Supercharge Your Google App Productivity with Google Script
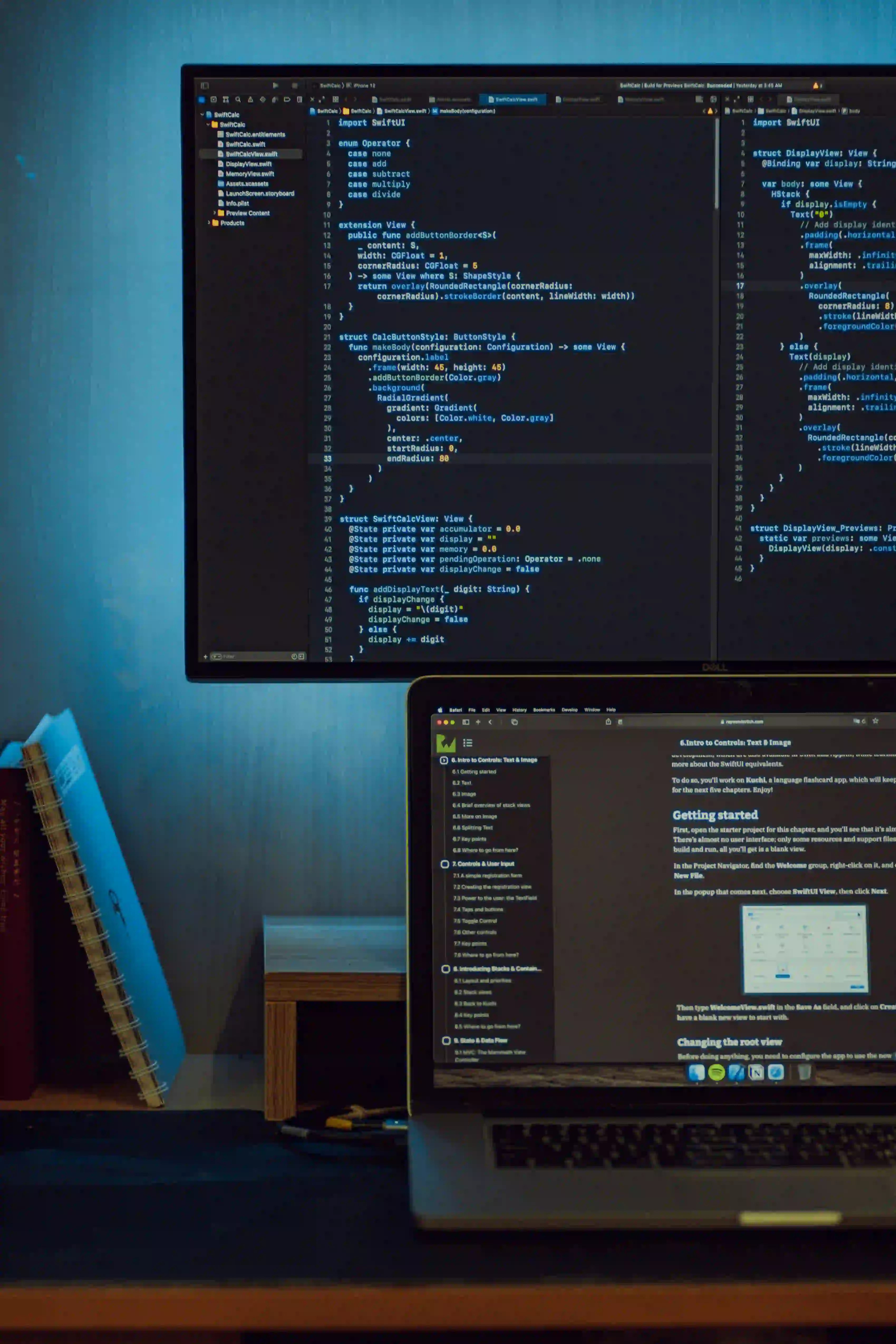
Supercharge Your Google App Productivity with Google Script
If you use Google Apps, you know how powerful they can be. But did you know that you can supercharge your productivity by automating tasks and creating custom functions using Google Script? In this post, we'll explore how you can use Google Script to enhance the functionality of your favorite Google Apps, such as Sheets, Docs, and Forms.
