Using Swagger for Java EE 7 REST Services
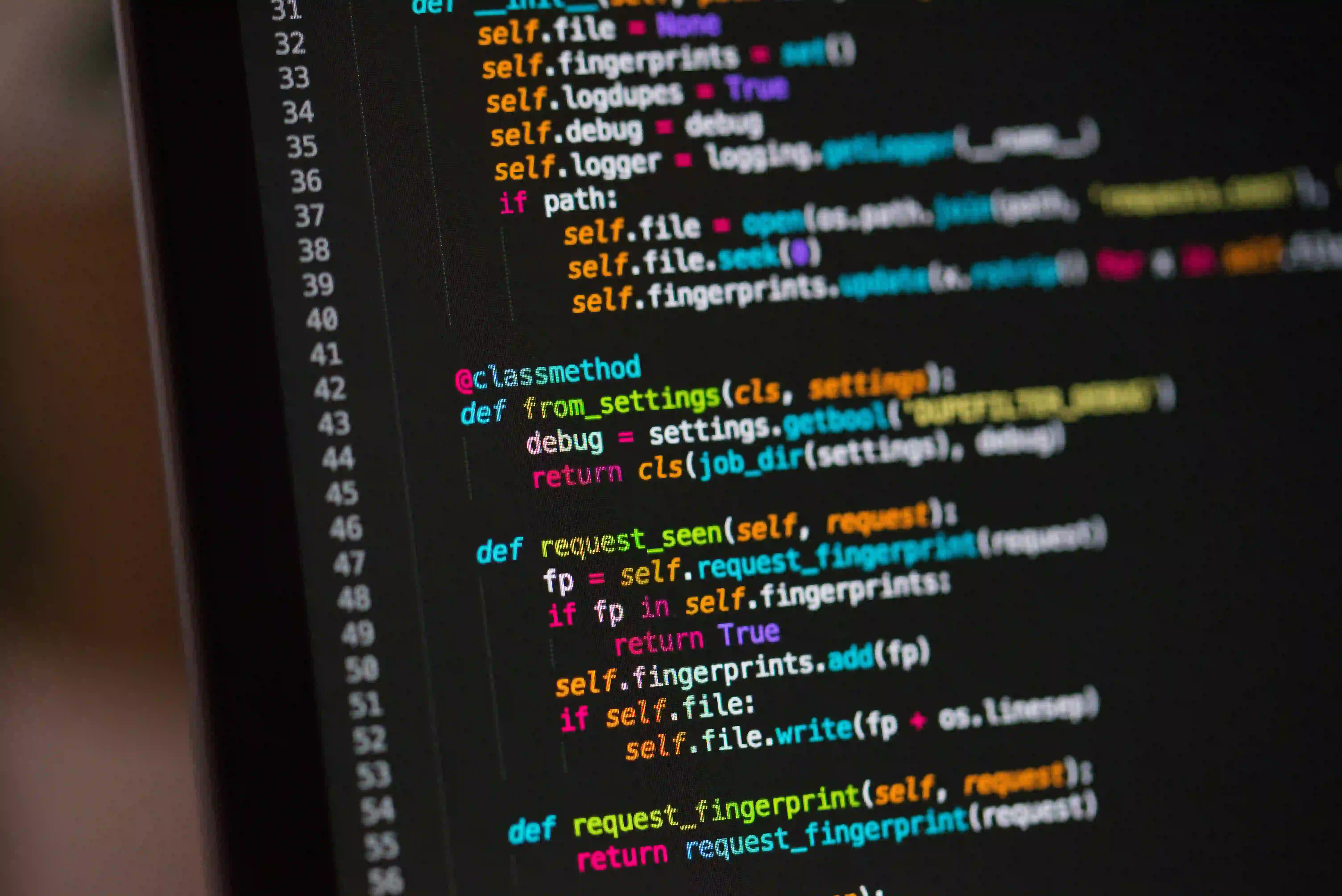
Demystifying Swagger for Java EE 7 REST Services
In today's rapidly evolving world of software development, creating efficient and well-documented RESTful services is crucial. Swagger, now known as the OpenAPI Specification, has emerged as a powerful tool for documenting and defining RESTful APIs. In this article, we will explore how to integrate Swagger with Java EE 7 to streamline the process of building and documenting RESTful services.
