Configuring Couchbase deployment parameters using REST API
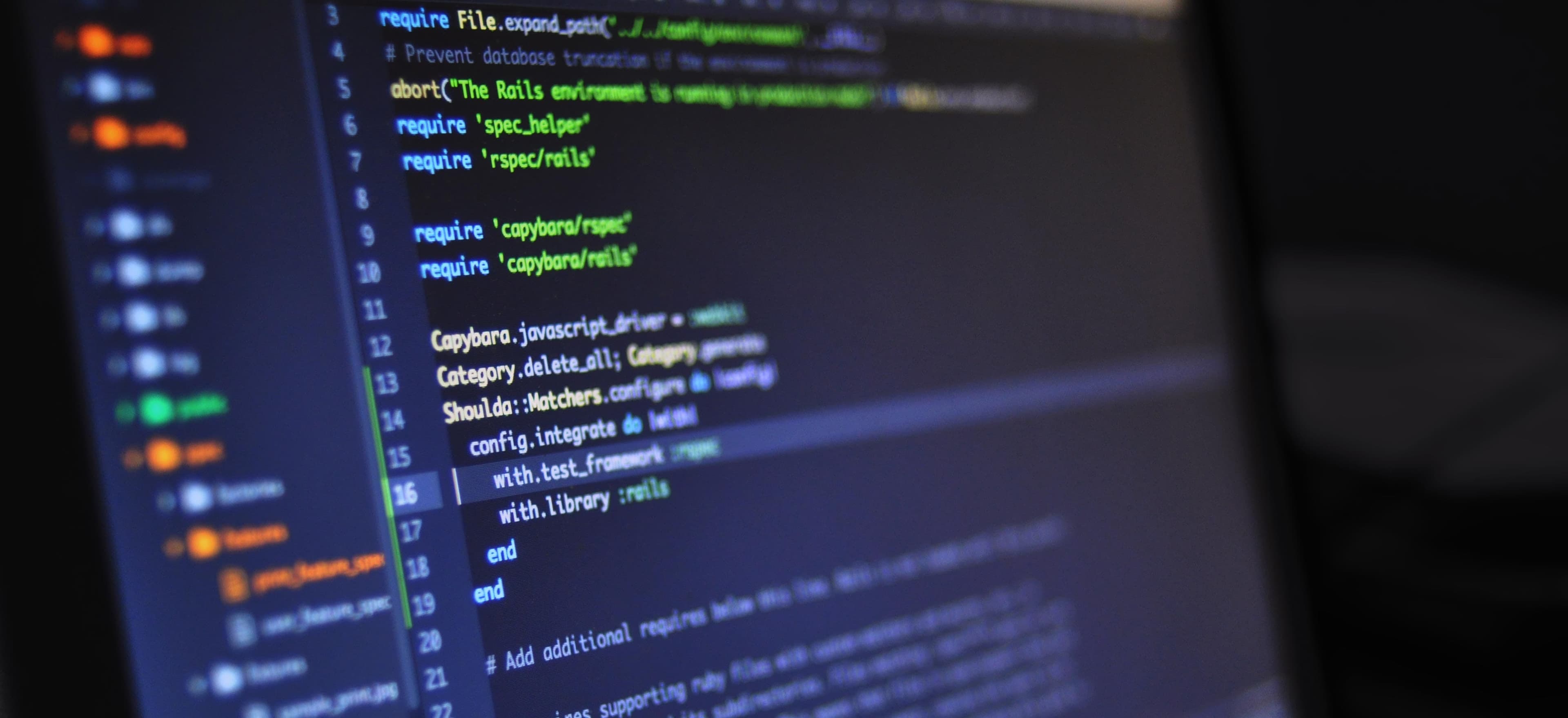
- Published on
Optimizing Your Couchbase Deployment with Java and REST API
Couchbase, a popular NoSQL database, is designed to manage large amounts of unstructured data. To configure and fine-tune your Couchbase deployment, you can harness the power of the REST API. In this post, we will delve into how to utilize Java to interact with the Couchbase REST API, optimizing your deployment to achieve superior performance.
Preparing the Environment
Before we jump into coding, ensure you have the following setup:
- Couchbase Server: Make sure you have a Couchbase Server instance up and running.
- Java Development Kit (JDK): Install the latest JDK to compile and run your Java code.
- Maven or Gradle: Choose your preferred build tool for managing dependencies and building the project.
Managing Deployment Parameters with REST API
Creating a Cluster
To start, let's see how to create a cluster using the Couchbase REST API. We'll use Java to send HTTP requests to the Couchbase Server.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class CouchbaseCluster {
public static void main(String[] args) {
try {
URL url = new URL("http://your-couchbase-instance:8091/pools/default");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.connect();
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the example above, we send a POST request to create a cluster by connecting to http://your-couchbase-instance:8091/pools/default
. Upon successful execution, you will receive a response containing the cluster details.
Fine-Tuning Parameters
Next, let's focus on fine-tuning various parameters of the Couchbase deployment using the REST API. One crucial parameter is the RAM quota allocation for data storage.
public class CouchbaseRAMQuota {
public static void main(String[] args) {
try {
URL url = new URL("http://your-couchbase-instance:8091/pools/default");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
conn.setDoOutput(true);
String data = "memoryQuota=300"; // Set the memory quota to 300 MB
conn.getOutputStream().write(data.getBytes("UTF-8"));
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, we adjust the RAM quota by sending a POST request with the desired memory quota specified in the data
variable.
Creating Buckets
Additionally, we can utilize the REST API to create buckets with specific configurations.
public class CouchbaseBucket {
public static void main(String[] args) {
try {
URL url = new URL("http://your-couchbase-instance:8091/pools/default/buckets");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
conn.setRequestProperty("Authorization", "Basic " + Base64.getEncoder().encodeToString("username:password".getBytes()));
conn.setDoOutput(true);
String data = "name=myBucket&ramQuotaMB=100&proxyPort=11211"; // Customize bucket parameters
conn.getOutputStream().write(data.getBytes("UTF-8"));
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
By executing the code above, you can create a new bucket with specific settings such as name, RAM quota, and proxy port.
The Closing Argument
By leveraging the Couchbase REST API with Java, you can seamlessly manage and fine-tune deployment parameters to optimize performance and resource utilization. Remember to handle exceptions properly and ensure secure communication by incorporating authentication where necessary.
Now, armed with the knowledge of utilizing the Couchbase REST API in Java, you are equipped to tailor your Couchbase deployment to meet your specific requirements, ensuring a well-optimized and efficient database environment.
Continue to explore the extensive capabilities of Couchbase and empower your applications with a robust and finely-tuned data management solution.
Happy coding!