Maximizing Team Efficiency Through Continuous Integration
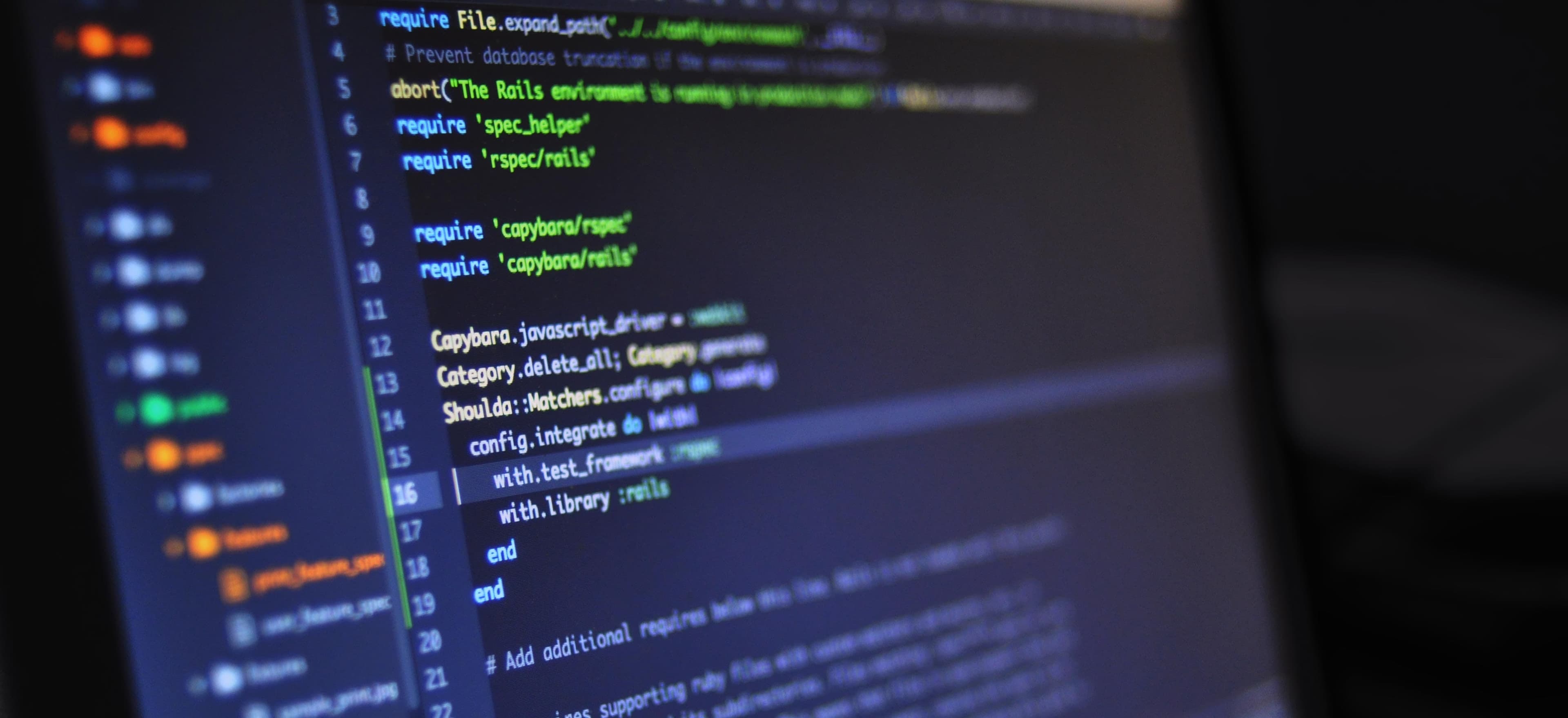
- Published on
Maximizing Team Efficiency Through Continuous Integration
In today's fast-paced software development industry, effective collaboration and efficient workflows are critical for the success of any project. Continuous Integration (CI) is a development practice that emphasizes frequent integration of code changes into a shared repository. This practice, when implemented correctly, can significantly enhance team productivity, streamline the development process, and minimize integration issues. In this blog post, we'll explore the concept of continuous integration, its benefits, best practices, and how to implement it using Java.
