Building a Robust Enterprise Java Application: Tackling Performance Bottlenecks
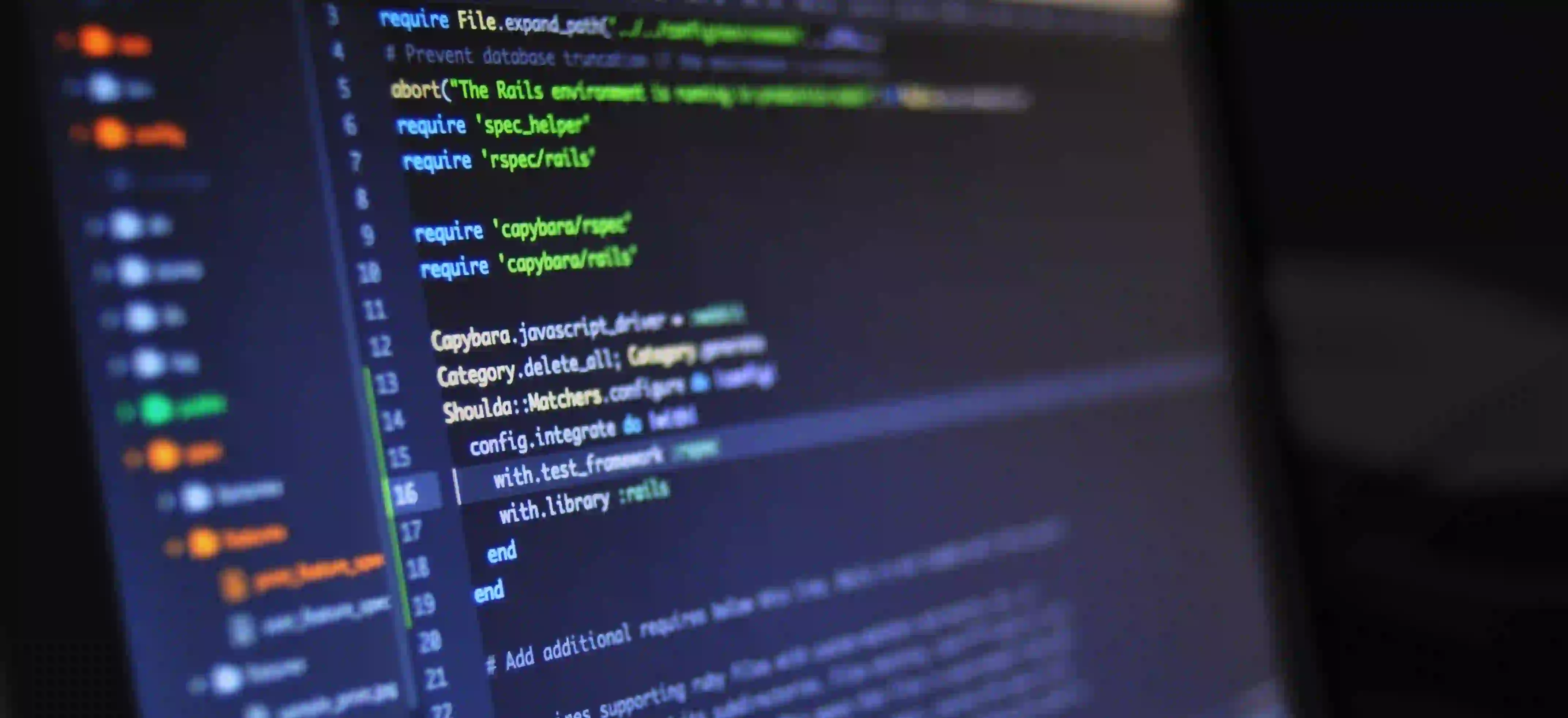
Building a Robust Enterprise Java Application: Tackling Performance Bottlenecks
In the world of enterprise Java applications, performance bottlenecks can be a nightmare. As usage scales, a well-designed application that was once fast and efficient can become sluggish and unresponsive. In this blog post, we'll explore some common performance bottlenecks in Java applications and discuss strategies for identifying and addressing them.
