Building a Lightweight Web App with PrimeFaces: Efficient Integration of JSF, Guice, and MyBatis
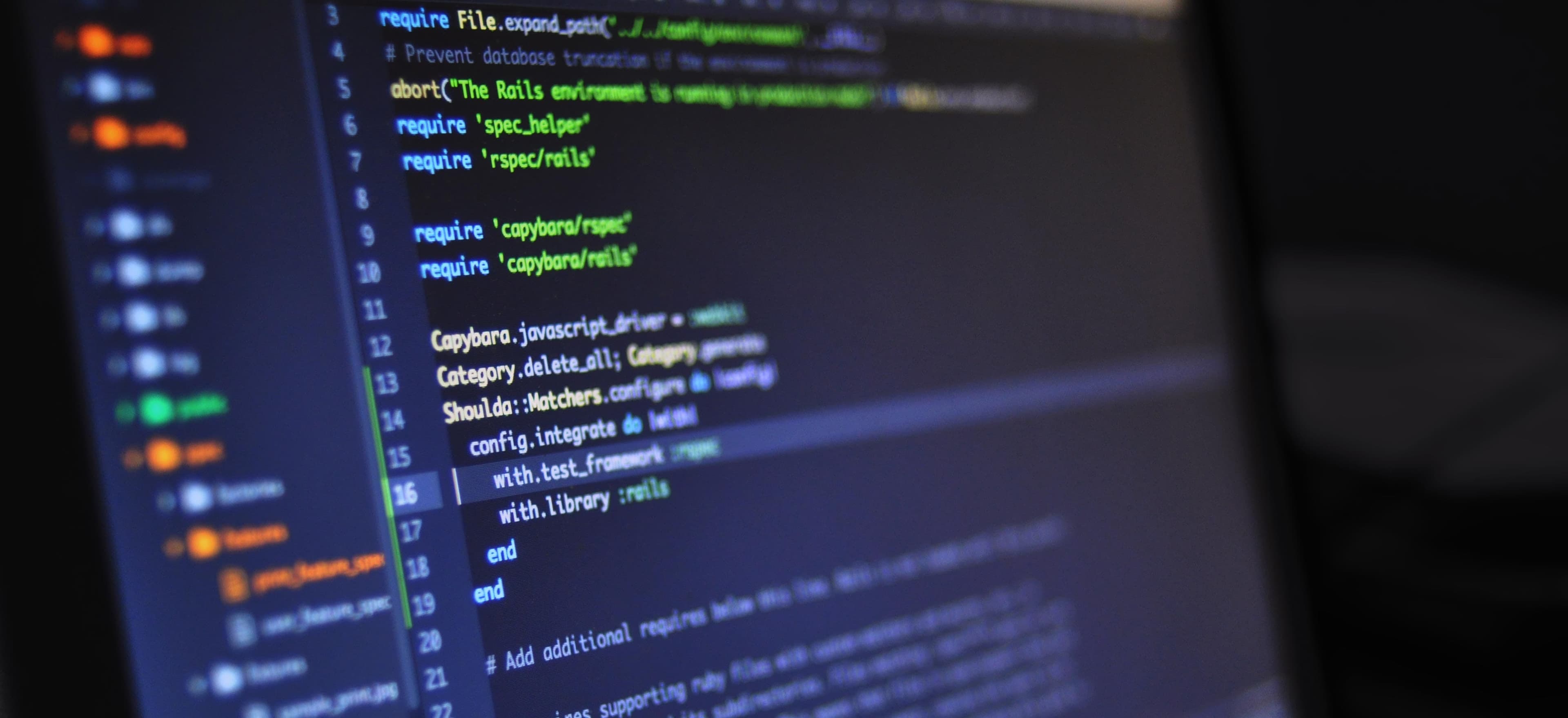
- Published on
Building a Lightweight Web App with PrimeFaces
In today's fast-paced world, developing lightweight web applications with a seamless user interface is crucial. As a Java developer, you often encounter the need to build web applications that are not only robust and efficient but also visually appealing. In this blog post, we will explore the efficient integration of JavaServer Faces (JSF), Guice, MyBatis, and PrimeFaces to develop a lightweight web app.
What is PrimeFaces?
PrimeFaces is a popular open-source user interface (UI) component library for JavaServer Faces (JSF). It provides a rich set of components, themes, and user interface solutions for web applications. PrimeFaces simplifies the development of JSF applications by offering a wide range of powerful and customizable UI components.
Why Use PrimeFaces?
