Maximizing Android App Performance with Kotlin
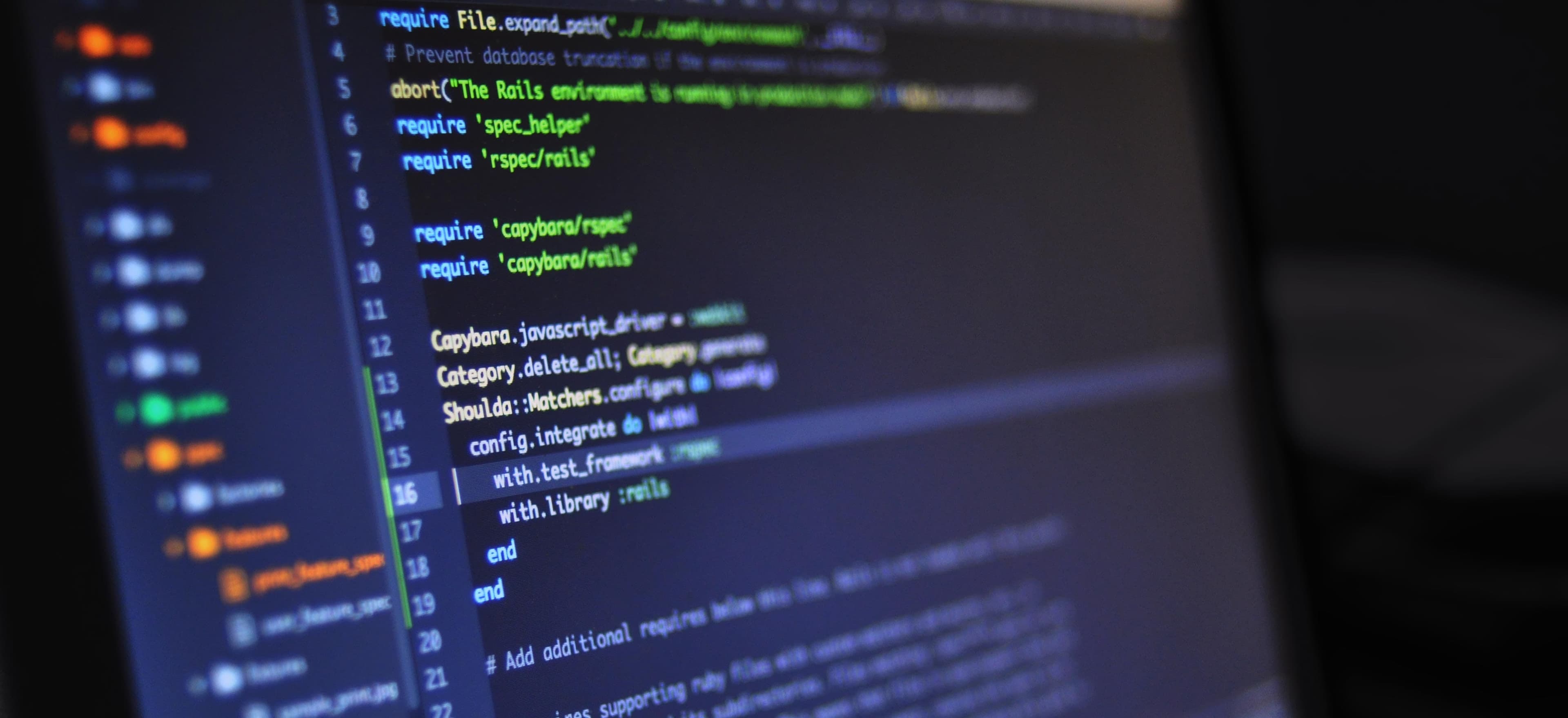
- Published on
Maximizing Android App Performance with Kotlin
As an Android developer, you know how critical it is to ensure that your app performs well. Slow or inefficient apps can lead to user frustration and abandonment. This blog post will provide you with valuable tips and best practices for optimizing the performance of your Android app using Kotlin.
Understanding the Importance of Performance Optimization
Before we delve into the specific techniques for optimizing app performance, let's first understand why it's crucial. Performance optimization is essential for several reasons:
-
User Experience: A performant app provides a seamless user experience, leading to higher user satisfaction and retention.
-
Battery Life: Optimized apps consume less battery, which is a critical factor for mobile users.
-
Retention and Engagement: Users are more likely to engage with and retain an app that is fast and responsive.