Understanding Performance Bottlenecks
Performance bottlenecks can manifest in various forms, such as slow response times, high memory consumption, or excessive CPU utilization. Identifying the root cause of these bottlenecks is crucial for optimizing the application's performance.
Common Performance Bottlenecks in Java Applications
-
Inefficient Algorithms and Data Structures: Using inefficient algorithms or inappropriate data structures can significantly impact performance.
-
Memory Leaks: Improper memory management can lead to memory leaks, causing the application to consume excessive memory.
-
Excessive Garbage Collection: Frequent garbage collection cycles can introduce significant pauses, affecting the application's responsiveness.
-
Database Query Performance: Suboptimal database queries can result in slow data retrieval and processing.
-
Concurrency Issues: Inadequate management of concurrent access to shared resources can lead to contention and performance degradation.
Profiling the Application
Before addressing performance issues, it's essential to profile the application to identify the specific areas that require optimization.
Using Java Profiling Tools
Tools such as Java Mission Control and VisualVM provide insights into the application's performance, including CPU and memory usage, thread activity, and garbage collection behavior.
Let's take a look at a basic Java code snippet and discuss how profiling can help identify performance bottlenecks.
public class ProfilingExample {
public static void main(String[] args) {
// Simulate a computationally intensive task
for (int i = 0; i < 1000000; i++) {
// Perform some operations
}
}
}
By using a profiling tool to analyze this code, we can determine the execution time, CPU usage, and any potential bottleneck caused by the intensive task.
Strategies for Tackling Performance Bottlenecks
1. Optimizing Algorithms and Data Structures
Choosing the right algorithms and data structures is crucial for efficient application performance. For instance, using a HashMap for fast key-based retrieval or employing efficient sorting algorithms can significantly improve overall performance.
2. Memory Management and Garbage Collection
Proper memory management, including minimizing object creation and avoiding unnecessary object retention, can mitigate memory-related performance issues. Additionally, tuning garbage collection parameters based on application behavior can reduce pauses caused by excessive garbage collection.
3. Database Query Optimization
Identifying and optimizing inefficient database queries, such as by adding appropriate indexes or restructuring the queries, can significantly enhance data retrieval performance.
4. Concurrency Control
Effective concurrency management, using tools like synchronized blocks, locks, or concurrent data structures, can mitigate contention and improve the application's responsiveness under concurrent access scenarios.
5. Caching Strategies
Introducing caching mechanisms, such as using in-memory caches (e.g., Redis or Memcached) or adopting application-level caching, can reduce the load on backend systems and improve overall responsiveness.
Example: Improving Database Query Performance
Let's consider a scenario where a Java application experiences slow database query performance. By using a profiling tool, we've identified a particularly inefficient SQL query that retrieves large amounts of data. To address this bottleneck, we can employ the following strategies:
Rewriting the Query
String inefficientQuery = "SELECT * FROM huge_table WHERE condition = true";
Optimize the query to retrieve only the necessary columns and employ proper indexing to enhance retrieval efficiency.
Using JPA Criteria API
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Entity> query = cb.createQuery(Entity.class);
Root<Entity> root = query.from(Entity.class);
query.select(root).where(cb.equal(root.get("condition"), true));
Utilize JPA Criteria API to construct optimized queries, benefiting from type safety and better query construction.
By addressing database query performance using these strategies, the application's overall responsiveness and data retrieval efficiency can be significantly improved.
Closing the Chapter
In the world of enterprise Java applications, tackling performance bottlenecks is a critical aspect of ensuring optimal application responsiveness. By understanding common performance issues, leveraging profiling tools for identification, and employing targeted optimization strategies, developers can build robust and efficient Java applications capable of handling increasing usage and scaling demands.
Remember, optimizing performance is an ongoing process, and continuously monitoring and fine-tuning the application is essential for maintaining peak performance as the application evolves and grows.
As you tackle performance bottlenecks in your enterprise Java applications, keep these strategies in mind to ensure that your applications remain fast, efficient, and responsive in the face of increasing demands.
Happy coding!