Using PrimeFaces in your web application development offers several benefits, including:
-
Rich Set of Components: PrimeFaces provides an extensive collection of UI components such as input fields, buttons, calendars, data tables, and charts, allowing you to create visually appealing and functional user interfaces.
-
Customization: PrimeFaces components are highly customizable, enabling you to tailor the look and feel of your web application according to your requirements.
-
Responsive Design: PrimeFaces supports responsive design, ensuring that your application looks and functions well across various devices and screen sizes.
Now, let's dive into the process of integrating PrimeFaces with JSF, Guice, and MyBatis to build a lightweight web application.
Integration of PrimeFaces with JSF, Guice, and MyBatis
Setting Up the Project Structure
To begin, let's set up the project structure for our lightweight web application. We'll use Maven as the build tool for managing dependencies and project configuration. The following is a basic structure for the project:
project
│ pom.xml
└───src
└───main
└───java
│ └───com.example
│ │ ApplicationModule.java
│ │ User.java
│ └───dao
│ │ UserDAO.java
│ └───mapper
│ │ UserMapper.java
└───resources
└───mybatis
│ UserMapper.xml
Integrating PrimeFaces with JSF
First, we need to include the PrimeFaces dependency in our pom.xml
file. This will allow us to utilize the PrimeFaces components in our JSF pages. Add the following dependency to the pom.xml
file:
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>10.0.0</version>
</dependency>
Now, we can create JSF pages and leverage PrimeFaces components within them to build the user interface of our web application.
Configuring Guice
Guice is a lightweight dependency injection framework by Google, providing support for binding and managing dependencies within an application. We can configure Guice to manage the dependencies of our web application, including the services and data access objects (DAOs).
public class ApplicationModule extends AbstractModule {
@Override
protected void configure() {
// Bind services and DAOs
bind(UserService.class).to(UserServiceImpl.class);
bind(UserDAO.class).to(UserDAOImpl.class);
}
}
In the above example, we bind the UserService
interface to its implementation UserServiceImpl
and bind the UserDAO
interface to its implementation UserDAOImpl
.
Integrating MyBatis
MyBatis is a simple yet powerful object-relational mapping (ORM) framework that simplifies the interaction with a relational database. We can use MyBatis to map Java objects to database records and perform CRUD operations.
Let's define a MyBatis mapper interface for the User
entity:
public interface UserMapper {
@Select("SELECT * FROM users")
List<User> getAllUsers();
@Select("SELECT * FROM users WHERE id = #{id}")
User getUserById(int id);
@Insert("INSERT INTO users (name, email) VALUES (#{name}, #{email})")
@Options(useGeneratedKeys = true, keyProperty = "id")
void insertUser(User user);
@Update("UPDATE users SET name = #{name}, email = #{email} WHERE id = #{id}")
void updateUser(User user);
@Delete("DELETE FROM users WHERE id = #{id}")
void deleteUser(int id);
}
The UserMapper
interface defines SQL statements for retrieving, inserting, updating, and deleting user records.
Building the Web UI with PrimeFaces Components
Now, let's create a JSF page (users.xhtml
) and utilize PrimeFaces components to display and manage user data:
<h:form>
<p:dataTable var="user" value="#{userBean.users}">
<p:column headerText="ID">
<h:outputText value="#{user.id}" />
</p:column>
<p:column headerText="Name">
<h:outputText value="#{user.name}" />
</p:column>
<p:column headerText="Email">
<h:outputText value="#{user.email}" />
</p:column>
</p:dataTable>
</h:form>
In this example, we use the p:dataTable
component to display a table of users, with columns for ID, name, and email. The #{userBean.users}
expression retrieves the list of users from the associated managed bean.
Why Use PrimeFaces Components?
PrimeFaces components offer several advantages:
-
Rich Functionality: PrimeFaces components come with built-in functionality such as sorting, filtering, and pagination for data tables, reducing the need for manual implementation.
-
Ajax Support: PrimeFaces components support Ajax, allowing for seamless updating of specific parts of the UI without refreshing the entire page.
-
Theming and Skinning: PrimeFaces provides themes and skins to customize the appearance of components, ensuring a consistent and polished look for the web application.
Closing the Chapter
In this blog post, we have explored the efficient integration of JSF, Guice, MyBatis, and PrimeFaces to build a lightweight web application. By leveraging PrimeFaces components for the user interface, Guice for dependency injection, and MyBatis for database interactions, we have created a robust and visually appealing web application.
Integrating these technologies enables Java developers to streamline the development process and deliver high-quality web applications that meet modern user experience standards.
I hope this guide has provided you with valuable insights into building lightweight web apps with PrimeFaces. Happy coding!