Optimizing Custom User Types in GORM
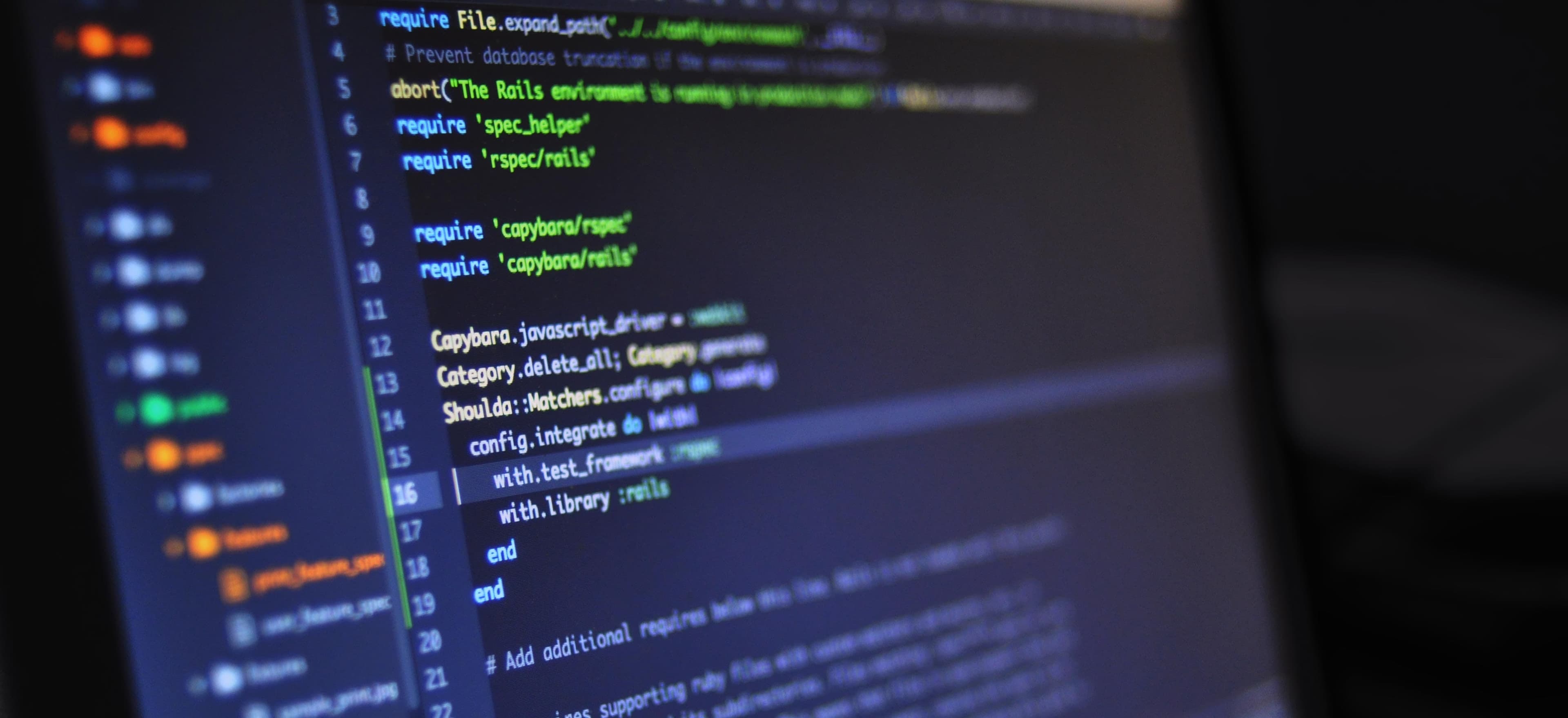
- Published on
Understanding Custom User Types in GORM
In Grails applications, GORM (Grails Object Relational Mapping) provides a powerful tool for interacting with databases. GORM enables developers to work with domain classes and persistent data in a simplified and efficient manner. One of the exceptional features of GORM is the ability to define custom user types, which allow developers to extend the capabilities of their domain classes and handle complex data types more effectively.
In this article, we will delve into the concept of custom user types in GORM, understand their significance, and explore optimization techniques to make the most of this feature.
What are Custom User Types in GORM?
At its core, GORM's custom user types are a way to map complex data types in database columns. By default, GORM provides support for a wide range of basic data types, including strings, numbers, dates, and boolean values. However, in real-world applications, it is common to encounter scenarios where we need to store and retrieve complex data types, such as JSON, XML, or custom objects, in our domain classes.
This is where custom user types come into play. They enable us to define and use custom data types that are not natively supported by GORM. By implementing custom user types, developers can seamlessly map their complex data structures to the underlying database, providing a more flexible and tailored approach to data persistence.
