How to Efficiently Test Expected Exceptions in JUnit
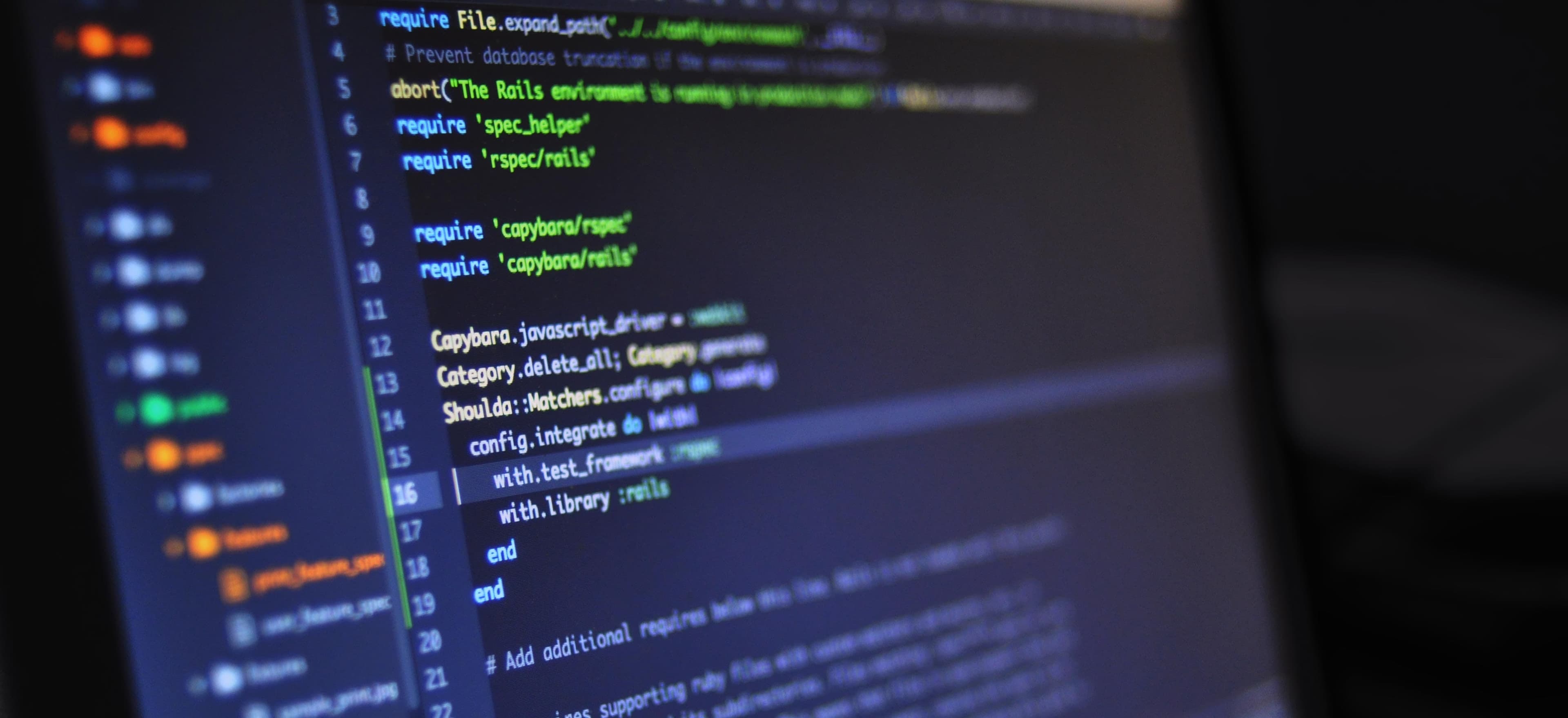
- Published on
How to Efficiently Test Expected Exceptions in JUnit
In Java development, testing plays a crucial role in ensuring the reliability and stability of our applications. When it comes to handling exceptions, it's vital to verify that the correct exceptions are thrown under specific circumstances. This is where JUnit, a popular testing framework, comes into play. In this article, we'll explore how to efficiently test expected exceptions in JUnit.
