Streamlining Data Processing with Java 8 Collectors
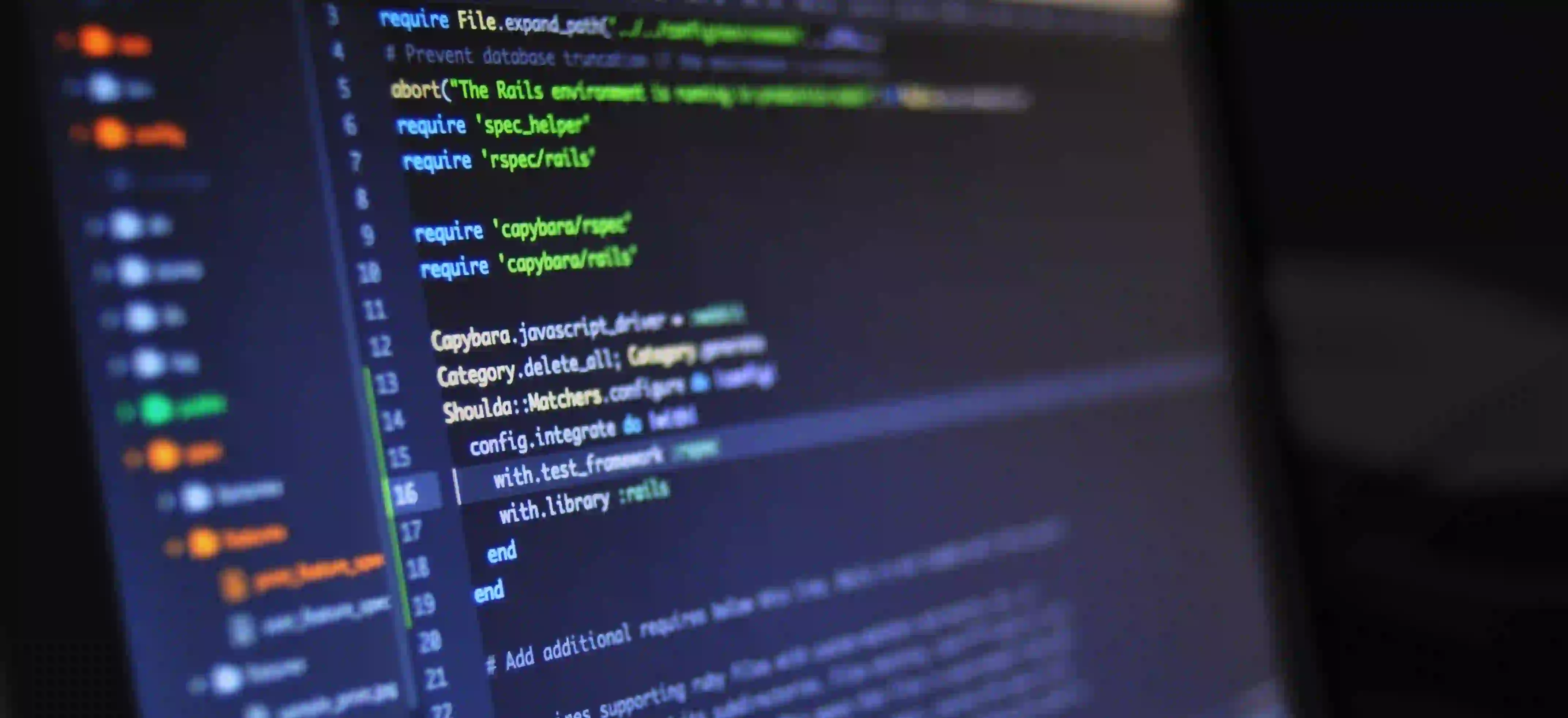
Streamlining Data Processing with Java 8 Collectors
In the world of Java programming, data processing is an integral part of many applications. Whether you're working with collections of data from a database, parsing data from external sources, or simply manipulating in-memory data structures, Java offers a powerful set of tools for processing and transforming data.
