Dealing with Unwanted Git Changes
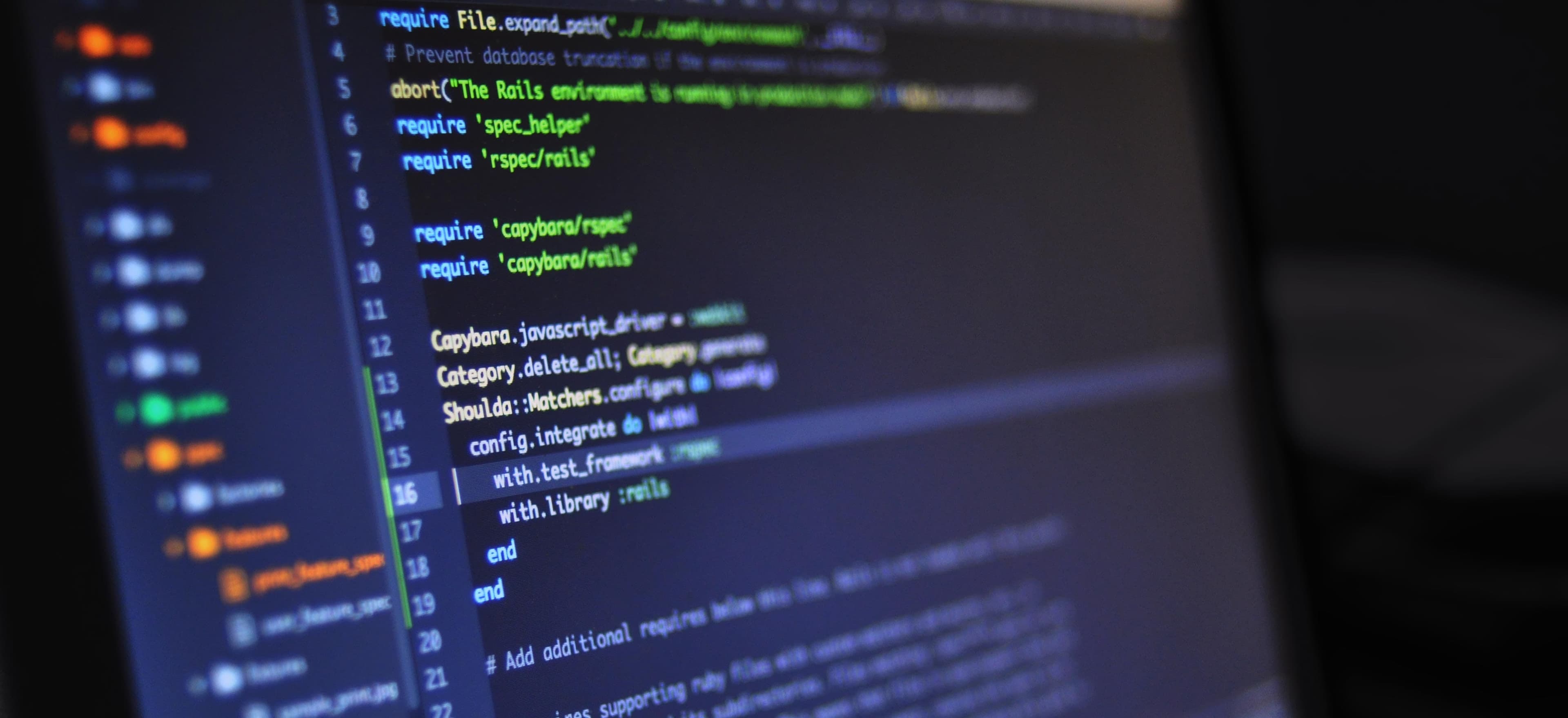
- Published on
Dealing with Unwanted Git Changes
Have you ever found yourself in a situation where you made unwanted changes in your code and committed them to your Git repository? If the answer is yes, don't worry, we've all been there. Fortunately, Git provides several ways to deal with this common issue. In this post, we'll explore how to undo unwanted changes and clean up your Git history.
Identifying Unwanted Changes
Before we dive into the solutions, let's first identify the different types of unwanted changes in a Git repository. These can include:
- Uncommitted changes in your working directory.
- Unstaged changes that have been added to the staging area but not yet committed.
- Committed changes that exist in the commit history.
Now, let's take a look at some strategies to address each of these scenarios.
Undoing Uncommitted Changes
If you've made changes to your code that you haven't yet committed, Git provides a straightforward way to discard these changes and revert to the last committed state.
Discarding Uncommitted Changes in a Single File
If you only need to discard changes in a specific file, you can use the following command:
git checkout -- <file>
This will discard all changes in the specified file and revert it to the state of the last commit.
Discarding All Uncommitted Changes
To discard all changes in your working directory and revert to the last committed state for all files, you can use the following command:
git reset --hard
Keep in mind that this action cannot be undone, so use it with caution.
Undoing Unstaged Changes
If you've added changes to the staging area but haven't committed them yet, you can unstage these changes and revert the files to their state at the time of the last commit.
Unstaging Changes for a Single File
To unstage changes for a specific file while keeping the modifications in your working directory, you can use the following command:
git reset HEAD <file>
This command removes the file from the staging area but leaves the actual changes in the file intact.
Discarding Unstaged Changes
If you want to discard the unstaged changes and revert the files to the state at the time of the last commit, you can combine the git reset
and git checkout
commands:
git reset HEAD
git checkout .
The git reset HEAD
command unsets all changes from the staging area, and git checkout .
discards the changes in the working directory.
Undoing Committed Changes
Undoing committed changes is a bit more involved and generally not recommended if the commits have already been pushed to a remote repository. However, if you need to remove the last commit from your local repository, you can use the following command:
git reset --hard HEAD~1
This command will remove the last commit along with any changes introduced in that commit.
Cleaning Up Git History
In some cases, you may want to clean up your Git history by removing specific commits or rewriting the commit history. This is particularly useful if you've accidentally committed sensitive information or if your commit history has become cluttered.
Removing the Last Commit
To remove the last commit from your local repository without modifying the working directory, you can use the --soft
option with git reset
:
git reset --soft HEAD~1
This will remove the last commit while keeping the changes from that commit in the staging area.
Modifying Commits
If you need to modify the message of the last commit, you can use the --amend
option with the git commit
command:
git commit --amend
This will open your default text editor, allowing you to modify the commit message.
Interactive Rebase
For more advanced history rewriting, Git provides the rebase
command with the interactive flag:
git rebase -i <commit>
This allows you to interactively reword, reorder, squash, or even remove commits from your commit history.
Key Takeaways
In conclusion, Git provides a variety of options to deal with unwanted changes, whether they are uncommitted, staged, or already committed. By understanding these techniques, you can effectively manage your Git repository and maintain a clean commit history. Remember to use these commands with caution, especially when rewriting history or discarding changes, and always make sure to back up your work before performing any destructive operations.
Now that you've learned how to deal with unwanted Git changes, you can confidently navigate through common version control issues and keep your repository in a pristine state. Happy coding!
By following the discussed strategies, you can efficiently manage your Git repository and handle unwanted changes with confidence. If you want to delve deeper into Git commands and best practices, consider checking out the official Git documentation.