What is Swagger?
Swagger is a powerful open-source framework backed by a large ecosystem of tools, that can help you design, build, document, and consume your RESTful APIs. With Swagger, you can define your API's structure and operations using a common language, allowing both humans and computers to understand the capabilities of a service without access to source code or documentation.
Integrating Swagger with Java EE 7
Prerequisites
Before we begin, make sure you have Java EE 7 installed, as well as Maven for dependency management. You will also need a Java IDE, such as IntelliJ IDEA or Eclipse, to follow along with the examples.
Setting Up the Maven Project
Let's start by setting up a new Maven project for our Java EE 7 REST services. In your pom.xml
file, add the following dependencies:
<dependencies>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-jaxrs</artifactId>
<version>1.5.20</version>
</dependency>
</dependencies>
The javaee-api
dependency provides the necessary Java EE 7 APIs, while the swagger-jaxrs
dependency includes Swagger's JAX-RS integration, which allows us to automatically generate API documentation.
Creating a REST Service
Now, let's create a simple RESTful service using JAX-RS. We'll focus on a basic "Hello World" endpoint for demonstration purposes:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Path("/hello")
public class HelloService {
@GET
public Response getHello() {
return Response.ok("Hello, World!").build();
}
}
Here, we've created a HelloService
class with a single GET
method mapped to the /hello
path, which returns a "Hello, World!" message.
Integrating Swagger
To integrate Swagger with our REST service, we need to configure it within our application. We can do this by creating a JAX-RS Application subclass and annotating it with @ApplicationPath
and @SwaggerDefinition
:
import io.swagger.jaxrs.config.BeanConfig;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class JaxrsApplication extends Application {
public JaxrsApplication() {
BeanConfig beanConfig = new BeanConfig();
beanConfig.setVersion("1.0.0");
beanConfig.setSchemes(new String[]{"http"});
beanConfig.setHost("localhost:8080");
beanConfig.setBasePath("/yourapp/api");
beanConfig.setResourcePackage("your.package.name");
beanConfig.setScan(true);
}
}
In this example, we've created a JAX-RS Application subclass JaxrsApplication
and specified the base path for our API. We've also configured the Swagger BeanConfig to specify details about our API, such as the version, schemes, host, and resource package.
Accessing Swagger UI
With Swagger now integrated into our Java EE 7 project, we can access the automatically generated API documentation by navigating to the following URL in a web browser:
http://localhost:8080/yourapp/api/swagger.json
This URL provides access to the Swagger JSON file, which represents the entire Swagger definition of our RESTful API. Additionally, the Swagger UI can be accessed by visiting:
http://localhost:8080/yourapp/api/swagger-ui
The Swagger UI provides an interactive interface for exploring and testing the endpoints of our API.
Why Use Swagger with Java EE 7?
Integrating Swagger with Java EE 7 offers several benefits:
-
Automated Documentation: With Swagger, our API documentation is automatically generated based on the code, reducing the need for manually maintaining documentation.
-
Interactive API UI: The Swagger UI provides an interactive interface for developers to explore and test the endpoints of the API, simplifying the process of understanding and consuming the API.
-
Standardized API Specification: By using the OpenAPI Specification, we adhere to a widely-adopted standard for defining RESTful APIs, enhancing interoperability and collaboration with other teams and systems.
Lessons Learned
In this article, we've explored the integration of Swagger with Java EE 7 to streamline the process of building and documenting RESTful services. By following the steps outlined in this article, you can enhance the documentation and accessibility of your RESTful APIs, improving the developer experience and fostering collaboration across teams. With Swagger, the days of cumbersome and outdated API documentation are behind us, as we usher in a new era of well-documented, standardized, and interactive RESTful services.
Remember, adopting Swagger is not just about documentation - it's about empowering developers and consumers with clear, interactive, and standardized API specifications. So, why wait? Integrate Swagger with your Java EE 7 project today and elevate your RESTful APIs to new heights.
Start enhancing your Java EE 7 REST services with Swagger and unlock a world of well-documented, user-friendly APIs. Happy coding!