Understanding Hot and Cold Observables in RxJava
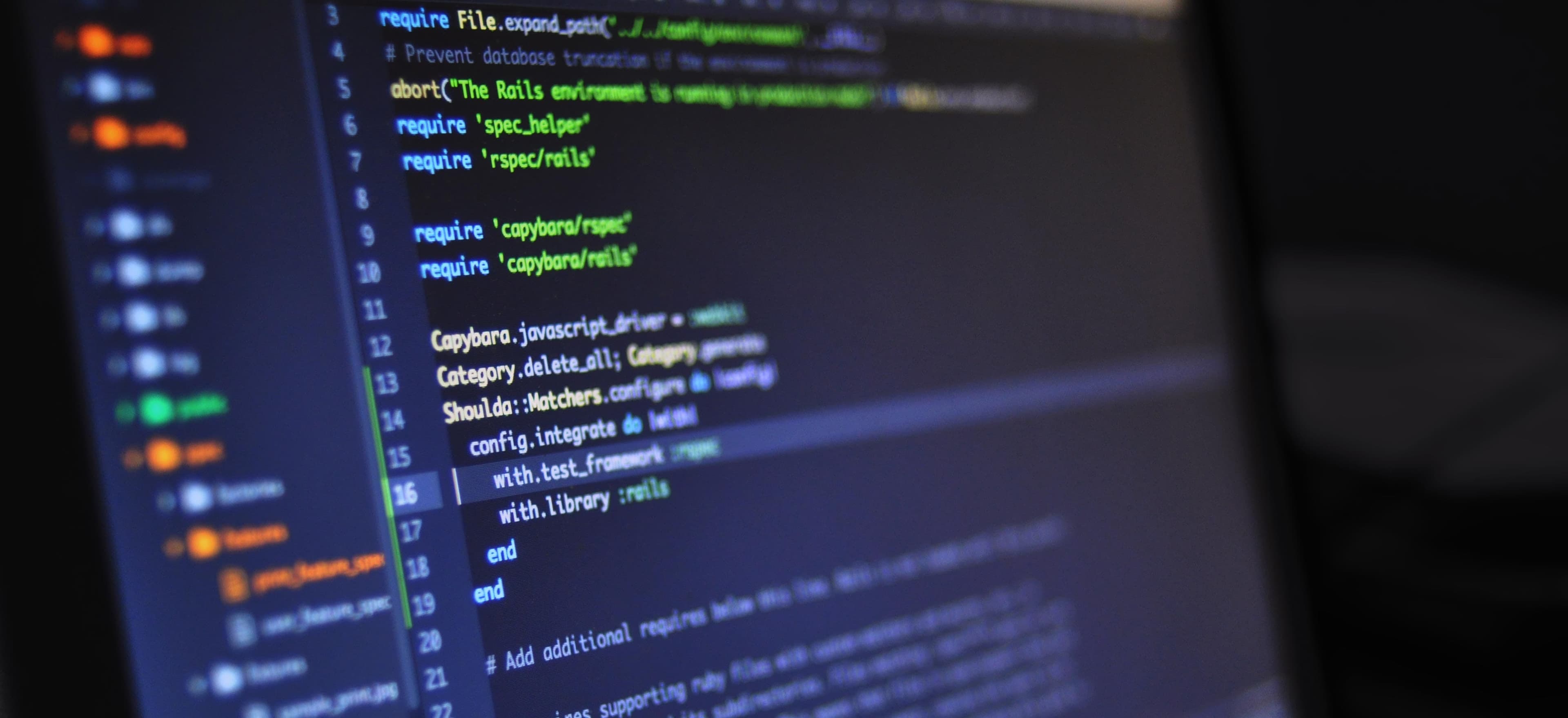
- Published on
Understanding Hot and Cold Observables in RxJava
Reactive programming has gained immense popularity in recent years due to its ability to efficiently handle asynchronous data streams. RxJava is one of the most widely used libraries for reactive programming in Java.
In RxJava, observables play a crucial role in emitting data and propagating changes. Observables can be categorized into two main types: hot observables and cold observables. Understanding the differences between these two types is essential for effectively utilizing RxJava in your projects.
What are Hot Observables?
Hot observables emit data regardless of the presence of subscribers. This means that even if there are no subscribers, the hot observable will continue to emit data. When a subscriber connects to a hot observable, it begins receiving the data stream from the point of subscription, potentially missing previously emitted items.
Use Case for Hot Observables
A classic use case for a hot observable is a stock ticker that continuously emits the latest stock prices. Subscribers can connect to the ticker at any time and start receiving the current stock prices, but they will miss any prices that were emitted before they subscribed.
What are Cold Observables?
On the other hand, cold observables only start emitting data when a subscriber connects to them. Each subscriber receives the entire sequence of data from the beginning of the stream. This ensures that subscribers do not miss any items, as they are always replayed from the start of the stream.
Use Case for Cold Observables
A common use case for a cold observable is a list of user data fetched from a database. Each subscriber should receive the complete list of user data, starting from the first item in the list, regardless of when they subscribe.
Code Examples for Hot and Cold Observables
Let's take a look at some code examples to illustrate the differences between hot and cold observables using RxJava.
Creating a Hot Observable
import io.reactivex.subjects.PublishSubject;
import io.reactivex.subjects.Subject;
// Create a PublishSubject as a hot observable
Subject<String> subject = PublishSubject.create();
// Emits "Hello" to all subscribers
subject.onNext("Hello");
In this example, the PublishSubject
acts as a hot observable and emits the string "Hello" to all subscribers, regardless of when they subscribe.
Creating a Cold Observable
import io.reactivex.Observable;
// Create an Observable as a cold observable
Observable<Integer> numbersObservable = Observable.just(1, 2, 3, 4, 5);
// Subscribe to the cold observable
numbersObservable.subscribe(number -> System.out.println("Received: " + number));
In this case, the Observable
emits the numbers 1 to 5 to any subscriber, replaying the entire sequence from the start for each new subscriber.
Choosing Between Hot and Cold Observables
The choice between hot and cold observables depends on the specific use case and the behavior you want to achieve in your application. Hot observables are suitable for scenarios where subscribers need to access the most current data, such as real-time event streams.
On the other hand, cold observables are preferable when you want to ensure that each subscriber receives the complete sequence of data from the beginning, without missing any items.
Wrapping Up
Understanding the distinction between hot and cold observables is crucial for effectively leveraging RxJava in reactive programming. By choosing the appropriate type of observable for your use case, you can ensure that data streams are handled in a manner that aligns with the requirements of your application.
To delve deeper into RxJava and its observables, consider exploring the official RxJava documentation to enrich your understanding and discover additional nuances in reactive programming.
In conclusion, mastering hot and cold observables provides a strong foundation for building robust and efficient reactive applications using RxJava.