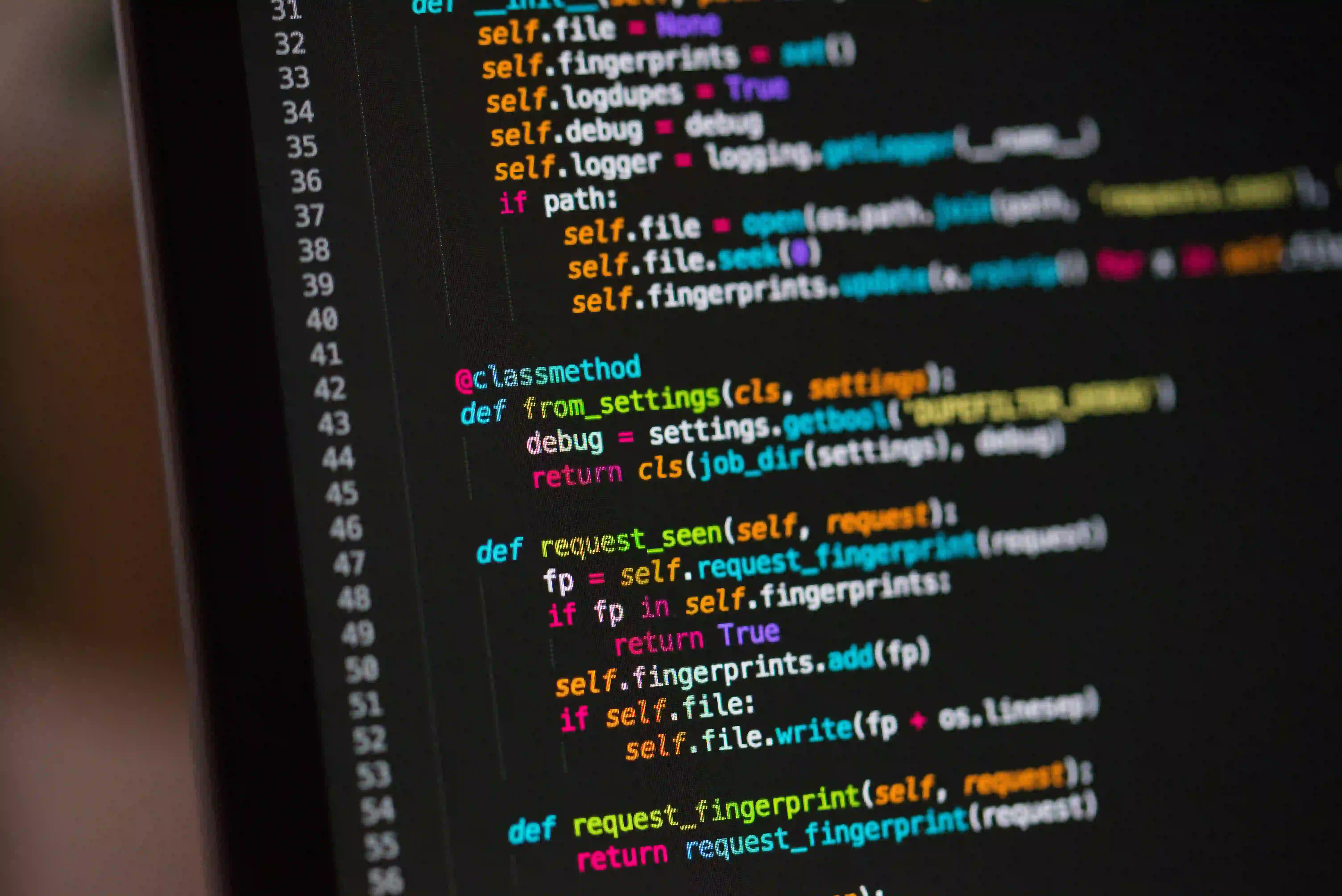
Optimizing Query Performance in JPA Entities
When working with Java Persistence API (JPA) and dealing with large datasets, optimizing query performance is crucial for maintaining the efficiency and responsiveness of your application. In this article, we will explore various strategies and best practices for optimizing query performance in JPA entities.
