Managing Concurrent Access with Java Synchronized Keyword
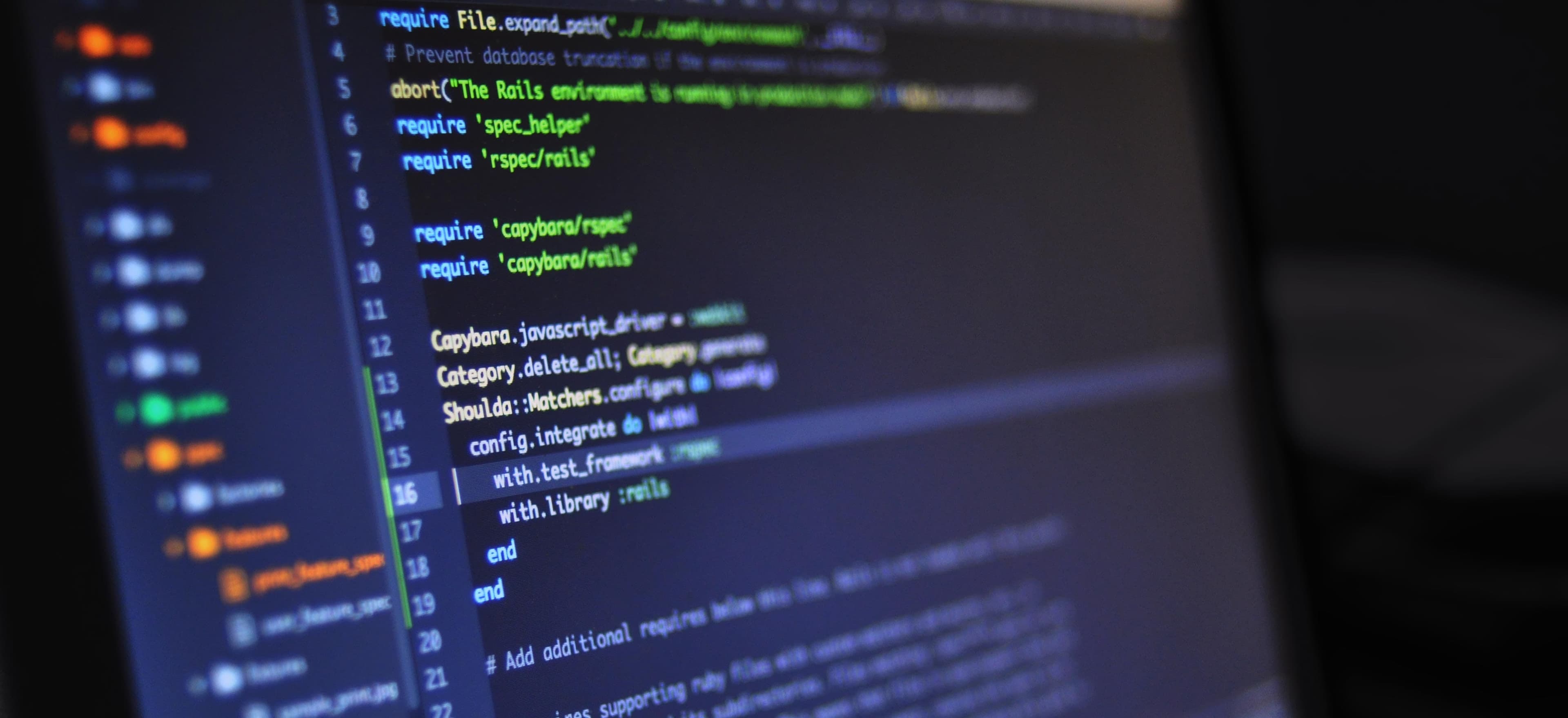
- Published on
Managing Concurrent Access with Java Synchronized Keyword
When developing multi-threaded applications in Java, managing concurrent access to shared resources is crucial to prevent data corruption and ensure the integrity of the application. One approach to achieve this is through the use of the synchronized
keyword.
Understanding Concurrent Access
In a multi-threaded environment, multiple threads may attempt to access and modify shared resources simultaneously. Without proper synchronization, this can lead to race conditions, where the outcome of the operations depends on the precise timing of the threads, often resulting in unexpected behavior and potential data corruption.
The Synchronized Keyword
The synchronized
keyword in Java ensures that only one thread can execute a synchronized method or block of code on a given object at a time. This prevents concurrent access and ensures that only one thread can modify the shared resource at any given time, effectively eliminating race conditions.
Synchronized Methods
In Java, methods can be declared as synchronized to achieve thread-safe access to the shared resources. When a method is marked as synchronized, only one thread can execute that method on an object at a time, effectively serializing the access to the method.
Consider the following example where a Counter
class has a synchronized method to increment a shared count:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
In this example, the increment
method is declared as synchronized, ensuring that only one thread can execute it at a time, preventing concurrent modification of the count
variable.
Synchronized Blocks
In addition to synchronized methods, Java also supports synchronized blocks, allowing more granular control over the synchronized code. Synchronized blocks are particularly useful when only a specific portion of the method needs to be synchronized.
The syntax for a synchronized block is as follows:
synchronized (object) {
// Synchronized code block
}
Here, object
is the reference to the object used for synchronization. Only one thread can execute the synchronized block associated with the given object at a time.
Let's consider an example where a synchronized block is used to ensure thread-safe access within a method:
public class SharedResource {
private Object lock = new Object();
private int sharedData = 0;
public void performOperation() {
synchronized (lock) {
// Thread-safe operations
sharedData++;
}
}
}
In this example, the performOperation
method utilizes a synchronized block to ensure that the sharedData
variable is modified by only one thread at a time, using the lock
object as the monitor for synchronization.
Understanding the Why
It's crucial to understand the rationale behind using the synchronized
keyword and the implications of managing concurrent access. By synchronizing methods or blocks, developers ensure that critical sections of code are executed atomically, preventing inconsistent states and ensuring thread safety.
The synchronized
keyword provides a simple and effective means of controlling concurrent access, allowing for the development of robust, multi-threaded applications without the complexities of lower-level thread management.
To Wrap Things Up
In Java, the synchronized
keyword plays a pivotal role in managing concurrent access to shared resources in multi-threaded applications. By synchronizing methods and code blocks, developers can mitigate race conditions and ensure the integrity of shared data.
Understanding when and how to use the synchronized
keyword is essential for writing efficient, thread-safe code. However, it's important to note that while synchronized
keyword provides a convenient way to manage concurrent access, it's not always the most performant approach, and developers should explore other synchronization mechanisms such as Lock
interfaces and atomic variables for more granular control and improved performance in certain scenarios.
By mastering the use of the synchronized
keyword, developers can pave the way for robust, scalable, and reliable multi-threaded applications.