Deploying Artifacts to Nexus Repository Using Maven
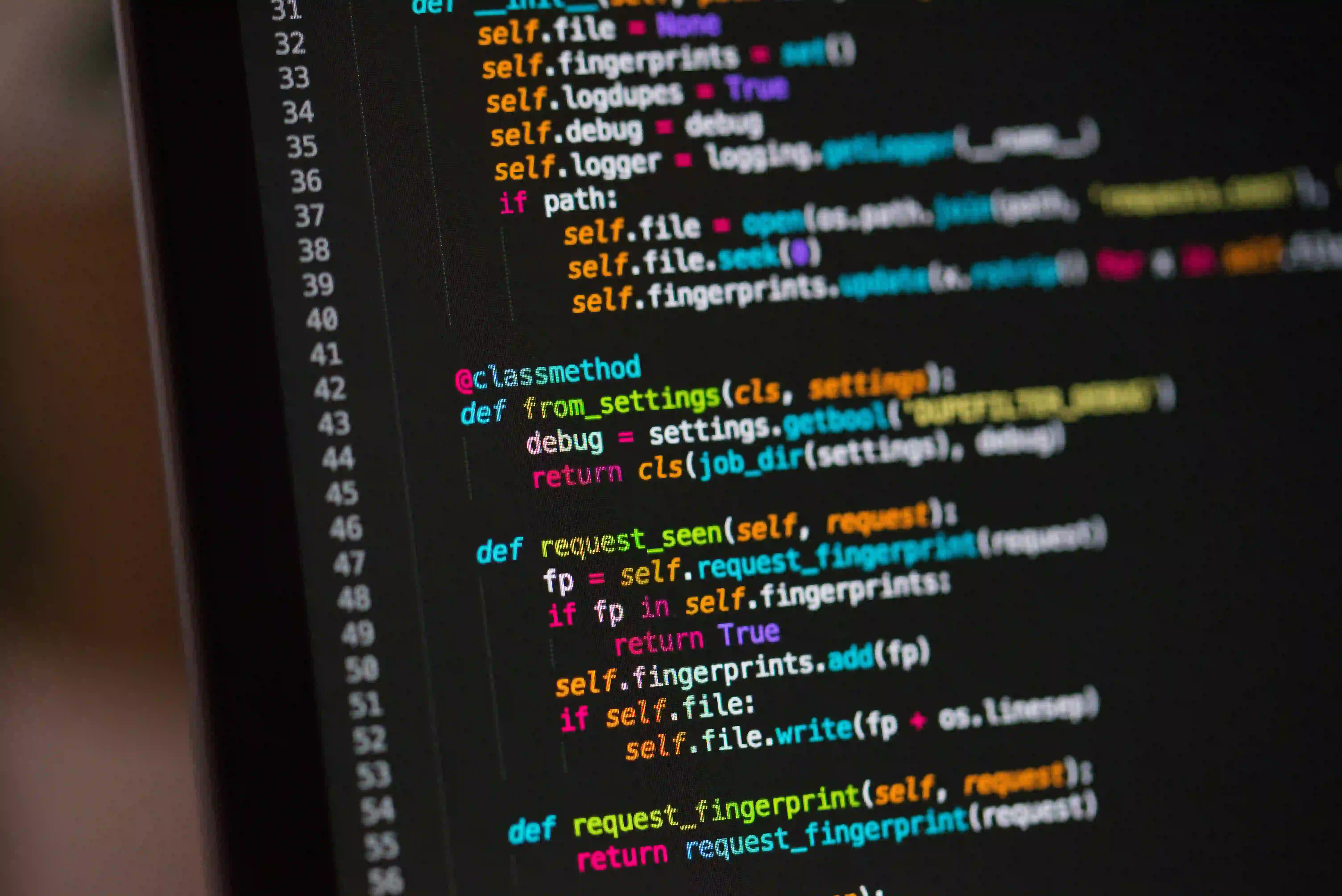
Deploying Artifacts to Nexus Repository Using Maven
In software development, a crucial aspect is managing dependencies and artifacts. Maven, a popular build automation tool, provides the capability to deploy these artifacts to a central repository such as Nexus. This allows for efficient sharing and reusability of components across different projects. In this article, we will explore the process of deploying artifacts to Nexus repository using Maven.
Prerequisites
Before we begin, ensure that you have the following prerequisites in place:
- Maven installed on your system
- Access to a Nexus repository
Configuring Deployment in Maven
To deploy artifacts to Nexus using Maven, you need to configure the deployment settings in the pom.xml
file of your project. Add the following configuration within the <project>
tag:
<distributionManagement>
<repository>
<id>nexus-releases</id>
<url>https://your-nexus-repository/releases</url>
</repository>
<snapshotRepository>
<id>nexus-snapshots</id>
<url>https://your-nexus-repository/snapshots</url>
</snapshotRepository>
</distributionManagement>
Replace https://your-nexus-repository/releases
and https://your-nexus-repository/snapshots
with the actual URLs of your Nexus repository for releases and snapshots respectively.
Setting Up Server Credentials
Once the repository configuration is in place, Maven needs the credentials to authenticate and deploy artifacts to Nexus. These credentials should be kept secure and not hardcoded in the pom.xml
file for security reasons.
Maven supports storing server credentials in the settings.xml
file. Add the server configuration for Nexus repository within the <servers>
tag:
<servers>
<server>
<id>nexus-releases</id>
<username>your-username</username>
<password>your-password</password>
</server>
<server>
<id>nexus-snapshots</id>
<username>your-username</username>
<password>your-password</password>
</server>
</servers>
Replace your-username
and your-password
with your Nexus repository credentials.
Deploying Artifacts
With the configuration in place, you can now deploy artifacts to Nexus using the following Maven commands:
- Deploy a release artifact:
mvn deploy
- Deploy a snapshot artifact:
mvn deploy -DrepositoryId=nexus-snapshots
Repository Structure
When deploying artifacts to Nexus, it's essential to understand the repository structure used by Nexus. Maven repositories in Nexus follow a standardized layout with the following main directories:
releases
: Stores stable release artifactssnapshots
: Stores snapshot artifacts that are works-in-progress or unstable versions
Understanding this structure is important for organizing artifacts and managing dependencies effectively.
Best Practices for Artifact Deployment
To ensure a smooth deployment process, consider the following best practices:
Versioning
Follow a standardized versioning scheme for your artifacts. Semantic versioning is a widely adopted approach that conveys meaning about the underlying changes in the artifact.
GPG Signature
Consider signing your artifacts with GPG (GNU Privacy Guard) to provide a level of trust and security for the consumers of your artifacts.
Repository Cleanup
Regularly clean up old and unused artifacts from your Nexus repository to maintain a clean and efficient repository.
Final Thoughts
In this article, we have covered the process of deploying artifacts to Nexus repository using Maven. By configuring the deployment settings, setting up server credentials, understanding the repository structure, and following best practices, you can effectively manage and share your project's artifacts. Incorporating these practices into your build and deployment workflows will contribute to a more streamlined and organized development process.
Now that you have a good understanding, it's time to put this knowledge into practice. Happy deploying!