What is Google Script?
Google Script is a subset of JavaScript that allows you to automate tasks and extend the functionality of various Google Apps. Whether you want to create custom functions in Google Sheets, automate emails in Gmail, or build custom add-ons for Google Docs, Google Script provides a powerful and versatile platform to achieve these tasks.
One of the great advantages of Google Script is that it runs on Google's servers, which means you don't have to worry about managing infrastructure or hosting. It also integrates seamlessly with other Google Apps, allowing you to access and manipulate your data with ease.
Getting Started with Google Script
To get started with Google Script, you need to have a Google account. Once you're logged in, you can access Google Script by opening any of the Google Apps and navigating to the "Tools" menu, where you'll find the option to open the script editor. This will open a new tab with the Google Script editor, where you can write and manage your scripts.
Creating Custom Functions in Google Sheets
Google Sheets is a powerful tool for organizing and analyzing data, and with Google Script, you can take its functionality to the next level by creating custom functions. Let's say you have a sheet with sales data, and you want to calculate the total revenue for a specific product category. Instead of manually entering the formula for each category, you can create a custom function to automate this process.
function calculateTotalRevenue(category) {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var data = sheet.getRange(2, 1, sheet.getLastRow(), 3).getValues();
var total = 0;
for (var i = 0; i < data.length; i++) {
if (data[i][0] == category) {
total += data[i][2];
}
}
return total;
}
In this example, we define a function calculateTotalRevenue
that takes a category
parameter. The function then retrieves the data from the active sheet, iterates through it to find the matching category, and calculates the total revenue. This custom function can be used in any cell within the sheet, just like any built-in function.
Automating Tasks in Google Apps
Google Script allows you to automate repetitive tasks across various Google Apps. For instance, you can create a script to automatically send personalized email reminders from a Google Sheets database, or to generate custom reports in Google Docs based on data from Google Forms.
Here's an example of a simple script to send personalized email reminders:
function sendEmailReminder() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var data = sheet.getRange(2, 1, sheet.getLastRow(), 3).getValues();
for (var i = 0; i < data.length; i++) {
var name = data[i][0];
var email = data[i][1];
var dueDate = data[i][2];
var today = new Date();
if (dueDate < today) {
MailApp.sendEmail(email, 'Reminder', 'Hi ' + name + ', This is a reminder for the task due on ' + dueDate);
}
}
}
In this script, we retrieve data from a sheet and iterate through each row to check if a task is overdue. If it is, we send a personalized email reminder to the task owner using the MailApp.sendEmail
function.
Creating Custom Add-ons for Google Docs
Google Script also allows you to build custom add-ons to extend the functionality of Google Docs. Add-ons can be used to automate document processing, integrate with external services, or provide custom formatting options.
Let's say you want to create a custom add-on to automatically generate a table of contents for a long document in Google Docs:
function generateTableOfContents() {
var body = DocumentApp.getActiveDocument().getBody();
var headers = body.getParagraphs().filter(function (paragraph) {
return paragraph.getHeading() != DocumentApp.ParagraphHeading.NORMAL;
});
var toc = 'Table of Contents\n';
for (var i = 0; i < headers.length; i++) {
var text = headers[i].getText();
var level = headers[i].getHeading();
var indent = ' '.repeat(level - 1);
toc += indent + text + '\n';
}
body.insertParagraph(0, toc);
}
In this example, we define a function generateTableOfContents
that retrieves all the headers in the document and creates a table of contents based on their levels. This add-on can be triggered from the "Extensions" menu in Google Docs, providing a quick and easy way to generate a table of contents for long documents.
My Closing Thoughts on the Matter
Google Script is a powerful tool for enhancing the functionality of Google Apps, allowing you to automate tasks, create custom functions, and build custom add-ons. Whether you're a business user looking to streamline your workflows or a developer looking to extend the capabilities of Google Apps, Google Script provides a versatile platform to achieve your goals.
By leveraging the capabilities of Google Script, you can save time, reduce errors, and unlock new possibilities for using Google Apps in your day-to-day work. So why not give it a try and supercharge your Google App productivity today?
To learn more about Google Script and its capabilities, check out the official documentation. Happy scripting!