1. Choose the Right Fetching Strategy
In JPA, fetching strategy determines how and when related entities are retrieved from the database. The two main fetching strategies are EAGER
and LAZY
.
-
EAGER fetching: When an entity is retrieved, all its related entities are also retrieved immediately. This can lead to performance issues, especially when dealing with large datasets.
-
LAZY fetching: Related entities are only retrieved when explicitly accessed in the code. This is the preferred strategy for optimizing performance, as it avoids unnecessary data retrieval.
Example
@Entity
public class Order {
// other fields
@OneToMany(mappedBy = "order", fetch = FetchType.LAZY)
private List<OrderItem> orderItems;
// getters and setters
}
In this example, the orderItems
collection is configured with a LAZY
fetching strategy, ensuring that it is only retrieved when accessed in the code.
2. Use Entity Graphs for Fetching
Entity graphs allow you to specify a graph of entity relationships that should be retrieved from the database in a single query, reducing the number of database calls and improving performance.
@Entity
@NamedEntityGraph(name = "order-items-graph",
attributeNodes = @NamedAttributeNode("orderItems"))
public class Order {
// other fields
@OneToMany(mappedBy = "order")
private List<OrderItem> orderItems;
// getters and setters
}
The @NamedEntityGraph
annotation defines an entity graph named "order-items-graph", which specifies that when an Order
entity is retrieved, its orderItems
should also be fetched.
3. Use Batch Fetching for Collections
Batch fetching is a technique where related entities are retrieved in batches, reducing the number of database calls and improving performance.
@OneToMany(mappedBy = "order")
@BatchSize(size = 25)
private List<OrderItem> orderItems;
In this example, the @BatchSize
annotation specifies that the orderItems
collection should be fetched in batches of 25, reducing the number of database calls when accessing the collection.
4. Utilize Second-Level Caching
Second-level caching allows entities and their relationships to be cached at the session factory level, reducing the need for repeated database calls.
@Entity
@Cacheable
public class Product {
// entity fields and methods
}
By adding the @Cacheable
annotation to the entity class, you can enable second-level caching for the entity, improving performance by reducing database access.
5. Avoid N+1 Query Issues
The N+1 query issue occurs when fetching related entities in a loop, resulting in multiple database calls. This can severely impact performance, especially with large datasets.
List<Order> orders = entityManager.createQuery("SELECT o FROM Order o", Order.class).getResultList();
for (Order order : orders) {
// N+1 query issue
System.out.println(order.getOrderItems().size());
}
To avoid the N+1 query issue, you can use the JOIN FETCH
clause in JPQL to retrieve the related entities in a single query.
List<Order> orders = entityManager.createQuery("SELECT DISTINCT o FROM Order o JOIN FETCH o.orderItems", Order.class).getResultList();
By using JOIN FETCH
, you can eagerly fetch the orderItems
collection in a single query, avoiding the N+1 query problem and improving performance.
Lessons Learned
Optimizing query performance in JPA entities is essential for maintaining the efficiency and responsiveness of your application, especially when dealing with large datasets. By carefully choosing fetching strategies, using entity graphs and batch fetching, utilizing second-level caching, and avoiding N+1 query issues, you can significantly improve the performance of your JPA-based application.
By following these best practices and leveraging the features provided by JPA, you can ensure that your application performs optimally even with large and complex datasets.
Remember, embracing these performance optimization strategies will not only enhance the user experience but also make your application more scalable and easier to maintain in the long run.
To learn more about optimizing the performance of JPA entities, check out the official Hibernate documentation and Baeldung's JPA performance tuning guide.
Now, go forth and optimize your JPA entities for peak performance!