Troubleshooting Common Android Development Issues
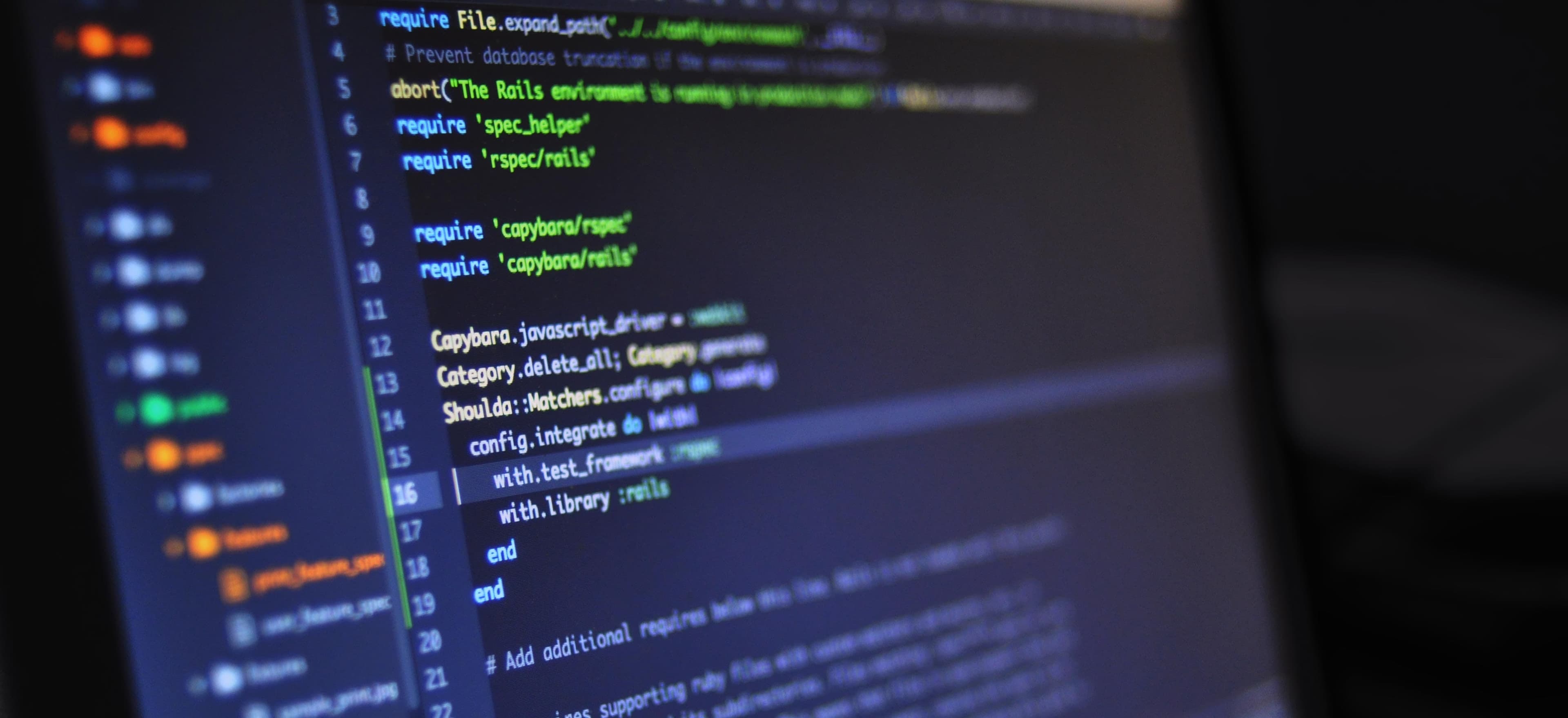
- Published on
1. Introduction
When working on Android application development, encountering issues is inevitable. However, with the right troubleshooting techniques, you can easily overcome these hurdles. In this blog post, we will explore some common Android development issues and how to troubleshoot them effectively using Java.
