Using Hibernate Filters in Grails: Handling Dynamic Filtering
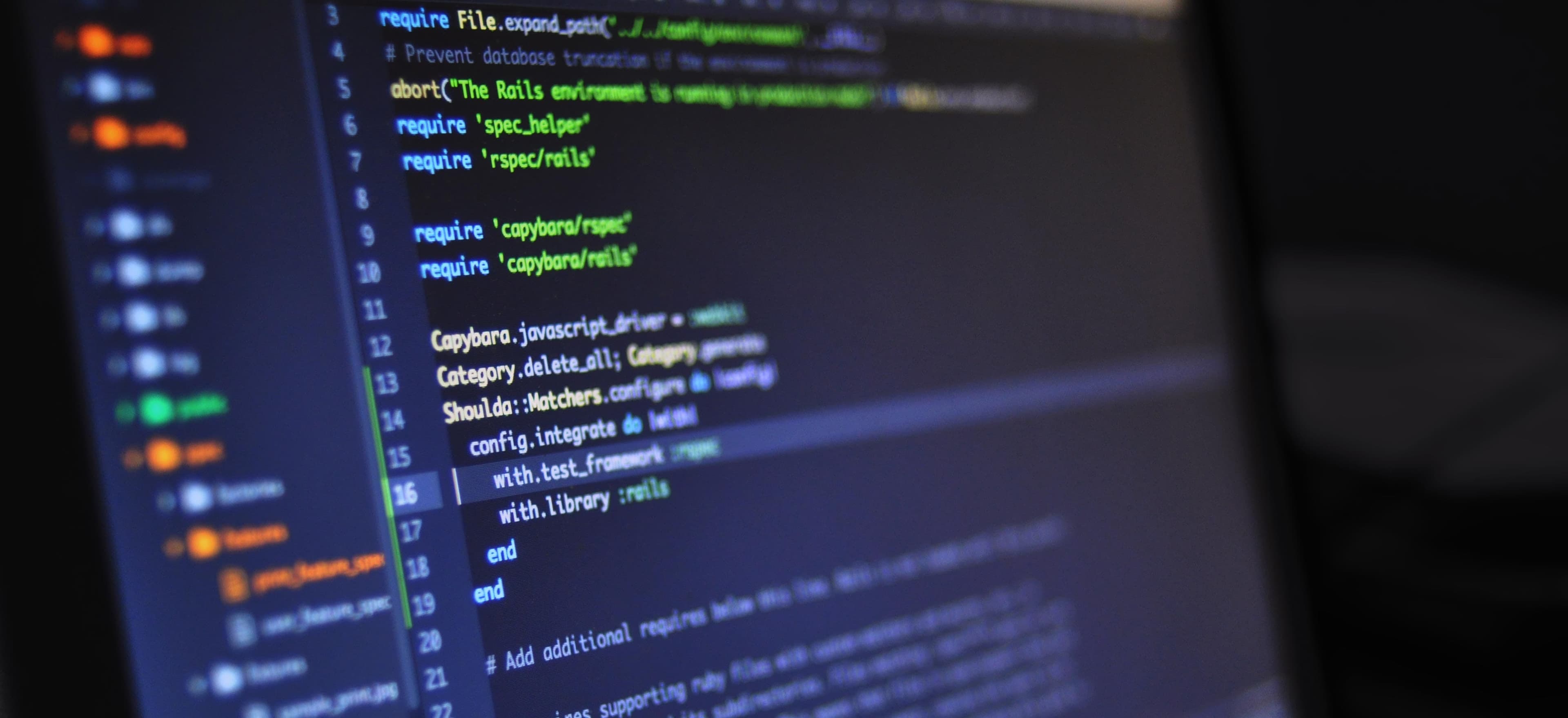
- Published on
Using Hibernate Filters in Grails: Handling Dynamic Filtering
When working with Grails and Hibernate, there are times when you need to apply dynamic filtering to query results. Dynamic filtering allows you to define runtime conditions on your data retrieval operations. In this blog post, we'll explore how to handle dynamic filtering in Grails using Hibernate filters.
What are Hibernate Filters?
Hibernate Filters provide a way to predefine criteria that restrict the rows and collections of an entity based on certain conditions. These conditions can be static or dynamic, allowing for more flexible and customizable data retrieval.
Setting Up Hibernate Filters in Grails
Before we dive into the implementation of dynamic filtering, let's first set up Hibernate Filters in Grails. We'll be working with a simple Book
domain class for demonstration purposes.
class Book {
String title
boolean isDeleted
// Other properties
static mapping = {
filters {
deletedFilter(condition: 'is_deleted = :isDeleted', default: 'false')
}
}
}
In the above code snippet, we've defined a deletedFilter
to be applied to the Book
domain class. The condition
attribute specifies the SQL condition for the filter, while the default
attribute sets the default value for the filter parameter.
Applying Static Filtering
Let's start by applying a static filter to the Book
entity. This filter will always be active whenever a query is performed on the Book
entity.
def books = Book.withNewSession {
Book.withFilter('deletedFilter', [isDeleted: true]) {
Book.list()
}
}
In the above code, we've used the withFilter
method to apply the deletedFilter
with a condition to retrieve only the deleted books.
Implementing Dynamic Filtering
Dynamic filtering allows us to apply filters based on runtime conditions. This can be achieved by using the enableFilter
method from the Hibernate session.
def dynamicFilteredBooks(boolean showDeleted) {
Book.withNewSession { session ->
def filterName = 'deletedFilter'
def filter = session.enableFilter(filterName)
filter.setParameter('isDeleted', showDeleted)
def results = Book.list()
session.disableFilter(filterName)
results
}
}
In the above method, we've defined a dynamicFilteredBooks
method that takes a boolean parameter showDeleted
to determine whether to include deleted books in the results. We enable the deletedFilter
and set the isDeleted
parameter based on the showDeleted
value. After retrieving the results, we disable the filter to ensure it doesn't affect subsequent queries.
Final Thoughts
In this blog post, we've explored how to handle dynamic filtering in Grails using Hibernate filters. By setting up static and dynamic filters, you can customize your data retrieval operations based on specific conditions, leading to more efficient and targeted data retrieval.
Implementing dynamic filtering not only provides flexibility in querying data but also allows for a more organized and maintainable codebase. As you continue to work with Grails and Hibernate, consider leveraging Hibernate filters for a more streamlined data access layer.
For more information on Hibernate filters and Grails, you can refer to the official Grails documentation and the Hibernate Filters guide.
Happy coding!