With the introduction of Java 8, the language received a significant enhancement with the inclusion of functional programming features. One of the most powerful additions to Java 8 is the Stream API, which provides a functional approach to processing collections of data.
In this article, we'll delve into the world of Java 8 Collectors, a versatile set of tools for transforming and aggregating data within streams. We'll explore the various methods and functionalities provided by Collectors, and demonstrate how they can be used to streamline data processing tasks in Java applications.
Understanding Java 8 Collectors
What are Collectors?
In Java 8, the java.util.stream.Collectors
class provides a set of static factory methods for creating collectors. Collectors are used with the Stream API to perform mutable reduction operations on the elements of the stream. These operations can involve grouping, partitioning, aggregating, and transforming the stream elements into a variety of data structures such as lists, maps, and summarization objects.
Why Use Collectors?
Collectors offer a succinct, expressive, and efficient way to perform complex data processing tasks on streams of data. They encapsulate common patterns of data processing, making the code more readable and reducing the need for boilerplate code. By using collectors, you can write concise and declarative data processing logic, leveraging the power of functional programming in Java.
Common Use Cases for Java 8 Collectors
Let's explore some common scenarios where Java 8 Collectors can be used to streamline data processing tasks.
Grouping Data
One of the most common use cases for Collectors is grouping data based on specific criteria. The Collectors.groupingBy
method allows you to group the elements of a stream into a Map, where the keys are the result of applying a classification function to the elements, and the values are Lists of the elements that share the same key.
List<Person> people = // populate the list
Map<String, List<Person>> peopleByCity =
people.stream()
.collect(Collectors.groupingBy(Person::getCity));
In this example, we are grouping a list of Person
objects by their city
attribute, resulting in a Map<String, List<Person>>
where the keys are city names and the values are lists of people living in each city.
Aggregating Data
Collectors can also be used to perform aggregation operations on stream elements. For example, the Collectors.summarizingInt
method can be used to calculate statistics such as count, sum, min, average, and max for integer attributes of the stream elements.
List<Transaction> transactions = // populate the list
IntSummaryStatistics stats =
transactions.stream()
.collect(Collectors.summarizingInt(Transaction::getAmount));
In this case, we are collecting summary statistics for the amount
attribute of Transaction
objects, providing insights into the distribution of transaction amounts.
Joining Data
Another common use case is joining the elements of a stream into a single String. The Collectors.joining
method concatenates the string representations of the elements, optionally applying a delimiter, prefix, and suffix.
List<String> words = // populate the list
String concatenated =
words.stream()
.collect(Collectors.joining(", ", "[", "]"));
Here, we are joining a list of words into a comma-separated string, enclosed within square brackets.
Custom Collectors
In addition to the built-in collectors provided by the Collectors
class, Java 8 allows you to create custom collectors by implementing the Collector
interface. Custom collectors are useful when you need to perform complex data processing operations that cannot be achieved using the built-in collector methods.
When creating a custom collector, you need to provide implementations for four key functions: supplier, accumulator, combiner, and finisher. The Collector.of
method allows you to create a custom collector by specifying these functions.
Collector<Person, StringJoiner, String> personNameCollector =
Collector.of(
() -> new StringJoiner(" | "),
(joiner, person) -> joiner.add(person.getName().toUpperCase()),
StringJoiner::merge,
StringJoiner::toString
);
List<Person> people = // populate the list
String names =
people.stream()
.collect(personNameCollector);
In this example, we are creating a custom collector to concatenate the names of people into a single string, separated by a pipe symbol.
To Wrap Things Up
In conclusion, Java 8 Collectors provide a powerful and expressive way to streamline data processing tasks when working with streams. Whether you need to group, aggregate, join, or perform custom operations on stream elements, collectors enable you to write concise and declarative code, leveraging the functional programming features of Java 8.
By understanding the capabilities of Java 8 Collectors and incorporating them into your data processing workflows, you can write more efficient, maintainable, and expressive code for handling collections of data in Java applications.
To further explore the capabilities of Java 8 Collectors and the Stream API, check out the official Java documentation.
Start leveraging the power of Java 8 Collectors in your data processing tasks and unlock the full potential of functional programming in Java!