Common Memory Issues in Java Virtual Machines
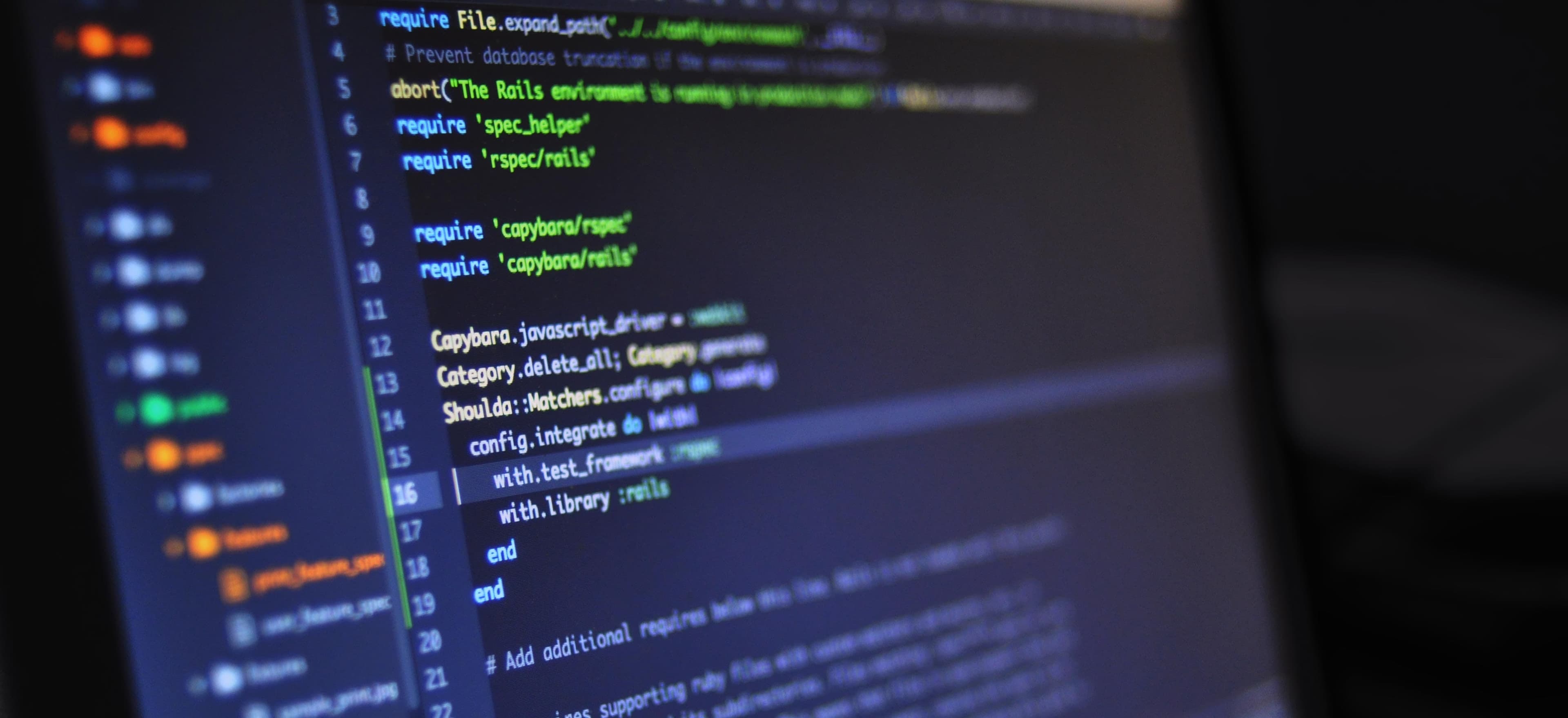
- Published on
Common Memory Issues in Java Virtual Machines
Java is widely used for building robust and scalable applications, thanks to its platform independence and automatic memory management. However, like any other programming language, Java applications are susceptible to memory-related issues that can lead to performance degradation and even application crashes. In this article, we will delve into some of the common memory issues in Java Virtual Machines (JVM) and explore how to identify, mitigate, and prevent them.