What is Continuous Integration?
Continuous Integration is a software development practice where members of a team integrate their work frequently, usually multiple times a day. Each integration is verified by an automated build (including tests) to detect integration errors as quickly as possible. By integrating regularly, you can detect errors quickly, and locate them more easily.
Benefits of Continuous Integration
Early Detection of Issues
By integrating and testing code frequently, CI helps to identify errors and conflicts in the early stages of development. Detecting issues early makes them easier and less costly to resolve.
Faster Feedback Loop
CI provides a faster feedback loop by running automated tests and builds as soon as code is committed. This allows developers to identify and address issues promptly, leading to faster iteration and delivery of features.
Quality Improvement
Continuous Integration encourages the writing of automated tests, which leads to higher code quality and more confidence in the codebase. This, in turn, reduces the chances of shipping defective code to production.
Enhanced Collaboration
CI promotes collaboration among team members by creating a centralized integration point and providing visibility into the changes made by each developer. It helps in resolving merge conflicts and keeping everyone in sync.
Best Practices for Continuous Integration
Maintain a Single Source Repository
It's crucial to maintain a single source repository that serves as the central hub for all code changes. This enables seamless integration and ensures that all team members work with the latest codebase.
Automate the Build and Test Process
Automating the build and test process is fundamental to CI. A robust CI system should automatically build the project and run all tests upon each commit, providing immediate feedback on the code changes.
Keep Builds Fast
Fast builds are essential for maintaining a responsive development feedback loop. Slow builds can delay integration and hinder productivity. Therefore, optimizing build times is crucial for an efficient CI pipeline.
Practice Continuous Deployment
Continuous Integration is often paired with Continuous Deployment (CD), where code changes are automatically deployed to testing or production environments after passing the CI tests. This practice streamlines the release process and ensures that new features are delivered to users quickly and consistently.
Implementing Continuous Integration with Java
Let's walk through a simple example of implementing Continuous Integration using Java and popular CI/CD tools like Jenkins or Travis CI.
Setting Up a Build Script
Here's an example of a simple build script using Apache Maven, a popular build automation tool for Java projects:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myproject</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<!-- Dependencies here -->
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
In this example, we define a basic Maven project configuration with the necessary dependencies and build settings.
Incorporating Automated Testing
Using a testing framework like JUnit, we can write automated tests for our Java code. Here's a simple example of a JUnit test for a Java class:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyMathTest {
@Test
public void testAddition() {
MyMath math = new MyMath();
int result = math.add(3, 4);
assertEquals(7, result);
}
}
In this test, we create a simple unit test to verify the functionality of the add
method in the MyMath
class.
Configuring CI/CD Pipeline
Once the codebase and tests are in place, we can set up a CI/CD pipeline using tools like Jenkins or Travis CI. These tools can be configured to automatically build the project, run tests, and trigger deployment processes based on the outcome of the CI tests.
The Closing Argument
Continuous Integration plays a vital role in modern software development by promoting collaboration, reducing integration issues, and accelerating the feedback loop. By adhering to best practices and leveraging the right tools, teams can maximize their efficiency, deliver higher-quality software, and adapt to rapid changes in the industry.
In this blog post, we've merely scratched the surface of Continuous Integration and its implementation with Java. Incorporating CI into your development process requires careful planning, consistent execution, and a willingness to embrace automation and rapid feedback. As you delve deeper into CI, exploring advanced topics such as automated deployment, containerization, and infrastructure as code will further enhance your team's efficiency and agility.
Continuous Integration isn't just a technological practice; it's a cultural shift that fosters a collaborative and quality-focused mindset within development teams. Embracing CI empowers teams to innovate, respond to user feedback, and deliver value to customers at a rapid pace, ultimately driving business success in today's competitive digital landscape.