2. Issue: App Crashes on Startup
Troubleshooting Steps
- Check the Manifest File: Ensure that the
Launcher Activity
is defined correctly in the AndroidManifest.xml file. - Review Logcat: Look for any error messages in Logcat to identify the potential cause of the crash.
- Permissions: Verify if the app requires any specific permissions that are missing.
Code Example
public class MainActivity extends AppCompatActivity {
// ...
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// ...
}
// ...
}
In this example, the onCreate
method sets the content view and performs other initialization tasks. If the app crashes on startup, it is crucial to check for any issues within this method.
3. Issue: Networking Errors
Troubleshooting Steps
- Network Permission: Ensure the app has the necessary permissions to access the network.
- Network Connection: Verify if the device is connected to the internet.
- Use AsyncTask or Threads: When performing network operations on the main thread, it can lead to
NetworkOnMainThreadException
. Utilize AsyncTask or background threads for network operations.
Code Example
public class NetworkTask extends AsyncTask<String, Void, String> {
// ...
@Override
protected String doInBackground(String... params) {
// Perform network operations here
// ...
return result;
}
// ...
}
The AsyncTask
class allows you to perform background operations and publish results on the UI thread without causing any blocked UI.
4. Issue: Memory Leaks
Troubleshooting Steps
- Use Android Profiler: Identify memory allocation patterns and recognize potential memory leaks using tools like Android Profiler in Android Studio.
- Context Cleanup: Be cautious with long-lived references to
Context
objects, as they can lead to memory leaks. - Static Inner Classes: Avoid using static inner classes as they hold an implicit reference to the outer class, preventing it from being garbage collected.
Code Example
public class MyActivity extends AppCompatActivity {
private static MyAsyncTask myTask;
// ...
public void startTask() {
myTask = new MyAsyncTask();
myTask.execute();
}
// ...
}
In this example, the MyActivity
class holds a static reference to MyAsyncTask
, which can potentially cause a memory leak.
5. Issue: UI Not Responsive
Troubleshooting Steps
- Optimize UI Rendering: Utilize tools such as
Hierarchy Viewer
orLayout Inspector
to identify UI elements causing rendering issues. - Background Processing: Move heavy processing tasks to background threads or use mechanisms like
Handler
andLooper
to update the UI. - ProGuard: If using ProGuard for code obfuscation, ensure that necessary classes and methods are not obfuscated, leading to runtime issues.
Code Example
public class MainActivity extends AppCompatActivity {
// ...
private Handler mHandler = new Handler(Looper.getMainLooper());
private void updateUI() {
mHandler.post(new Runnable() {
@Override
public void run() {
// Update UI here
// ...
}
});
}
// ...
}
Using Handler
and Looper
in this example allows you to update the UI from a background thread safely.
6. Issue: Slow Database Operations
Troubleshooting Steps
- Database Indexing: Ensure that the database tables are appropriately indexed for faster query performance.
- Use Transactions: Group database operations into transactions to minimize the number of disk writes and improve speed.
- Optimize Queries: Review and optimize SQL queries for efficiency, avoiding unnecessary operations and joins.
Code Example
public void updateRecord(String data) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
db.beginTransaction();
try {
// Perform database operations
db.setTransactionSuccessful();
} finally {
db.endTransaction();
}
}
The use of transactions in this example helps to maintain data consistency and optimize database operations.
7. Conclusion
In the world of Android development, troubleshooting is an essential skill that every developer must possess. By understanding common issues and their respective troubleshooting steps, you can streamline the development process and deliver robust, high-quality Android applications. Keep exploring and learning, and you'll be well-equipped to handle any challenges that come your way. Happy coding!
In conclusion, while troubleshooting Android development issues, it's important to stay informed and continuously seek knowledge through reputable sources such as the official Android Developer Guide. This will ensure that your troubleshooting techniques align with best practices and the latest industry standards.