The Importance of Testing Expected Exceptions
Exceptions are a fundamental part of Java programming, used to handle errors and unexpected scenarios. When writing unit tests, it's essential to verify that the code throws the expected exceptions under certain conditions. Testing expected exceptions provides confidence in the robustness of our code and ensures that it behaves as intended in exceptional cases.
Traditional Approach to Testing Expected Exceptions
In traditional JUnit testing, handling expected exceptions involved writing verbose try-catch blocks within test methods. While this approach is functional, it often leads to code duplication and makes the test cases less readable and maintainable.
@Test
public void testDivideByZero() {
try {
// Code that is expected to throw an ArithmeticException
int result = 5 / 0;
// Fail if the exception is not thrown
fail("Expected ArithmeticException was not thrown");
} catch (ArithmeticException e) {
// Expected exception
}
}
Improved Approach Using AssertJ and AssertThrows
To streamline the process of testing expected exceptions, we can leverage AssertJ, a fluent assertion library, and JUnit 5's assertThrows
method.
Using AssertJ
AssertJ provides a concise and readable way to perform assertions. By using AssertJ's Assertions.assertThatThrownBy
method, we can effectively test expected exceptions without the need for a try-catch block.
@Test
public void testDivideByZero() {
assertThatThrownBy(() -> {
// Code that is expected to throw an ArithmeticException
int result = 5 / 0;
}).isInstanceOf(ArithmeticException.class);
}
In this example, assertThatThrownBy
captures the exception thrown by the specified code block, and isInstanceOf
verifies that the caught exception is of the expected type.
Using JUnit 5's assertThrows
JUnit 5 introduced the assertThrows
method, which further simplifies the testing of expected exceptions. This method takes the expected exception type and a Executable
(a functional interface) that represents the code expected to throw the exception.
@Test
public void testDivideByZero() {
Executable executable = () -> {
// Code that is expected to throw an ArithmeticException
int result = 5 / 0;
};
assertThrows(ArithmeticException.class, executable);
}
By using assertThrows
, we can achieve the same result in a more straightforward and elegant manner, enhancing the readability and maintainability of our test cases.
Parameterized Tests with Expected Exceptions
In certain scenarios, we may need to write parameterized tests where different inputs result in the same type of exception being thrown. JUnit 5 provides the @ParameterizedTest
annotation along with @MethodSource
to achieve this.
@ParameterizedTest
@MethodSource("divisionParameters")
public void testDivideByZero(int dividend, int divisor) {
Executable executable = () -> {
// Code that is expected to throw an ArithmeticException
int result = dividend / divisor;
};
assertThrows(ArithmeticException.class, executable);
}
static Stream<Arguments> divisionParameters() {
return Stream.of(
Arguments.of(10, 0),
Arguments.of(20, 0),
Arguments.of(15, 0)
);
}
In this example, the testDivideByZero
method is annotated with @ParameterizedTest
to indicate that it should be executed multiple times with different sets of arguments. The divisionParameters
method provides the different input combinations, and the assertThrows
method validates the expected exception for each set of inputs.
Final Considerations
Testing expected exceptions is a critical aspect of writing robust and reliable Java applications. By adopting AssertJ and JUnit 5's assertThrows
, we can streamline the process of testing expected exceptions, leading to more readable, maintainable, and efficient test cases. Additionally, leveraging parameterized tests further enhances our ability to test different scenarios that result in the same type of exception being thrown, adding to the overall robustness of our test suite.
Ensuring comprehensive test coverage, including expected exceptions, is essential for building software that meets high standards of quality and reliability.
To further enhance your understanding of testing in Java, take a look at this article on Best Practices for Testing in Java. Additionally, for in-depth knowledge of parameterized tests, check out the official JUnit 5 documentation.
By incorporating these techniques and best practices into your testing strategy, you can bolster the quality and stability of your Java applications, ultimately leading to more satisfied users and stakeholders.