Optimizing Data Crunching for Efficient Big Data Analysis
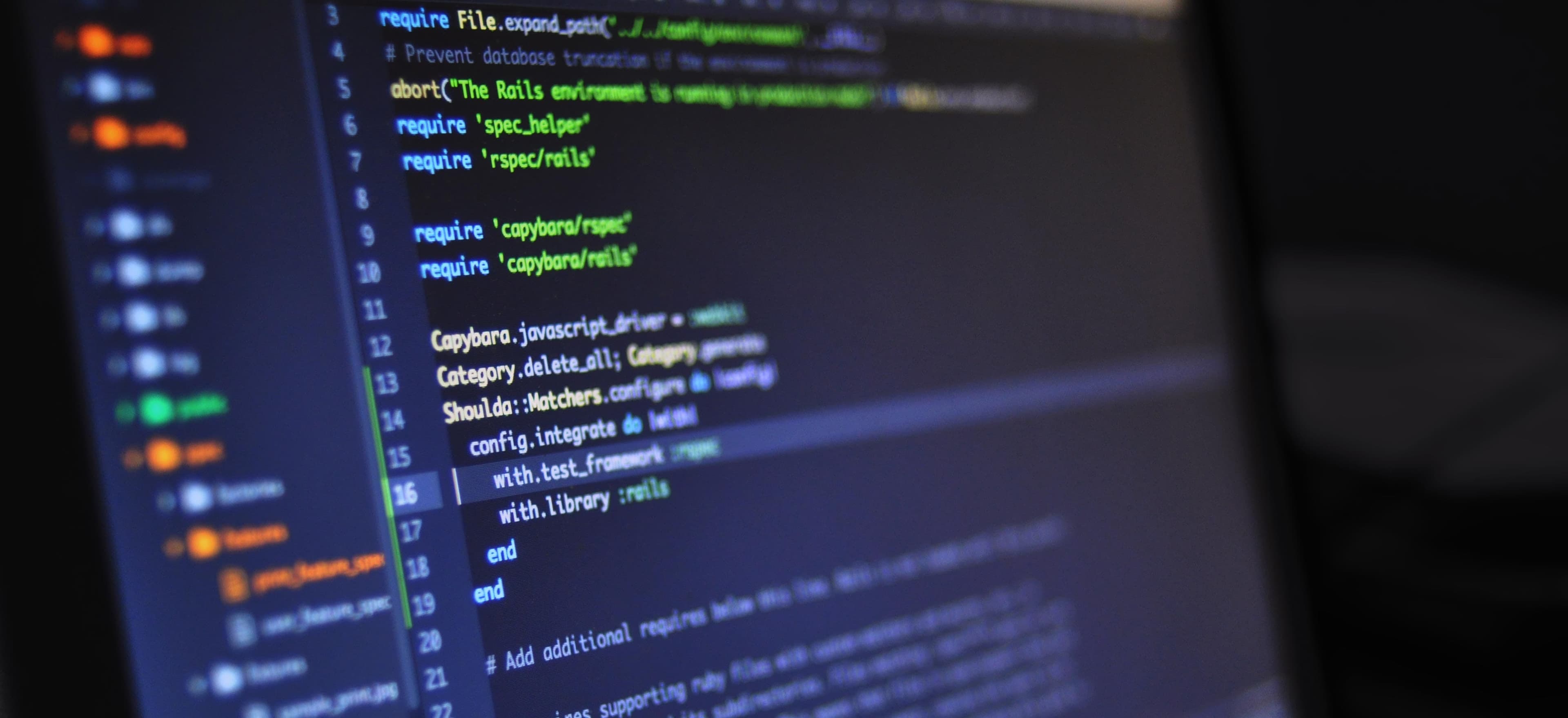
- Published on
Mastering Big Data Analysis with Java
In the era of big data, efficient processing and analysis are essential for extracting valuable insights. Java, with its powerful libraries and ecosystem, offers a robust platform for handling large-scale data processing. From data ingestion to complex analytics, Java provides the tools needed to tackle the challenges of big data. In this blog post, we will explore how to optimize data crunching for efficient big data analysis using Java.
Leveraging Multithreading for Parallel Processing
One of the key strategies for optimizing big data analysis in Java is leveraging multithreading to achieve parallel processing. By utilizing Java's ExecutorService
and ThreadPoolExecutor
, we can distribute the workload across multiple threads, enabling concurrent execution of tasks.
