Converting Decimal to Hex: The Ultimate Guide
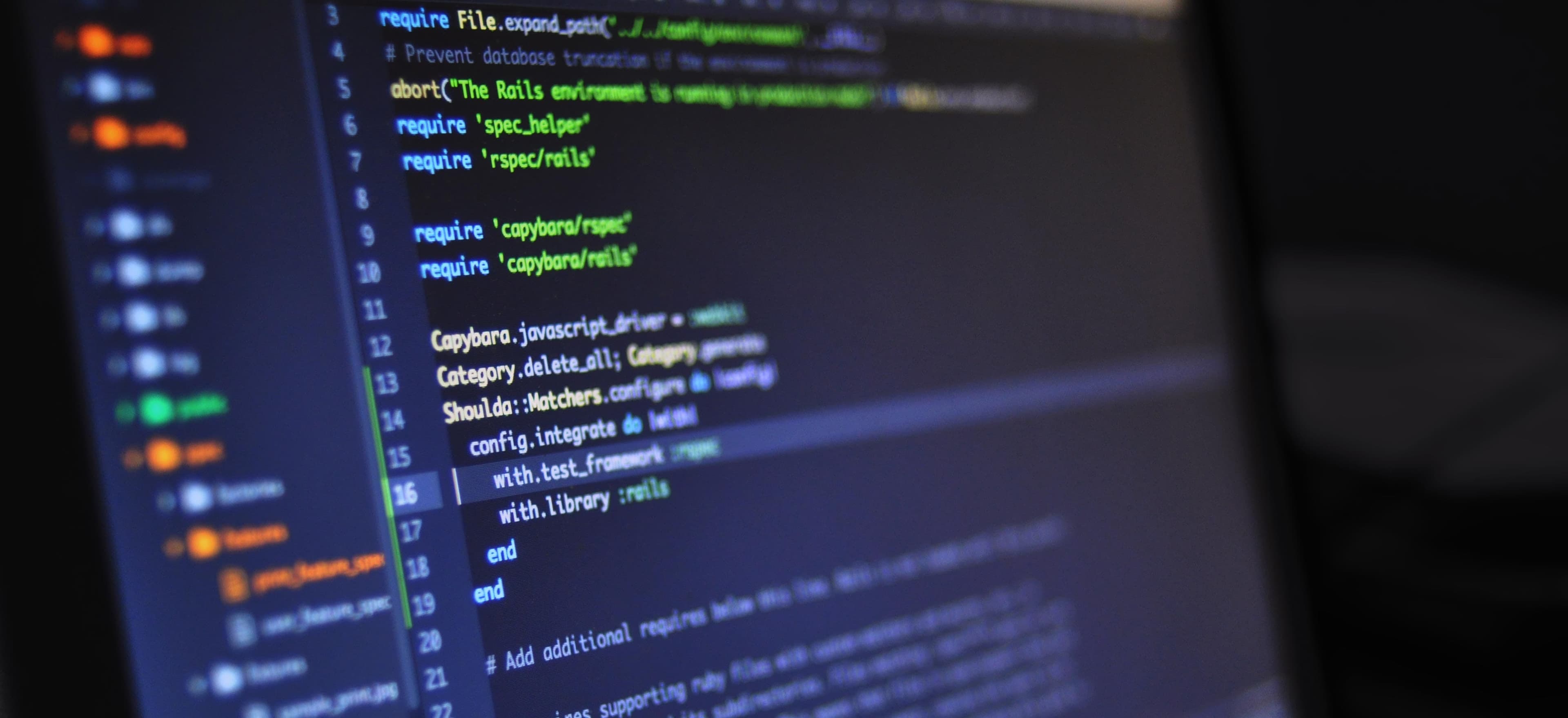
- Published on
Converting Decimal to Hex: The Ultimate Guide
In Java, converting decimal numbers to hexadecimal is a common requirement in various applications. Whether you are working with color codes, network protocols, or cryptography, understanding how to convert decimal numbers to hexadecimal is essential. This guide will walk you through the process, providing explanations, code examples, and best practices to help you master this fundamental task.
Understanding Decimal and Hexadecimal Number Systems
Before diving into the conversion process, let’s refresh our understanding of the decimal and hexadecimal number systems.
- Decimal System: The decimal system, also known as the base-10 system, represents numbers using 10 symbols (0-9). Each position in a decimal number represents a power of 10.
- Hexadecimal System: The hexadecimal system, or base-16 system, uses 16 symbols (0-9 and A-F) to represent numbers. Each position in a hexadecimal number represents a power of 16.
Converting Decimal to Hexadecimal in Java
Java provides built-in methods to convert decimal numbers to hexadecimal representation. The Integer
class in Java offers the toHexString
method for this purpose.
Let’s start with a simple example to illustrate the basic conversion:
int decimalNumber = 305;
String hexadecimalNumber = Integer.toHexString(decimalNumber);
System.out.println("Hexadecimal equivalent of " + decimalNumber + " is " + hexadecimalNumber);
In this example, the toHexString
method converts the decimal number 305
to its hexadecimal representation. Running this code will produce the output: "Hexadecimal equivalent of 305 is 131".
Why Use Integer.toHexString Method?
The Integer.toHexString
method is the most straightforward approach to convert decimal to hexadecimal in Java. It handles the conversion seamlessly without the need for manual calculations or complex algorithms.
Using built-in methods like toHexString
promotes code readability and reduces the likelihood of errors in the conversion process.
Handling Edge Cases
While the Integer.toHexString
method is convenient for most scenarios, it’s essential to consider potential edge cases when converting decimal numbers to hexadecimal in Java.
Negative Numbers
When dealing with negative decimal numbers, the output of toHexString
may not match expectations. To address this, you can leverage the BigInteger
class to obtain the correct hexadecimal representation, as demonstrated in the following snippet:
int negativeDecimalNumber = -127;
String hexadecimalNegativeNumber = new BigInteger(String.valueOf(negativeDecimalNumber)).toString(16);
System.out.println("Hexadecimal equivalent of " + negativeDecimalNumber + " is " + hexadecimalNegativeNumber);
Custom Implementation for Fine-grained Control
In some cases, you may require a custom implementation to handle specific requirements or constraints. Implementing a custom converter allows you to exert precise control over the conversion process.
Here’s a simplified custom implementation to convert a decimal number to hexadecimal by performing manual calculations:
public static String decimalToHexCustom(int decimalNumber) {
if (decimalNumber == 0) {
return "0";
}
StringBuilder hex = new StringBuilder();
while (decimalNumber > 0) {
int remainder = decimalNumber % 16;
if (remainder < 10) {
hex.insert(0, remainder);
} else {
hex.insert(0, (char) ('A' + remainder - 10));
}
decimalNumber /= 16;
}
return hex.toString();
}
The decimalToHexCustom
method provides fine-grained control over the conversion process and can be adapted to suit specific requirements.
Closing Remarks
Converting decimal numbers to hexadecimal in Java is a fundamental operation with diverse applications. Leveraging the toHexString
method of the Integer
class offers a convenient and reliable approach for most scenarios. However, understanding the edge cases and having the ability to create a custom implementation provides additional flexibility and control over the conversion process.
By mastering the conversion from decimal to hexadecimal, you are equipped to work with various systems, data formats, and algorithms that rely on hexadecimal representations. This foundational skill is crucial for tackling advanced programming tasks and building robust, efficient software solutions.
To delve deeper into number systems and their applications in Java, consider exploring the Java Documentation and experimenting with different conversion scenarios to solidify your understanding.