Advantages of Using Custom User Types
1. Improved Data Encapsulation
Custom user types allow developers to encapsulate complex data structures within domain classes, promoting better organization and encapsulation of data. This encapsulation helps in maintaining clean and coherent domain models, enhancing the overall readability and maintainability of the codebase.
2. Seamless Integration with Existing Code
By utilizing custom user types, developers can seamlessly integrate custom data types into their domain classes without the need to compromise the existing code structure. This flexibility enables the incorporation of complex data representations without cluttering the domain classes with intricate database handling logic.
3. Enhanced Database Interoperability
Custom user types empower developers to work with varied database systems and handle custom data types consistently across different database platforms. This unified approach to data mapping simplifies database interoperability and ensures a consistent data representation regardless of the underlying database technology.
Implementing Custom User Types in GORM
To illustrate the implementation of custom user types in GORM, let's consider a scenario where we need to store and retrieve JSON data in our Grails domain class. We will create a custom user type to handle the JSON serialization and deserialization seamlessly.
import com.fasterxml.jackson.databind.ObjectMapper
import org.hibernate.type.AbstractSingleColumnStandardBasicType
import org.hibernate.type.descriptor.sql.VarcharTypeDescriptor
import org.hibernate.type.descriptor.java.StringTypeDescriptor
class JsonUserType extends AbstractSingleColumnStandardBasicType {
JsonUserType() {
super(VarcharTypeDescriptor.INSTANCE, new JsonTypeDescriptor())
}
@Override
public String getName() {
"json"
}
static class JsonTypeDescriptor extends StringTypeDescriptor {
JsonTypeDescriptor() {
setJavaTypeDescriptor(StringTypeDescriptor.INSTANCE)
}
@Override
public <X> X fromString(String string) {
if (string) {
return new ObjectMapper().readValue(string, Object.class) as X
}
return null
}
@Override
public String toString(Object value) {
if (value) {
return new ObjectMapper().writeValueAsString(value)
}
return null
}
}
}
In the above code snippet, we define a custom user type JsonUserType
that extends AbstractSingleColumnStandardBasicType
and implements custom serialization and deserialization logic for JSON data. This user type leverages Jackson's ObjectMapper
to handle the JSON conversion seamlessly.
Why Use Custom User Types?
The use of custom user types in GORM offers several compelling advantages:
1. Flexibility in Data Representation
Custom user types empower developers to represent complex data structures in database columns, providing the flexibility to store and retrieve non-standard data types effortlessly. This flexibility is invaluable when dealing with unconventional data storage requirements in domain classes.
2. Simplified Data Persistence
By encapsulating the conversion logic within custom user types, developers can seamlessly persist and retrieve complex data types without cluttering the domain classes with intricate database interaction code. This simplification enhances the overall readability and maintainability of the codebase.
3. Streamlined Mapping of Custom Data Types
Custom user types streamline the mapping of custom data types to the underlying database, ensuring a cohesive and standardized approach to handling complex data structures. This consistency is particularly beneficial when working with diverse database systems and platforms.
Optimizing Custom User Types
While implementing custom user types brings notable advantages, optimizing their usage is crucial for maximizing performance and maintainability.
1. Efficient Serialization and Deserialization Logic
When defining custom user types, it is imperative to optimize the serialization and deserialization logic to ensure efficient conversion of data. Performance bottlenecks can arise if the conversion processes are not optimized, impacting the overall responsiveness of the application.
2. Consistent Error Handling
Robust error handling within custom user types is essential to maintain the integrity of data operations. By incorporating thorough error handling mechanisms, developers can ensure that custom user types gracefully handle exceptional scenarios, such as data conversion failures or format inconsistencies.
3. Compatibility Across Database Systems
Optimizing custom user types involves ensuring compatibility and consistent behavior across different database systems. Validating the behavior of custom user types across various database platforms helps in preemptively addressing any compatibility issues and ensuring seamless interoperability.
4. Performance Benchmarking
Conducting performance benchmarking and profiling of custom user types is instrumental in identifying potential optimizations. By analyzing the performance characteristics of custom user types, developers can pinpoint areas for improvement and fine-tune the implementation for optimal efficiency.
Closing the Chapter
Custom user types in GORM offer a versatile mechanism for handling complex data types within domain classes. By embracing custom user types, developers can enhance the flexibility, maintainability, and efficiency of their data persistence strategies. Optimizing the usage of custom user types through efficient serialization logic, robust error handling, and compatibility validation is vital to maximize their benefits.
In essence, leveraging custom user types empowers developers to tailor the data persistence layer to suit diverse and intricate data storage requirements, contributing to the overall robustness and versatility of Grails applications.
To explore more about GORM and custom user types, visit the official documentation.
Incorporating custom user types in GORM unlocks the potential to effectively manage and interact with diverse and intricate data structures within the context of Grails applications. Through optimizing the implementation and usage of custom user types, developers can ensure seamless data persistence and enhanced flexibility in managing custom data types.
Checkout our other articles