ExecutorService executor = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors());
// Submitting tasks for parallel execution
executor.submit(() -> {
// Code for data processing task 1
});
executor.submit(() -> {
// Code for data processing task 2
});
// Shutting down the executor after all tasks are completed
executor.shutdown();
By parallelizing data processing tasks, we can significantly reduce the overall processing time, especially when dealing with large datasets. However, it's crucial to carefully manage concurrency and synchronization to avoid potential data integrity issues.
Batch Processing with Java Streams
Java Streams provide a functional approach to processing collections of data. When dealing with big data, utilizing Java Streams for batch processing can offer significant performance improvements. The functional programming style allows for concise and expressive code, while the internal optimizations in Java Streams can enhance overall efficiency.
Consider the following example of using Java Streams for data aggregation:
List<Double> data = Arrays.asList(1.2, 2.5, 3.8, 4.1, 5.4);
double sum = data.parallelStream()
.mapToDouble(Double::doubleValue)
.sum();
System.out.println("Sum of data: " + sum);
In this example, the use of parallelStream()
enables parallel processing of the data, taking advantage of multicore processors for faster aggregation. When working with large datasets, leveraging parallel streams can lead to substantial performance gains.
Memory Management and Garbage Collection Optimization
Efficient memory management is critical for big data applications to prevent excessive garbage collection pauses and minimize memory overhead. Utilizing Java's memory management tools, such as tuning the heap size, garbage collection algorithms, and memory allocation, can greatly impact the performance of data crunching operations.
For instance, setting the initial and maximum heap size using JVM options can optimize memory allocation for big data processing:
java -Xms4G -Xmx8G BigDataProcessor
In this example, we allocate an initial heap size of 4GB and a maximum heap size of 8GB, catering to the memory requirements of large-scale data processing.
Additionally, adopting garbage collection strategies like G1GC (Garbage First Garbage Collector) or CMS (Concurrent Mark-Sweep) can mitigate long pauses caused by garbage collection activities, ensuring smoother data processing operations.
Efficient Data Structures for Big Data Storage
Choosing the right data structures is crucial for efficient storage and retrieval of big data. Java offers various data structures, each suited for different use cases. When dealing with massive datasets, optimizing the choice of data structures can have a significant impact on performance.
For instance, utilizing HashMap
instead of ArrayList
for fast retrieval of key-value pairs or employing HashSet
for efficient duplicate elimination within large datasets can streamline data processing operations.
Map<String, Integer> wordCountMap = new HashMap<>();
// Incrementing word count using HashMap
wordCountMap.put(word, wordCountMap.getOrDefault(word, 0) + 1);
By strategically selecting data structures that align with the specific requirements of big data analysis, we can minimize overhead and enhance overall efficiency.
Using External Libraries for Distributed Computing
Java ecosystem boasts a plethora of external libraries and frameworks designed for distributed computing and big data processing, such as Apache Hadoop, Apache Spark, and Apache Flink. These frameworks provide powerful abstractions and tools for parallel processing, fault tolerance, and scalability, making them invaluable assets for tackling big data challenges.
For instance, Apache Spark's resilient distributed dataset (RDD) abstraction enables distributed processing of large-scale data across a cluster of machines, while its rich set of APIs allows for expressive and efficient data manipulation.
JavaRDD<Integer> data = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
int sum = data.reduce((a, b) -> a + b);
System.out.println("Sum of data: " + sum);
By harnessing the capabilities of external libraries tailored for big data analysis, Java developers can leverage distributed computing paradigms to handle massive datasets with ease.
The Closing Argument
Optimizing data crunching for efficient big data analysis in Java involves a multifaceted approach, encompassing parallel processing, memory management, data structures, and harnessing external libraries for distributed computing. By utilizing Java's robust features and ecosystem, developers can conquer the challenges posed by big data, unlocking valuable insights and driving impactful decisions.
In this blog post, we've only scratched the surface of the myriad strategies and techniques available for optimizing big data analysis in Java. As the world of big data continues to evolve, Java remains a stalwart ally for developers and data engineers seeking to conquer the realm of data at scale.
Remember, the journey to mastering big data analysis with Java is an ongoing exploration of optimization and innovation, where each line of code and every architectural decision can shape the trajectory of success in the realm of big data.
So, harness the power of Java, dive into the world of big data, and unleash the potential of data-driven insights with efficiency and finesse. Happy data crunching!