1. Memory Leaks
Memory leaks occur when objects that are no longer being used are not garbage collected, leading to a gradual increase in memory consumption. In Java, memory leaks are often caused by inadvertently holding references to objects, preventing the garbage collector from reclaiming the memory they occupy.
Example of Memory Leak
public class MemoryLeakExample {
private static List<Object> list = new ArrayList<>();
public void addToLeakedMemory() {
while (true) {
list.add(new Object());
}
}
}
In this example, the addToLeakedMemory
method continuously adds objects to the list
without ever removing them, eventually leading to a memory leak.
Why It Happens
The list
holds strong references to the objects, preventing them from being garbage collected, thus causing the memory leak.
How to Avoid Memory Leaks
-
Use Weak References: Replace strong references with weak references when appropriate, allowing objects to be garbage collected even if they are referenced weakly.
-
Remove Unused References: Ensure that objects are removed from collections when they are no longer needed, allowing them to be garbage collected.
-
Use Profiling Tools: Utilize memory profiling tools like YourKit, JProfiler, or VisualVM to identify and diagnose memory leaks in Java applications.
2. OutOfMemoryError
OutOfMemoryError is a common issue that occurs when the JVM runs out of memory. This can happen due to various reasons such as memory leaks, improper memory allocation, or simply insufficient memory to support the application's workload.
Example of OutOfMemoryError
public class OutOfMemoryExample {
public static void main(String[] args) {
List<byte[]> bytes = new ArrayList<>();
while (true) {
bytes.add(new byte[1048576]); // Allocate 1MB memory in each iteration
}
}
}
In this example, the application keeps allocating 1MB of memory in a loop, eventually leading to an OutOfMemoryError.
Why It Happens
The loop continuously allocates memory without releasing any, eventually exhausting the available memory.
How to Avoid OutOfMemoryError
-
Properly Size JVM Heap: Configure the JVM heap size according to the application's memory requirements using parameters like
-Xms
and-Xmx
. -
Optimize Memory Usage: Analyze the application's memory consumption and optimize data structures and algorithms to minimize memory usage.
-
Use Memory Profilers: Employ memory profiling tools to identify memory hotspots and optimize memory usage.
3. Garbage Collection Overhead
While garbage collection is a key feature of Java's memory management, inefficient garbage collection can lead to increased CPU utilization and application pauses, impacting overall performance.
Example of Garbage Collection Overhead
public class GCOverheadExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
for (int i = 0; i < Integer.MAX_VALUE; i++) {
list.add(String.valueOf(i));
}
}
}
In this example, the continuous addition of strings to the list can lead to substantial garbage collection overhead, impacting application performance.
Why It Happens
The continuous allocation of objects without proper memory management can overload the garbage collector, leading to increased overhead.
How to Mitigate Garbage Collection Overhead
-
Tune Garbage Collection Settings: Adjust garbage collection settings such as heap size, garbage collector type, and collection strategies based on the application's memory and performance requirements.
-
Minimize Object Instantiation: Avoid unnecessary object creation and reuse objects whenever possible to reduce the frequency of garbage collection.
-
Use Concurrent Garbage Collectors: Consider using concurrent garbage collectors like G1GC or CMS, which minimize application pause times by performing garbage collection concurrently with the application's execution.
A Final Look
In Java applications, memory issues can significantly impact performance and stability. Understanding and addressing common memory problems such as memory leaks, OutOfMemoryError, and garbage collection overhead is crucial for building robust and efficient Java applications.
By utilizing techniques such as using weak references, optimizing memory usage, and tuning garbage collection settings, developers can mitigate and prevent these memory-related issues, ensuring that Java applications operate smoothly and efficiently.
To dive deeper into Java memory management and performance optimization, consider exploring the Java Memory Management Guide and Tuning Java Garbage Collection.
Remember, proactive memory management is key to ensuring that your Java applications deliver optimal performance and reliability.
Checkout our other articles