Now that we understand the significance of performance optimization, let's explore some effective strategies for achieving it with Kotlin.
Utilizing Kotlin's Concise and Expressive Syntax
Kotlin's concise and expressive syntax enables developers to write clean, readable, and efficient code. By leveraging features such as extension functions, higher-order functions, and null safety, you can streamline your code and minimize potential performance bottlenecks.
Example: Using Extension Functions for Collection Operations
// Before
fun filterUsersByAge(users: List<User>, minAge: Int): List<User> {
val filteredUsers = mutableListOf<User>()
for (user in users) {
if (user.age >= minAge) {
filteredUsers.add(user)
}
}
return filteredUsers
}
// After
fun List<User>.filterByAge(minAge: Int): List<User> {
return filter { it.age >= minAge }
}
By using Kotlin's extension functions, we can simplify the code for filtering users based on age, resulting in cleaner and more efficient code.
In this example, we are not only achieving cleaner code but also improving performance by leveraging Kotlin's powerful features.
Leveraging Kotlin Coroutines for Asynchronous Programming
Asynchronous programming is essential for keeping your app responsive. Kotlin's built-in support for coroutines allows you to perform asynchronous tasks in a concise and efficient manner.
Example: Fetching Data Using Coroutines
// Before
fun fetchData() {
GlobalScope.launch(Dispatchers.IO) {
val result = longRunningApiCall()
withContext(Dispatchers.Main) {
updateUi(result)
}
}
}
// After
suspend fun fetchData() {
val result = withContext(Dispatchers.IO) {
longRunningApiCall()
}
updateUi(result)
}
By utilizing Kotlin coroutines, we can simplify the asynchronous data fetching process, making the code more readable and maintainable while ensuring optimal performance.
Employing Kotlin's Standard Library Functions for Performance
Kotlin's standard library offers a wide range of functions that can significantly contribute to the performance optimization of your app. Functions such as filter
, map
, and reduce
provide concise and efficient ways to manipulate data collections.
Example: Using Standard Library Functions for Data Transformation
val numbers = listOf(1, 2, 3, 4, 5)
val doubledNumbers = numbers.map { it * 2 }
val sum = doubledNumbers.reduce { acc, i -> acc + i }
By leveraging Kotlin's standard library functions, we can perform complex data transformations with minimal code, enhancing both readability and performance.
Utilizing Inline Functions for Performance-Critical Operations
Kotlin's inline
functions can be instrumental in optimizing performance-critical operations. By using inline functions, you can eliminate the overhead of function calls and achieve better performance in scenarios where performance is paramount.
Example: Utilizing Inline Functions
inline fun measureTimeMillis(block: () -> Unit): Long {
val startTime = System.currentTimeMillis()
block()
return System.currentTimeMillis() - startTime
}
fun main() {
val executionTime = measureTimeMillis {
// Code block for performance measurement
// ...
}
println("Execution time: $executionTime ms")
}
In this example, the measureTimeMillis
function uses the inline
keyword to eliminate the overhead of function calls, resulting in improved performance when measuring the execution time of a code block.
Employing ProGuard for Code Minification and Obfuscation
ProGuard is a valuable tool for minimizing the size of your app's code and obfuscating it, making reverse engineering more challenging. By enabling ProGuard in your build configuration, you can significantly reduce the footprint of your app, leading to improved performance and reduced load times.
Example: Enabling ProGuard in Gradle Build Configuration
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
By configuring ProGuard in your Gradle build, you can optimize the size of your app's code, enhance performance, and strengthen its security against reverse engineering.
Closing the Chapter
In this blog post, we've explored several effective strategies for maximizing the performance of your Android app using Kotlin. By embracing Kotlin's concise syntax, leveraging coroutines for asynchronous programming, employing the standard library for efficient operations, utilizing inline functions, and enabling ProGuard for code optimization, you can elevate the performance of your app to new heights.
Optimizing app performance is an ongoing process, and staying updated with the latest advancements in Kotlin and Android development is crucial for maintaining a high-performance app. By integrating these best practices and adopting a performance-oriented mindset, you can ensure that your app delivers a stellar user experience while operating at peak efficiency.
For more in-depth insights into Kotlin and Android app development, check out the official Kotlin documentation and the Android Developer website.
Remember, high-performing apps lead to satisfied users and successful app experiences. So, keep optimizing and happy coding!